mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-01 18:32:32 +00:00
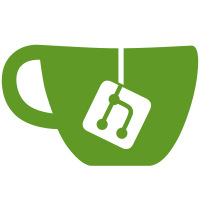
* [NOD-1551] Make UTXO-Diff implemented fully in utils/utxo * [NOD-1551] Fixes everywhere except database * [NOD-1551] Fix database * [NOD-1551] Add comments * [NOD-1551] Partial commit * [NOD-1551] Comlete making UTXOEntry immutable + don't clone it in UTXOCollectionClone * [NOD-1551] Rename ToUnmutable -> ToImmutable * [NOD-1551] Track immutable references generated from mutable UTXODiff, and invalidate them if the mutable one changed * [NOD-1551] Clone scriptPubKey in NewUTXOEntry * [NOD-1551] Remove redundant code * [NOD-1551] Remove redundant call for .CloneMutable and then .ToImmutable * [NOD-1551] Make utxoEntry pointert-receiver + clone ScriptPubKey in getter
87 lines
2.0 KiB
Go
87 lines
2.0 KiB
Go
package utxo
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
type immutableUTXODiff struct {
|
|
mutableUTXODiff *mutableUTXODiff
|
|
|
|
isInvalidated bool
|
|
}
|
|
|
|
func (iud *immutableUTXODiff) ToAdd() model.UTXOCollection {
|
|
if iud.isInvalidated {
|
|
panic("Attempt to read from an invalidated UTXODiff")
|
|
}
|
|
|
|
return iud.mutableUTXODiff.ToAdd()
|
|
}
|
|
|
|
func (iud *immutableUTXODiff) ToRemove() model.UTXOCollection {
|
|
if iud.isInvalidated {
|
|
panic("Attempt to read from an invalidated UTXODiff")
|
|
}
|
|
|
|
return iud.mutableUTXODiff.ToRemove()
|
|
}
|
|
|
|
func (iud *immutableUTXODiff) WithDiff(other model.UTXODiff) (model.UTXODiff, error) {
|
|
if iud.isInvalidated {
|
|
panic("Attempt to read from an invalidated UTXODiff")
|
|
}
|
|
|
|
return iud.mutableUTXODiff.WithDiff(other)
|
|
}
|
|
|
|
func (iud *immutableUTXODiff) DiffFrom(other model.UTXODiff) (model.UTXODiff, error) {
|
|
if iud.isInvalidated {
|
|
panic("Attempt to read from an invalidated UTXODiff")
|
|
}
|
|
|
|
return iud.mutableUTXODiff.DiffFrom(other)
|
|
}
|
|
|
|
// NewUTXODiff creates an empty UTXODiff
|
|
func NewUTXODiff() model.UTXODiff {
|
|
return newUTXODiff()
|
|
}
|
|
|
|
func newUTXODiff() *immutableUTXODiff {
|
|
return &immutableUTXODiff{
|
|
mutableUTXODiff: newMutableUTXODiff(),
|
|
isInvalidated: false,
|
|
}
|
|
}
|
|
|
|
// NewUTXODiffFromCollections returns a new UTXODiff with the given toAdd and toRemove collections
|
|
func NewUTXODiffFromCollections(toAdd, toRemove model.UTXOCollection) (model.UTXODiff, error) {
|
|
add, ok := toAdd.(utxoCollection)
|
|
if !ok {
|
|
return nil, errors.New("toAdd is not of type utxoCollection")
|
|
}
|
|
remove, ok := toRemove.(utxoCollection)
|
|
if !ok {
|
|
return nil, errors.New("toRemove is not of type utxoCollection")
|
|
}
|
|
return &immutableUTXODiff{
|
|
mutableUTXODiff: &mutableUTXODiff{
|
|
toAdd: add,
|
|
toRemove: remove,
|
|
},
|
|
}, nil
|
|
}
|
|
|
|
func (iud *immutableUTXODiff) CloneMutable() model.MutableUTXODiff {
|
|
return iud.cloneMutable()
|
|
}
|
|
|
|
func (iud *immutableUTXODiff) cloneMutable() *mutableUTXODiff {
|
|
if iud == nil {
|
|
return nil
|
|
}
|
|
|
|
return iud.mutableUTXODiff.clone()
|
|
}
|