mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-04 03:42:30 +00:00
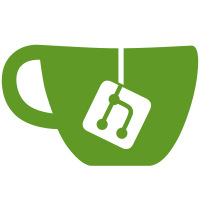
* '' * '' * '' * Changes genesis block version to 0. * a * a * All tests are done. * All tests passed for changed block version from int32 to uint16 * Adds validation of rejecting blocks with unknown versions. * Changes txn version from int32 to uint16. * . * Adds comments to exported functions. * Change functions name from ConvertFromRpcScriptPubKeyToRPCScriptPubKey to ConvertFromAppMsgRPCScriptPubKeyToRPCScriptPubKey and from ConvertFromRPCScriptPubKeyToRpcScriptPubKey to ConvertFromRPCScriptPubKeyToAppMsgRPCScriptPubKey * change comment to "ScriptPublicKey represents a Kaspad ScriptPublicKey" * delete part (tx.Version < 0) that cannot be exist on the if statement. * Revert protobuf version. * Fix a comment. * Fix a comment. * Rename a variable. * Rename a variable. * Remove a const. * Rename a type. * Rename a field. * Rename a field. * Remove commented-out code. * Remove dangerous nil case in DomainTransactionOutput.Clone(). * Remove a constant. * Fix a string. * Fix wrong totalScriptPubKeySize in transactionMassStandalonePart. * Remove a constant. * Remove an unused error. * Fix a serialization error. * Specify version types to be uint16 explicitly. * Use constants.ScriptPublicKeyVersion. * Fix a bad test. * Remove some whitespace. * Add a case to utxoEntry.Equal(). * Rename scriptPubKey to scriptPublicKey. * Remove a TODO. * Rename constants. * Rename a variable. * Add version to parseShortForm. Co-authored-by: tal <tal@daglabs.com> Co-authored-by: stasatdaglabs <stas@daglabs.com>
81 lines
2.0 KiB
Go
81 lines
2.0 KiB
Go
package utxo
|
|
|
|
import (
|
|
"bytes"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
type utxoEntry struct {
|
|
amount uint64
|
|
scriptPublicKey *externalapi.ScriptPublicKey
|
|
blockBlueScore uint64
|
|
isCoinbase bool
|
|
}
|
|
|
|
// NewUTXOEntry creates a new utxoEntry representing the given txOut
|
|
func NewUTXOEntry(amount uint64, scriptPubKey *externalapi.ScriptPublicKey, isCoinbase bool, blockBlueScore uint64) externalapi.UTXOEntry {
|
|
scriptPubKeyClone := externalapi.ScriptPublicKey{Script: make([]byte, len(scriptPubKey.Script)), Version: scriptPubKey.Version}
|
|
copy(scriptPubKeyClone.Script, scriptPubKey.Script)
|
|
return &utxoEntry{
|
|
amount: amount,
|
|
scriptPublicKey: &scriptPubKeyClone,
|
|
blockBlueScore: blockBlueScore,
|
|
isCoinbase: isCoinbase,
|
|
}
|
|
}
|
|
|
|
func (u *utxoEntry) Amount() uint64 {
|
|
return u.amount
|
|
}
|
|
|
|
func (u *utxoEntry) ScriptPublicKey() *externalapi.ScriptPublicKey {
|
|
clone := externalapi.ScriptPublicKey{Script: make([]byte, len(u.scriptPublicKey.Script)), Version: u.scriptPublicKey.Version}
|
|
copy(clone.Script, u.scriptPublicKey.Script)
|
|
return &clone
|
|
}
|
|
|
|
func (u *utxoEntry) BlockBlueScore() uint64 {
|
|
return u.blockBlueScore
|
|
}
|
|
|
|
func (u *utxoEntry) IsCoinbase() bool {
|
|
return u.isCoinbase
|
|
}
|
|
|
|
// Equal returns whether entry equals to other
|
|
func (u *utxoEntry) Equal(other externalapi.UTXOEntry) bool {
|
|
if u == nil || other == nil {
|
|
return u == other
|
|
}
|
|
|
|
// If only the underlying value of other is nil it'll
|
|
// make `other == nil` return false, so we check it
|
|
// explicitly.
|
|
downcastedOther := other.(*utxoEntry)
|
|
if u == nil || downcastedOther == nil {
|
|
return u == downcastedOther
|
|
}
|
|
|
|
if u.Amount() != other.Amount() {
|
|
return false
|
|
}
|
|
|
|
if !bytes.Equal(u.ScriptPublicKey().Script, other.ScriptPublicKey().Script) {
|
|
return false
|
|
}
|
|
|
|
if u.ScriptPublicKey().Version != other.ScriptPublicKey().Version {
|
|
return false
|
|
}
|
|
|
|
if u.BlockBlueScore() != other.BlockBlueScore() {
|
|
return false
|
|
}
|
|
|
|
if u.IsCoinbase() != other.IsCoinbase() {
|
|
return false
|
|
}
|
|
|
|
return true
|
|
}
|