mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 15:26:42 +00:00
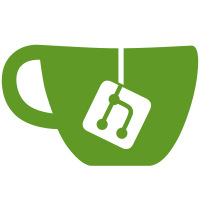
* [NOD-1414] Add interfaces for Factory and State. * [NOD-1414] Create interfaces for algorithms and data stores. * [NOD-1414] Create empty implementations for algorithms and data stores. * [NOD-1414] Add new functions for all the implementations. * [NOD-1414] Begin filling in the interfaces. * [NOD-1414] Fill in the interfaces for the data structures. * [NOD-1414] Fill in the interfaces for the algorithms. * [NOD-1414] Fix a bug in package names. * [NOD-1414] Connect up the various interfaces. * [NOD-1414] Add stubs to all the implementations. * [NOD-1414] Create MiningManager and its Factory. * [NOD-1414] Add interfaces for mempool and blockTemplateBuilder. * [NOD-1414] Add implementation structs for miningManager. * [NOD-1414] Add stub implementations for mempool and blockTemplateBuilder. * [NOD-1414] Rename state to kaspadState. * [NOD-1414] Restructure where interfaces sit. * [NOD-1414] Restructure where interfaces sit in the algorithms package as well. * [NOD-1414] Move remaining models out of models.go. * [NOD-1414] Modified some interfaces. * [NOD-1414] Make go vet happy. * [NOD-1414] Move SerializedUTXOSet into PruningManager. * [NOD-1414] Modify FindNextPruningPoint to return found and nextPruningPointUTXOSet. * [NOD-1414] Add IsDAGAncestorOf. * [NOD-1414] Add PruningPoint(). * [NOD-1414] Add Entry() to ReadOnlyUTXOSet. * [NOD-1414] Add MergeSet() to BlockGHOSTDAGData. * [NOD-1414] Write comments for all the exported types and functions in miningmanager. * [NOD-1414] Add comments to the upper levels of KaspadState. * [NOD-1414] Replace AddNode with ReachabilityChangeset. * [NOD-1414] Add payAddress and extraData to GetBlockTemplate. * [NOD-1414] Add scriptPublicKey and extraData to BuildBlock. * [NOD-1414] Rename algorithms to processes. * [NOD-1414] Rename kaspadState to consensus. * [NOD-1414] Add ValidateAgainstPastUTXO and ValidateFinality. * [NOD-1414] Add BlockGHOSTDAGData to ReachabilityChangeset. * [NOD-1414] Fix the comment over Mempool. * [NOD-1414] Fix the comment over ValidateTransaction. * [NOD-1414] Fill up the data structures. * [NOD-1414] Add comments to remaining uncommented items in miningmanager. * [NOD-1414] Add comments to structs and constructors. * [NOD-1414] Rename Set to Insert. * [NOD-1414] Add comments to everything inside datastructures. * [NOD-1414] Add comments to everything inside models. * [NOD-1414] Add comments to the interfaces in processes. * [NOD-1414] Add comments to everything in processes. * [NOD-1414] Make go vet happy. * [NOD-1414] Rename scriptPublicKey to coinbaseScriptPublicKey. * [NOD-1414] Add handlers to the consensus. * [NOD-1414] Add highHash to blockAtDepth. * [NOD-1414] Add resolveFinalityConflict. * [NOD-1414] Reorg BlockValidator. * [NOD-1414] In ResolveFinalityConflicts, rename blockHash to newFinalityBlockHash. * [NOD-1414] Fix a comment. * [NOD-1414] Make reachability structs public. * [NOD-1414] Make UTXO structs public.
87 lines
3.1 KiB
Go
87 lines
3.1 KiB
Go
package blockprocessor
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/domain/consensus/datastructures"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/processes"
|
|
"github.com/kaspanet/kaspad/domain/dagconfig"
|
|
"github.com/kaspanet/kaspad/infrastructure/db/dbaccess"
|
|
)
|
|
|
|
// BlockProcessor is responsible for processing incoming blocks
|
|
// and creating blocks from the current state
|
|
type BlockProcessor struct {
|
|
dagParams *dagconfig.Params
|
|
databaseContext *dbaccess.DatabaseContext
|
|
|
|
consensusStateManager processes.ConsensusStateManager
|
|
pruningManager processes.PruningManager
|
|
blockValidator processes.BlockValidator
|
|
dagTopologyManager processes.DAGTopologyManager
|
|
reachabilityTree processes.ReachabilityTree
|
|
acceptanceDataStore datastructures.AcceptanceDataStore
|
|
blockIndex datastructures.BlockIndex
|
|
blockMessageStore datastructures.BlockMessageStore
|
|
blockStatusStore datastructures.BlockStatusStore
|
|
}
|
|
|
|
// New instantiates a new BlockProcessor
|
|
func New(
|
|
dagParams *dagconfig.Params,
|
|
databaseContext *dbaccess.DatabaseContext,
|
|
consensusStateManager processes.ConsensusStateManager,
|
|
pruningManager processes.PruningManager,
|
|
blockValidator processes.BlockValidator,
|
|
dagTopologyManager processes.DAGTopologyManager,
|
|
reachabilityTree processes.ReachabilityTree,
|
|
acceptanceDataStore datastructures.AcceptanceDataStore,
|
|
blockIndex datastructures.BlockIndex,
|
|
blockMessageStore datastructures.BlockMessageStore,
|
|
blockStatusStore datastructures.BlockStatusStore) *BlockProcessor {
|
|
|
|
return &BlockProcessor{
|
|
dagParams: dagParams,
|
|
databaseContext: databaseContext,
|
|
pruningManager: pruningManager,
|
|
blockValidator: blockValidator,
|
|
dagTopologyManager: dagTopologyManager,
|
|
reachabilityTree: reachabilityTree,
|
|
|
|
consensusStateManager: consensusStateManager,
|
|
acceptanceDataStore: acceptanceDataStore,
|
|
blockIndex: blockIndex,
|
|
blockMessageStore: blockMessageStore,
|
|
blockStatusStore: blockStatusStore,
|
|
}
|
|
}
|
|
|
|
// BuildBlock builds a block over the current state, with the transactions
|
|
// selected by the given transactionSelector
|
|
func (bp *BlockProcessor) BuildBlock(coinbaseScriptPublicKey []byte, coinbaseExtraData []byte,
|
|
transactionSelector model.TransactionSelector) *appmessage.MsgBlock {
|
|
|
|
return nil
|
|
}
|
|
|
|
// ValidateAndInsertBlock validates the given block and, if valid, applies it
|
|
// to the current state
|
|
func (bp *BlockProcessor) ValidateAndInsertBlock(block *appmessage.MsgBlock) error {
|
|
return nil
|
|
}
|
|
|
|
// SetOnBlockAddedToDAGHandler set the onBlockAddedToDAGHandler for the block processor
|
|
func (bp *BlockProcessor) SetOnBlockAddedToDAGHandler(onBlockAddedToDAGHandler model.OnBlockAddedToDAGHandler) {
|
|
|
|
}
|
|
|
|
// SetOnChainChangedHandler set the onBlockAddedToDAGHandler for the block processor
|
|
func (bp *BlockProcessor) SetOnChainChangedHandler(onChainChangedHandler model.OnChainChangedHandler) {
|
|
|
|
}
|
|
|
|
// SetOnFinalityConflictHandler set the onBlockAddedToDAGHandler for the block processor
|
|
func (bp *BlockProcessor) SetOnFinalityConflictHandler(onFinalityConflictHandler model.OnFinalityConflictHandler) {
|
|
|
|
}
|