mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-22 14:56:44 +00:00
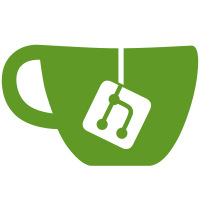
* [NOD-1551] Make UTXO-Diff implemented fully in utils/utxo * [NOD-1551] Fixes everywhere except database * [NOD-1551] Fix database * [NOD-1551] Add comments * [NOD-1551] Partial commit * [NOD-1551] Comlete making UTXOEntry immutable + don't clone it in UTXOCollectionClone * [NOD-1551] Rename ToUnmutable -> ToImmutable * [NOD-1551] Track immutable references generated from mutable UTXODiff, and invalidate them if the mutable one changed * [NOD-1551] Clone scriptPubKey in NewUTXOEntry * [NOD-1551] Remove redundant code * [NOD-1551] Remove redundant call for .CloneMutable and then .ToImmutable * [NOD-1551] Make utxoEntry pointert-receiver + clone ScriptPubKey in getter
40 lines
942 B
Go
40 lines
942 B
Go
package serialization
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/utxo"
|
|
)
|
|
|
|
// UTXODiffToDBUTXODiff converts UTXODiff to DbUtxoDiff
|
|
func UTXODiffToDBUTXODiff(diff model.UTXODiff) (*DbUtxoDiff, error) {
|
|
toAdd, err := utxoCollectionToDBUTXOCollection(diff.ToAdd())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
toRemove, err := utxoCollectionToDBUTXOCollection(diff.ToRemove())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return &DbUtxoDiff{
|
|
ToAdd: toAdd,
|
|
ToRemove: toRemove,
|
|
}, nil
|
|
}
|
|
|
|
// DBUTXODiffToUTXODiff converts DbUtxoDiff to UTXODiff
|
|
func DBUTXODiffToUTXODiff(diff *DbUtxoDiff) (model.UTXODiff, error) {
|
|
toAdd, err := dbUTXOCollectionToUTXOCollection(diff.ToAdd)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
toRemove, err := dbUTXOCollectionToUTXOCollection(diff.ToRemove)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return utxo.NewUTXODiffFromCollections(toAdd, toRemove)
|
|
}
|