mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-22 14:56:44 +00:00
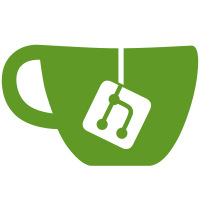
* [NOD-1566] Add a dependency to golang-lru. * [NOD-1566] Add caching to blockstore.go. * [NOD-1566] Add LRUCache to all store objects and initialize them. * [NOD-1566] Add caching to acceptanceDataStore. * [NOD-1566] Add caching to blockHeaderStore. * [NOD-1566] Implement a simpler LRU cache. * [NOD-1566] Use the simpler cache implementation everywhere. * [NOD-1566] Remove dependency in golang-lru. * [NOD-1566] Fix object reuse issues in store Get functions. * [NOD-1566] Add caching to blockRelationStore. * [NOD-1566] Add caching to blockStatusStore. * [NOD-1566] Add caching to ghostdagDataStore. * [NOD-1566] Add caching to multisetStore. * [NOD-1566] Add caching to reachabilityDataStore. * [NOD-1566] Add caching to utxoDiffStore. * [NOD-1566] Add caching to reachabilityReindexRoot. * [NOD-1566] Add caching to pruningStore. * [NOD-1566] Add caching to headerTipsStore. * [NOD-1566] Add caching to consensusStateStore. * [NOD-1566] Add comments explaining why we don't discard staging at the normal location in consensusStateStore. * [NOD-1566] Make go vet happy. * [NOD-1566] Fix merge errors. * [NOD-1566] Add a missing break statement. * [NOD-1566] Run go mod tidy. * [NOD-1566] Remove serializedUTXOSetCache.
75 lines
1.8 KiB
Go
75 lines
1.8 KiB
Go
package consensusstatestore
|
|
|
|
import (
|
|
"github.com/golang/protobuf/proto"
|
|
"github.com/kaspanet/kaspad/domain/consensus/database/serialization"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/dbkeys"
|
|
)
|
|
|
|
var tipsKey = dbkeys.MakeBucket().Key([]byte("tips"))
|
|
|
|
func (css *consensusStateStore) Tips(dbContext model.DBReader) ([]*externalapi.DomainHash, error) {
|
|
if css.tipsStaging != nil {
|
|
return externalapi.CloneHashes(css.tipsStaging), nil
|
|
}
|
|
|
|
if css.tipsCache != nil {
|
|
return externalapi.CloneHashes(css.tipsCache), nil
|
|
}
|
|
|
|
tipsBytes, err := dbContext.Get(tipsKey)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
tips, err := css.deserializeTips(tipsBytes)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
css.tipsCache = tips
|
|
return externalapi.CloneHashes(tips), nil
|
|
}
|
|
|
|
func (css *consensusStateStore) StageTips(tipHashes []*externalapi.DomainHash) {
|
|
css.tipsStaging = externalapi.CloneHashes(tipHashes)
|
|
}
|
|
|
|
func (css *consensusStateStore) commitTips(dbTx model.DBTransaction) error {
|
|
if css.tipsStaging == nil {
|
|
return nil
|
|
}
|
|
|
|
tipsBytes, err := css.serializeTips(css.tipsStaging)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
err = dbTx.Put(tipsKey, tipsBytes)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
css.tipsCache = css.tipsStaging
|
|
|
|
// Note: we don't discard the staging here since that's
|
|
// being done at the end of Commit()
|
|
return nil
|
|
}
|
|
|
|
func (css *consensusStateStore) serializeTips(tips []*externalapi.DomainHash) ([]byte, error) {
|
|
dbTips := serialization.TipsToDBTips(tips)
|
|
return proto.Marshal(dbTips)
|
|
}
|
|
|
|
func (css *consensusStateStore) deserializeTips(tipsBytes []byte) ([]*externalapi.DomainHash,
|
|
error) {
|
|
|
|
dbTips := &serialization.DbTips{}
|
|
err := proto.Unmarshal(tipsBytes, dbTips)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return serialization.DBTipsToTips(dbTips)
|
|
}
|