mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 06:12:32 +00:00
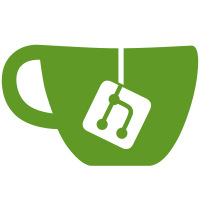
* [NOD-1313] Refactor AddressManager (#918) * [NOD-1313] Refactor AddressManager. * [NOD-1313]Remove old tests.Minor improvements,fixes. * [NOD-1313] After merge fixes. Fix import cycle. * [NOD-1313] Integration tests fixes. * [NOD-1313] Allocate new slice for the returned key. * [NOD-1313] AddressManager improvements and fixes. * Move local and banned addresses to separate lists. * Move AddressManager config to the separate file. * Add LocalAddressManager. * Remove redundant KnownAddress structure. * Restore local addresses functionality. * Call initListeners from the LocalAddressManager. * AddressManager minor improvements and fixes. * [NOD-1313] Minor fixes. * [NOD-1313] Implement HandleGetPeerAddresses. Refactoring. * [NOD-1313] After-merge fixes. * [NOD-1313] Minor improvements. * AddressManager: added BannedAddresses() method. * AddressManager: HandleGetPeerAddresses() add banned addresses separately. * AddressManager: remove addressEntry redundant struct. * ConnectionManager: checkOutgoingConnections() minor improvements and fixes. * Minor refactoring. * Minor fixes. * [NOD-1313] GetPeerAddresses RPC message update * GetPeerAddresses RPC: add BannedAddresses in the separate field. * Update protobuf. * [NOD-1534] Update messages.pb.go Co-authored-by: Kirill <gammerxpower@gmail.com>
33 lines
894 B
Go
33 lines
894 B
Go
package addressmanager
|
|
|
|
import (
|
|
"net"
|
|
"strconv"
|
|
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// AddAddressByIP adds an address where we are given an ip:port and not a
|
|
// appmessage.NetAddress.
|
|
func AddAddressByIP(am *AddressManager, addressIP string, subnetworkID *externalapi.DomainSubnetworkID) error {
|
|
// Split IP and port
|
|
ipString, portString, err := net.SplitHostPort(addressIP)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
// Put it in appmessage.Netaddress
|
|
ip := net.ParseIP(ipString)
|
|
if ip == nil {
|
|
return errors.Errorf("invalid ip %s", ipString)
|
|
}
|
|
port, err := strconv.ParseUint(portString, 10, 0)
|
|
if err != nil {
|
|
return errors.Errorf("invalid port %s: %s", portString, err)
|
|
}
|
|
netAddress := appmessage.NewNetAddressIPPort(ip, uint16(port), 0)
|
|
am.AddAddresses(netAddress)
|
|
return nil
|
|
}
|