mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
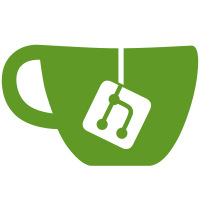
* [NOD-248] Implement waitgroup to enable waiting while adding * [NOD-248] fix waitGroup.done() error message * [NOD-248] atomically read wg.counter * [NOD-248] return lowPriorityMutex * [NOD-249] Add tests to waitgroup * [NOD-249] Change waitgroup to use channels * [NOD-249] Format project * [NOD-249] Add comments and logs to waitGroup, and remove timeouts from prioritymutex_test.go * [NOD-249] Fix comments
44 lines
790 B
Go
44 lines
790 B
Go
package locks
|
|
|
|
import (
|
|
"sync"
|
|
"sync/atomic"
|
|
)
|
|
|
|
type waitGroup struct {
|
|
counter int64
|
|
waitLock sync.Mutex
|
|
syncChannel chan struct{}
|
|
}
|
|
|
|
func newWaitGroup() *waitGroup {
|
|
return &waitGroup{
|
|
waitLock: sync.Mutex{},
|
|
syncChannel: make(chan struct{}),
|
|
}
|
|
}
|
|
|
|
func (wg *waitGroup) add() {
|
|
atomic.AddInt64(&wg.counter, 1)
|
|
}
|
|
|
|
func (wg *waitGroup) done() {
|
|
counter := atomic.AddInt64(&wg.counter, -1)
|
|
if counter < 0 {
|
|
panic("negative values for wg.counter are not allowed. This was likely caused by calling done() before add()")
|
|
}
|
|
if atomic.LoadInt64(&wg.counter) == 0 {
|
|
spawn(func() {
|
|
wg.syncChannel <- struct{}{}
|
|
})
|
|
}
|
|
}
|
|
|
|
func (wg *waitGroup) wait() {
|
|
wg.waitLock.Lock()
|
|
defer wg.waitLock.Unlock()
|
|
for atomic.LoadInt64(&wg.counter) != 0 {
|
|
<-wg.syncChannel
|
|
}
|
|
}
|