mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 15:26:42 +00:00
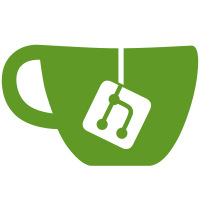
* [NOD-1579] Remove selected tip hash messages. * [NOD-1579] Start moving IBD stuff into blockrelay. * [NOD-1579] Rename relaytransactions to transactionrelay. * [NOD-1579] Move IBD files into blockrelay. * [NOD-1579] Remove flow stuff from ibd.go. * [NOD-1579] Bring back IsInIBD(). * [NOD-1579] Simplify block relay flow. * [NOD-1579] Check orphan pool for missing parents to avoid unnecessary processing. * [NOD-1579] Implement processOrphan. * [NOD-1579] Implement addToOrphanSetAndRequestMissingParents. * [NOD-1579] Fix TestIBD. * [NOD-1579] Implement isBlockInOrphanResolutionRange. * [NOD-1579] Implement limited block locators. * [NOD-1579] Add some comments. * [NOD-1579] Specifically check for StatusHeaderOnly in blockrelay. * [NOD-1579] Simplify runIBDIfNotRunning. * [NOD-1579] Don't run IBD if it is already running. * [NOD-1579] Fix a comment. * [NOD-1579] Rename mode to syncInfo. * [NOD-1579] Simplify validateAndInsertBlock. * [NOD-1579] Fix bad SyncStateSynced condition. * [NOD-1579] Implement validateAgainstSyncStateAndResolveInsertMode. * [NOD-1579] Use insertModeHeader. * [NOD-1579] Add logs to TrySetIBDRunning and UnsetIBDRunning. * [NOD-1579] Implement and use dequeueIncomingMessageAndSkipInvs. * [NOD-1579] Fix a log. * [NOD-1579] Fix a bug in createBlockLocator. * [NOD-1579] Rename a variable. * [NOD-1579] Fix a slew of bugs in missingBlockBodyHashes and selectedChildIterator. * [NOD-1579] Fix bad chunk size in syncMissingBlockBodies. * [NOD-1579] Remove maxOrphanBlueScoreDiff. * [NOD-1579] Fix merge errors. * [NOD-1579] Remove a debug log. * [NOD-1579] Add logs. * [NOD-1579] Make various go quality tools happy. * [NOD-1579] Fix a typo in a variable name. * [NOD-1579] Fix full blocks over header-only blocks not failing the missing-parents validation. * [NOD-1579] Add an error log about a condition that should never happen. * [NOD-1579] Check all antiPast hashes instead of just the lowHash's anticone to filter for header-only blocks. * [NOD-1579] Remove the nil stuff from GetBlockLocator. * [NOD-1579] Remove superfluous condition in handleRelayInvsFlow.start(). * [NOD-1579] Return a boolean from requestBlock instead of comparing to nil. * [NOD-1579] Fix a bad log.Debugf. * [NOD-1579] Remove a redundant check. * [NOD-1579] Change an info log to a warning log. * [NOD-1579] Move OnNewBlock out of relayBlock. * [NOD-1579] Remove redundant exists check from runIBDIfNotRunning. * [NOD-1579] Fix bad call to OnNewBlock. * [NOD-1579] Remove an impossible check. * [NOD-1579] Added a log. * [NOD-1579] Rename insertModeBlockWithoutUpdatingVirtual to insertModeBlockBody. * [NOD-1579] Add a check for duplicate headers. * [NOD-1579] Added a comment. * [NOD-1579] Tighten a stop condition. * [NOD-1579] Simplify a log. * [NOD-1579] Clarify a log. * [NOD-1579] Move a log.
163 lines
5.7 KiB
Go
163 lines
5.7 KiB
Go
package dagtopologymanager
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// dagTopologyManager exposes methods for querying relationships
|
|
// between blocks in the DAG
|
|
type dagTopologyManager struct {
|
|
reachabilityManager model.ReachabilityManager
|
|
blockRelationStore model.BlockRelationStore
|
|
databaseContext model.DBReader
|
|
}
|
|
|
|
// New instantiates a new DAGTopologyManager
|
|
func New(
|
|
databaseContext model.DBReader,
|
|
reachabilityManager model.ReachabilityManager,
|
|
blockRelationStore model.BlockRelationStore) model.DAGTopologyManager {
|
|
|
|
return &dagTopologyManager{
|
|
databaseContext: databaseContext,
|
|
reachabilityManager: reachabilityManager,
|
|
blockRelationStore: blockRelationStore,
|
|
}
|
|
}
|
|
|
|
// Parents returns the DAG parents of the given blockHash
|
|
func (dtm *dagTopologyManager) Parents(blockHash *externalapi.DomainHash) ([]*externalapi.DomainHash, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return blockRelations.Parents, nil
|
|
}
|
|
|
|
// Children returns the DAG children of the given blockHash
|
|
func (dtm *dagTopologyManager) Children(blockHash *externalapi.DomainHash) ([]*externalapi.DomainHash, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return blockRelations.Children, nil
|
|
}
|
|
|
|
// IsParentOf returns true if blockHashA is a direct DAG parent of blockHashB
|
|
func (dtm *dagTopologyManager) IsParentOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHashB)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
return isHashInSlice(blockHashA, blockRelations.Parents), nil
|
|
}
|
|
|
|
// IsChildOf returns true if blockHashA is a direct DAG child of blockHashB
|
|
func (dtm *dagTopologyManager) IsChildOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHashB)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
return isHashInSlice(blockHashA, blockRelations.Children), nil
|
|
}
|
|
|
|
// IsAncestorOf returns true if blockHashA is a DAG ancestor of blockHashB
|
|
func (dtm *dagTopologyManager) IsAncestorOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
return dtm.reachabilityManager.IsDAGAncestorOf(blockHashA, blockHashB)
|
|
}
|
|
|
|
// IsDescendantOf returns true if blockHashA is a DAG descendant of blockHashB
|
|
func (dtm *dagTopologyManager) IsDescendantOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
return dtm.reachabilityManager.IsDAGAncestorOf(blockHashB, blockHashA)
|
|
}
|
|
|
|
// IsAncestorOfAny returns true if `blockHash` is an ancestor of at least one of `potentialDescendants`
|
|
func (dtm *dagTopologyManager) IsAncestorOfAny(blockHash *externalapi.DomainHash, potentialDescendants []*externalapi.DomainHash) (bool, error) {
|
|
for _, potentialDescendant := range potentialDescendants {
|
|
isAncestorOf, err := dtm.IsAncestorOf(blockHash, potentialDescendant)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
|
|
if isAncestorOf {
|
|
return true, nil
|
|
}
|
|
}
|
|
|
|
return false, nil
|
|
}
|
|
|
|
// IsInSelectedParentChainOf returns true if blockHashA is in the selected parent chain of blockHashB
|
|
func (dtm *dagTopologyManager) IsInSelectedParentChainOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
return dtm.reachabilityManager.IsReachabilityTreeAncestorOf(blockHashA, blockHashB)
|
|
}
|
|
|
|
func isHashInSlice(hash *externalapi.DomainHash, hashes []*externalapi.DomainHash) bool {
|
|
for _, h := range hashes {
|
|
if *h == *hash {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (dtm *dagTopologyManager) SetParents(blockHash *externalapi.DomainHash, parentHashes []*externalapi.DomainHash) error {
|
|
hasRelations, err := dtm.blockRelationStore.Has(dtm.databaseContext, blockHash)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if hasRelations {
|
|
// Go over the block's current relations (if they exist), and remove the block from all its current parents
|
|
// Note: In theory we should also remove the block from all its children, however, in practice no block
|
|
// ever has its relations updated after getting any children, therefore we skip this step
|
|
|
|
currentRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHash)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, currentParent := range currentRelations.Parents {
|
|
parentRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, currentParent)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
for i, parentChild := range parentRelations.Children {
|
|
if *parentChild == *blockHash {
|
|
parentRelations.Children = append(parentRelations.Children[:i], parentRelations.Children[i+1:]...)
|
|
dtm.blockRelationStore.StageBlockRelation(currentParent, parentRelations)
|
|
break
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
// Go over all new parents and add block as their child
|
|
for _, parent := range parentHashes {
|
|
parentRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, parent)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
isBlockAlreadyInChildren := false
|
|
for _, parentChild := range parentRelations.Children {
|
|
if *parentChild == *blockHash {
|
|
isBlockAlreadyInChildren = true
|
|
break
|
|
}
|
|
}
|
|
if !isBlockAlreadyInChildren {
|
|
parentRelations.Children = append(parentRelations.Children, blockHash)
|
|
dtm.blockRelationStore.StageBlockRelation(parent, parentRelations)
|
|
}
|
|
}
|
|
|
|
// Finally - create the relations for the block itself
|
|
dtm.blockRelationStore.StageBlockRelation(blockHash, &model.BlockRelations{
|
|
Parents: parentHashes,
|
|
Children: []*externalapi.DomainHash{},
|
|
})
|
|
|
|
return nil
|
|
}
|