mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
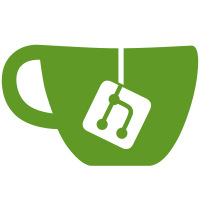
* [NOD-1223] Delete unused files/packages. * [NOD-1223] Move signal and limits to the os package. * [NOD-1223] Put database and dbaccess into the db package. * [NOD-1223] Fold the logs package into the logger package. * [NOD-1223] Rename domainmessage to appmessage. * [NOD-1223] Rename to/from DomainMessage to AppMessage. * [NOD-1223] Move appmessage to the app packge. * [NOD-1223] Move protocol to the app packge. * [NOD-1223] Move the network package to the infrastructure packge. * [NOD-1223] Rename cmd to executables. * [NOD-1223] Fix go.doc in the logger package.
75 lines
2.3 KiB
Go
75 lines
2.3 KiB
Go
package flowcontext
|
|
|
|
import (
|
|
"sync/atomic"
|
|
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/app/protocol/flows/blockrelay"
|
|
"github.com/kaspanet/kaspad/domain/blockdag"
|
|
"github.com/kaspanet/kaspad/util"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
)
|
|
|
|
// OnNewBlock updates the mempool after a new block arrival, and
|
|
// relays newly unorphaned transactions and possibly rebroadcast
|
|
// manually added transactions when not in IBD.
|
|
func (f *FlowContext) OnNewBlock(block *util.Block) error {
|
|
transactionsAcceptedToMempool, err := f.txPool.HandleNewBlock(block)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return f.broadcastTransactionsAfterBlockAdded(block, transactionsAcceptedToMempool)
|
|
}
|
|
|
|
func (f *FlowContext) broadcastTransactionsAfterBlockAdded(block *util.Block, transactionsAcceptedToMempool []*util.Tx) error {
|
|
f.updateTransactionsToRebroadcast(block)
|
|
|
|
// Don't relay transactions when in IBD.
|
|
if atomic.LoadUint32(&f.isInIBD) != 0 {
|
|
return nil
|
|
}
|
|
|
|
var txIDsToRebroadcast []*daghash.TxID
|
|
if f.shouldRebroadcastTransactions() {
|
|
txIDsToRebroadcast = f.txIDsToRebroadcast()
|
|
}
|
|
|
|
txIDsToBroadcast := make([]*daghash.TxID, len(transactionsAcceptedToMempool)+len(txIDsToRebroadcast))
|
|
for i, tx := range transactionsAcceptedToMempool {
|
|
txIDsToBroadcast[i] = tx.ID()
|
|
}
|
|
offset := len(transactionsAcceptedToMempool)
|
|
for i, txID := range txIDsToRebroadcast {
|
|
txIDsToBroadcast[offset+i] = txID
|
|
}
|
|
|
|
if len(txIDsToBroadcast) == 0 {
|
|
return nil
|
|
}
|
|
if len(txIDsToBroadcast) > appmessage.MaxInvPerTxInvMsg {
|
|
txIDsToBroadcast = txIDsToBroadcast[:appmessage.MaxInvPerTxInvMsg]
|
|
}
|
|
inv := appmessage.NewMsgInvTransaction(txIDsToBroadcast)
|
|
return f.Broadcast(inv)
|
|
}
|
|
|
|
// SharedRequestedBlocks returns a *blockrelay.SharedRequestedBlocks for sharing
|
|
// data about requested blocks between different peers.
|
|
func (f *FlowContext) SharedRequestedBlocks() *blockrelay.SharedRequestedBlocks {
|
|
return f.sharedRequestedBlocks
|
|
}
|
|
|
|
// AddBlock adds the given block to the DAG and propagates it.
|
|
func (f *FlowContext) AddBlock(block *util.Block, flags blockdag.BehaviorFlags) error {
|
|
_, _, err := f.DAG().ProcessBlock(block, flags)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
err = f.OnNewBlock(block)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return f.Broadcast(appmessage.NewMsgInvBlock(block.Hash()))
|
|
}
|