mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-05 12:22:30 +00:00
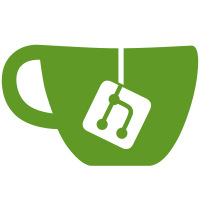
* [NOD-1579] Remove selected tip hash messages. * [NOD-1579] Start moving IBD stuff into blockrelay. * [NOD-1579] Rename relaytransactions to transactionrelay. * [NOD-1579] Move IBD files into blockrelay. * [NOD-1579] Remove flow stuff from ibd.go. * [NOD-1579] Bring back IsInIBD(). * [NOD-1579] Simplify block relay flow. * [NOD-1579] Check orphan pool for missing parents to avoid unnecessary processing. * [NOD-1579] Implement processOrphan. * [NOD-1579] Implement addToOrphanSetAndRequestMissingParents. * [NOD-1579] Fix TestIBD. * [NOD-1579] Implement isBlockInOrphanResolutionRange. * [NOD-1579] Implement limited block locators. * [NOD-1579] Add some comments. * [NOD-1579] Specifically check for StatusHeaderOnly in blockrelay. * [NOD-1579] Simplify runIBDIfNotRunning. * [NOD-1579] Don't run IBD if it is already running. * [NOD-1579] Fix a comment. * [NOD-1579] Rename mode to syncInfo. * [NOD-1579] Simplify validateAndInsertBlock. * [NOD-1579] Fix bad SyncStateSynced condition. * [NOD-1579] Implement validateAgainstSyncStateAndResolveInsertMode. * [NOD-1579] Use insertModeHeader. * [NOD-1579] Add logs to TrySetIBDRunning and UnsetIBDRunning. * [NOD-1579] Implement and use dequeueIncomingMessageAndSkipInvs. * [NOD-1579] Fix a log. * [NOD-1579] Fix a bug in createBlockLocator. * [NOD-1579] Rename a variable. * [NOD-1579] Fix a slew of bugs in missingBlockBodyHashes and selectedChildIterator. * [NOD-1579] Fix bad chunk size in syncMissingBlockBodies. * [NOD-1579] Remove maxOrphanBlueScoreDiff. * [NOD-1579] Fix merge errors. * [NOD-1579] Remove a debug log. * [NOD-1579] Add logs. * [NOD-1579] Make various go quality tools happy. * [NOD-1579] Fix a typo in a variable name. * [NOD-1579] Fix full blocks over header-only blocks not failing the missing-parents validation. * [NOD-1579] Add an error log about a condition that should never happen. * [NOD-1579] Check all antiPast hashes instead of just the lowHash's anticone to filter for header-only blocks. * [NOD-1579] Remove the nil stuff from GetBlockLocator. * [NOD-1579] Remove superfluous condition in handleRelayInvsFlow.start(). * [NOD-1579] Return a boolean from requestBlock instead of comparing to nil. * [NOD-1579] Fix a bad log.Debugf. * [NOD-1579] Remove a redundant check. * [NOD-1579] Change an info log to a warning log. * [NOD-1579] Move OnNewBlock out of relayBlock. * [NOD-1579] Remove redundant exists check from runIBDIfNotRunning. * [NOD-1579] Fix bad call to OnNewBlock. * [NOD-1579] Remove an impossible check. * [NOD-1579] Added a log. * [NOD-1579] Rename insertModeBlockWithoutUpdatingVirtual to insertModeBlockBody. * [NOD-1579] Add a check for duplicate headers. * [NOD-1579] Added a comment. * [NOD-1579] Tighten a stop condition. * [NOD-1579] Simplify a log. * [NOD-1579] Clarify a log. * [NOD-1579] Move a log.
145 lines
3.9 KiB
Go
145 lines
3.9 KiB
Go
package peer
|
|
|
|
import (
|
|
"sync"
|
|
"time"
|
|
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter"
|
|
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/id"
|
|
mathUtil "github.com/kaspanet/kaspad/util/math"
|
|
"github.com/kaspanet/kaspad/util/mstime"
|
|
)
|
|
|
|
// maxProtocolVersion version is the maximum supported protocol
|
|
// version this kaspad node supports
|
|
const maxProtocolVersion = 1
|
|
|
|
// Peer holds data about a peer.
|
|
type Peer struct {
|
|
connection *netadapter.NetConnection
|
|
|
|
userAgent string
|
|
services appmessage.ServiceFlag
|
|
advertisedProtocolVerion uint32 // protocol version advertised by remote
|
|
protocolVersion uint32 // negotiated protocol version
|
|
disableRelayTx bool
|
|
subnetworkID *externalapi.DomainSubnetworkID
|
|
|
|
timeOffset time.Duration
|
|
connectionStarted time.Time
|
|
|
|
pingLock sync.RWMutex
|
|
lastPingNonce uint64 // The nonce of the last ping we sent
|
|
lastPingTime time.Time // Time we sent last ping
|
|
lastPingDuration time.Duration // Time for last ping to return
|
|
}
|
|
|
|
// New returns a new Peer
|
|
func New(connection *netadapter.NetConnection) *Peer {
|
|
return &Peer{
|
|
connection: connection,
|
|
connectionStarted: time.Now(),
|
|
}
|
|
}
|
|
|
|
// Connection returns the NetConnection associated with this peer
|
|
func (p *Peer) Connection() *netadapter.NetConnection {
|
|
return p.connection
|
|
}
|
|
|
|
// SubnetworkID returns the subnetwork the peer is associated with.
|
|
// It is nil in full nodes.
|
|
func (p *Peer) SubnetworkID() *externalapi.DomainSubnetworkID {
|
|
return p.subnetworkID
|
|
}
|
|
|
|
// ID returns the peer ID.
|
|
func (p *Peer) ID() *id.ID {
|
|
return p.connection.ID()
|
|
}
|
|
|
|
// TimeOffset returns the peer's time offset.
|
|
func (p *Peer) TimeOffset() time.Duration {
|
|
return p.timeOffset
|
|
}
|
|
|
|
// UserAgent returns the peer's user agent.
|
|
func (p *Peer) UserAgent() string {
|
|
return p.userAgent
|
|
}
|
|
|
|
// AdvertisedProtocolVersion returns the peer's advertised protocol version.
|
|
func (p *Peer) AdvertisedProtocolVersion() uint32 {
|
|
return p.advertisedProtocolVerion
|
|
}
|
|
|
|
// TimeConnected returns the time since the connection to this been has been started.
|
|
func (p *Peer) TimeConnected() time.Duration {
|
|
return time.Since(p.connectionStarted)
|
|
}
|
|
|
|
// IsOutbound returns whether the peer is an outbound connection.
|
|
func (p *Peer) IsOutbound() bool {
|
|
return p.connection.IsOutbound()
|
|
}
|
|
|
|
// UpdateFieldsFromMsgVersion updates the peer with the data from the version message.
|
|
func (p *Peer) UpdateFieldsFromMsgVersion(msg *appmessage.MsgVersion) {
|
|
// Negotiate the protocol version.
|
|
p.advertisedProtocolVerion = msg.ProtocolVersion
|
|
p.protocolVersion = mathUtil.MinUint32(maxProtocolVersion, p.advertisedProtocolVerion)
|
|
log.Debugf("Negotiated protocol version %d for peer %s",
|
|
p.protocolVersion, p)
|
|
|
|
// Set the supported services for the peer to what the remote peer
|
|
// advertised.
|
|
p.services = msg.Services
|
|
|
|
// Set the remote peer's user agent.
|
|
p.userAgent = msg.UserAgent
|
|
|
|
p.disableRelayTx = msg.DisableRelayTx
|
|
p.subnetworkID = msg.SubnetworkID
|
|
|
|
p.timeOffset = mstime.Since(msg.Timestamp)
|
|
}
|
|
|
|
// SetPingPending sets the ping state of the peer to 'pending'
|
|
func (p *Peer) SetPingPending(nonce uint64) {
|
|
p.pingLock.Lock()
|
|
defer p.pingLock.Unlock()
|
|
|
|
p.lastPingNonce = nonce
|
|
p.lastPingTime = time.Now()
|
|
}
|
|
|
|
// SetPingIdle sets the ping state of the peer to 'idle'
|
|
func (p *Peer) SetPingIdle() {
|
|
p.pingLock.Lock()
|
|
defer p.pingLock.Unlock()
|
|
|
|
p.lastPingNonce = 0
|
|
p.lastPingDuration = time.Since(p.lastPingTime)
|
|
}
|
|
|
|
func (p *Peer) String() string {
|
|
return p.connection.String()
|
|
}
|
|
|
|
// Address returns the address associated with this connection
|
|
func (p *Peer) Address() string {
|
|
return p.connection.Address()
|
|
}
|
|
|
|
// LastPingDuration returns the duration of the last ping to
|
|
// this peer
|
|
func (p *Peer) LastPingDuration() time.Duration {
|
|
p.pingLock.Lock()
|
|
defer p.pingLock.Unlock()
|
|
|
|
return p.lastPingDuration
|
|
}
|