mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 06:12:32 +00:00
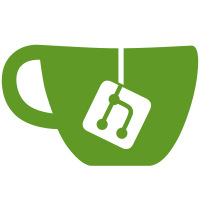
* [NOD-1579] Rename AcceptedTxIDs to AcceptedTransactionIDs. * [NOD-1579] Add InsertBlockResult to ValidateAndInsertBlock results. * [NOD-1593] Rename InsertBlockResult to BlockInsertionResult. * [NOD-1593] Add SelectedParentChainChanges to AddBlockToVirtual's result. * [NOD-1593] Implement findSelectedParentChainChanges. * [NOD-1593] Implement TestFindSelectedParentChainChanges. * [NOD-1593] Fix a string. * [NOD-1593] Finish implementing TestFindSelectedParentChainChanges. * [NOD-1593] Fix merge errors. * [NOD-1593] Fix merge errors. * [NOD-1593] Rename findSelectedParentChainChanges to calculateSelectedParentChainChanges. * [NOD-1593] Expand TestCalculateSelectedParentChainChanges.
70 lines
2.1 KiB
Go
70 lines
2.1 KiB
Go
package appmessage
|
|
|
|
// NotifyChainChangedRequestMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type NotifyChainChangedRequestMessage struct {
|
|
baseMessage
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *NotifyChainChangedRequestMessage) Command() MessageCommand {
|
|
return CmdNotifyChainChangedRequestMessage
|
|
}
|
|
|
|
// NewNotifyChainChangedRequestMessage returns a instance of the message
|
|
func NewNotifyChainChangedRequestMessage() *NotifyChainChangedRequestMessage {
|
|
return &NotifyChainChangedRequestMessage{}
|
|
}
|
|
|
|
// NotifyChainChangedResponseMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type NotifyChainChangedResponseMessage struct {
|
|
baseMessage
|
|
Error *RPCError
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *NotifyChainChangedResponseMessage) Command() MessageCommand {
|
|
return CmdNotifyChainChangedResponseMessage
|
|
}
|
|
|
|
// NewNotifyChainChangedResponseMessage returns a instance of the message
|
|
func NewNotifyChainChangedResponseMessage() *NotifyChainChangedResponseMessage {
|
|
return &NotifyChainChangedResponseMessage{}
|
|
}
|
|
|
|
// ChainChangedNotificationMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type ChainChangedNotificationMessage struct {
|
|
baseMessage
|
|
RemovedChainBlockHashes []string
|
|
AddedChainBlocks []*ChainBlock
|
|
}
|
|
|
|
// ChainBlock represents a DAG chain-block
|
|
type ChainBlock struct {
|
|
Hash string
|
|
AcceptedBlocks []*AcceptedBlock
|
|
}
|
|
|
|
// AcceptedBlock represents a block accepted into the DAG
|
|
type AcceptedBlock struct {
|
|
Hash string
|
|
AcceptedTransactionIDs []string
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *ChainChangedNotificationMessage) Command() MessageCommand {
|
|
return CmdChainChangedNotificationMessage
|
|
}
|
|
|
|
// NewChainChangedNotificationMessage returns a instance of the message
|
|
func NewChainChangedNotificationMessage(removedChainBlockHashes []string,
|
|
addedChainBlocks []*ChainBlock) *ChainChangedNotificationMessage {
|
|
|
|
return &ChainChangedNotificationMessage{
|
|
RemovedChainBlockHashes: removedChainBlockHashes,
|
|
AddedChainBlocks: addedChainBlocks,
|
|
}
|
|
}
|