mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
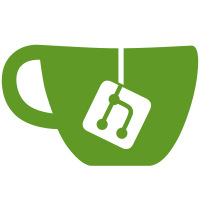
This commit modifies the error return type for errors during script validation to use the RuleError so they are consistent with the rest of the errors. This also helps the calling code differentiate blocks rejected due to script parsing and validation errors as opposed to internal issues such as inability to read from the disk. To accomplish this, two new two new RuleErrors, ErrScriptMalformed and ErrScriptValidation, have been added. Also, the errors for script parsing issues and script validation errors have been improved to include both transaction hashes and indexes involved in the validation effort. Previously script parsing issues had almost no additional information as to which transaction input/outputs the failing script came from.
93 lines
3.0 KiB
Go
93 lines
3.0 KiB
Go
// Copyright (c) 2014 Conformal Systems LLC.
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package btcchain_test
|
|
|
|
import (
|
|
"github.com/conformal/btcchain"
|
|
"testing"
|
|
)
|
|
|
|
// TestErrorCodeStringer tests the stringized output for the ErrorCode type.
|
|
func TestErrorCodeStringer(t *testing.T) {
|
|
tests := []struct {
|
|
in btcchain.ErrorCode
|
|
want string
|
|
}{
|
|
{btcchain.ErrDuplicateBlock, "ErrDuplicateBlock"},
|
|
{btcchain.ErrBlockVersionTooOld, "ErrBlockVersionTooOld"},
|
|
{btcchain.ErrInvalidTime, "ErrInvalidTime"},
|
|
{btcchain.ErrTimeTooOld, "ErrTimeTooOld"},
|
|
{btcchain.ErrTimeTooNew, "ErrTimeTooNew"},
|
|
{btcchain.ErrDifficultyTooLow, "ErrDifficultyTooLow"},
|
|
{btcchain.ErrUnexpectedDifficulty, "ErrUnexpectedDifficulty"},
|
|
{btcchain.ErrHighHash, "ErrHighHash"},
|
|
{btcchain.ErrBadMerkleRoot, "ErrBadMerkleRoot"},
|
|
{btcchain.ErrBadCheckpoint, "ErrBadCheckpoint"},
|
|
{btcchain.ErrForkTooOld, "ErrForkTooOld"},
|
|
{btcchain.ErrNoTransactions, "ErrNoTransactions"},
|
|
{btcchain.ErrNoTxInputs, "ErrNoTxInputs"},
|
|
{btcchain.ErrNoTxOutputs, "ErrNoTxOutputs"},
|
|
{btcchain.ErrBadTxOutValue, "ErrBadTxOutValue"},
|
|
{btcchain.ErrDuplicateTxInputs, "ErrDuplicateTxInputs"},
|
|
{btcchain.ErrBadTxInput, "ErrBadTxInput"},
|
|
{btcchain.ErrBadCheckpoint, "ErrBadCheckpoint"},
|
|
{btcchain.ErrMissingTx, "ErrMissingTx"},
|
|
{btcchain.ErrUnfinalizedTx, "ErrUnfinalizedTx"},
|
|
{btcchain.ErrDuplicateTx, "ErrDuplicateTx"},
|
|
{btcchain.ErrOverwriteTx, "ErrOverwriteTx"},
|
|
{btcchain.ErrImmatureSpend, "ErrImmatureSpend"},
|
|
{btcchain.ErrDoubleSpend, "ErrDoubleSpend"},
|
|
{btcchain.ErrSpendTooHigh, "ErrSpendTooHigh"},
|
|
{btcchain.ErrBadFees, "ErrBadFees"},
|
|
{btcchain.ErrTooManySigOps, "ErrTooManySigOps"},
|
|
{btcchain.ErrFirstTxNotCoinbase, "ErrFirstTxNotCoinbase"},
|
|
{btcchain.ErrMultipleCoinbases, "ErrMultipleCoinbases"},
|
|
{btcchain.ErrBadCoinbaseScriptLen, "ErrBadCoinbaseScriptLen"},
|
|
{btcchain.ErrBadCoinbaseValue, "ErrBadCoinbaseValue"},
|
|
{btcchain.ErrMissingCoinbaseHeight, "ErrMissingCoinbaseHeight"},
|
|
{btcchain.ErrBadCoinbaseHeight, "ErrBadCoinbaseHeight"},
|
|
{btcchain.ErrScriptMalformed, "ErrScriptMalformed"},
|
|
{btcchain.ErrScriptValidation, "ErrScriptValidation"},
|
|
{0xffff, "Unknown ErrorCode (65535)"},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
result := test.in.String()
|
|
if result != test.want {
|
|
t.Errorf("String #%d\n got: %s want: %s", i, result,
|
|
test.want)
|
|
continue
|
|
}
|
|
}
|
|
}
|
|
|
|
// TestRuleError tests the error output for the RuleError type.
|
|
func TestRuleError(t *testing.T) {
|
|
tests := []struct {
|
|
in btcchain.RuleError
|
|
want string
|
|
}{
|
|
{
|
|
btcchain.RuleError{Description: "duplicate block"},
|
|
"duplicate block",
|
|
},
|
|
{
|
|
btcchain.RuleError{Description: "human-readable error"},
|
|
"human-readable error",
|
|
},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
result := test.in.Error()
|
|
if result != test.want {
|
|
t.Errorf("Error #%d\n got: %s want: %s", i, result,
|
|
test.want)
|
|
continue
|
|
}
|
|
}
|
|
}
|