mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-01 18:32:32 +00:00
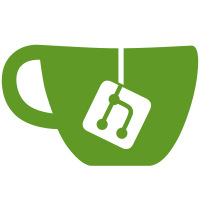
* [NOD-849] Cover ffldb/transaction with tests. * [NOD-849] Cover cursor.go with tests. * [NOD-849] Cover ldb/transaction with tests. * [NOD-849] Cover location.go with tests. * [NOD-849] Write TestFlatFileMultiFileRollback. * [NOD-849] Fix merge errors. * [NOD-849] Fix a comment. * [NOD-849] Fix a comment. * [NOD-849] Add a test that makes sure that files get deleted on rollback. * [NOD-849] Add a test that makes sure that serializeLocation serialized to an expected value. * [NOD-849] Improve TestFlatFileLocationDeserializationErrors. * [NOD-849] Fix a copy+paste error. * [NOD-849] Explain maxFileSize = 16. * [NOD-849] Remove redundant RollbackUnlessClosed call. * [NOD-849] Extract bucket to a variable in TestCursorSanity. * [NOD-849] Rename TestKeyValueTransactionCommit to TestTransactionCommitForLevelDBMethods. * [NOD-849] Extract prepareXXX into separate functions. * [NOD-849] Simplify function calls in TestTransactionCloseErrors. * [NOD-849] Extract validateCurrentCursorKeyAndValue to a separate function. * [NOD-849] Add a comment over TestCursorSanity. * [NOD-849] Add a comment over function in TestCursorCloseErrors. * [NOD-849] Add a comment over function in TestTransactionCloseErrors. * [NOD-849] Separate TestTransactionCloseErrors to TestTransactionCommitErrors and TestTransactionRollbackErrors. * [NOD-849] Separate TestTransactionCloseErrors to TestTransactionCommitErrors and TestTransactionRollbackErrors. * [NOD-849] Fix copy+paste error in comments. * [NOD-849] Fix merge errors. * [NOD-849] Merge TestTransactionCommitErrors and TestTransactionRollbackErrors into TestTransactionCloseErrors. * [NOD-849] Move prepareDatabaseForTest into ffldb_test.go. * [NOD-849] Add cursorKey to Value error messages in validateCurrentCursorKeyAndValue.
132 lines
3.5 KiB
Go
132 lines
3.5 KiB
Go
package ffldb
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/database"
|
|
"io/ioutil"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func prepareDatabaseForTest(t *testing.T, testName string) (db database.Database, teardownFunc func()) {
|
|
// Create a temp db to run tests against
|
|
path, err := ioutil.TempDir("", testName)
|
|
if err != nil {
|
|
t.Fatalf("%s: TempDir unexpectedly "+
|
|
"failed: %s", testName, err)
|
|
}
|
|
db, err = Open(path)
|
|
if err != nil {
|
|
t.Fatalf("%s: Open unexpectedly "+
|
|
"failed: %s", testName, err)
|
|
}
|
|
teardownFunc = func() {
|
|
err = db.Close()
|
|
if err != nil {
|
|
t.Fatalf("%s: Close unexpectedly "+
|
|
"failed: %s", testName, err)
|
|
}
|
|
}
|
|
return db, teardownFunc
|
|
}
|
|
|
|
func TestRepairFlatFiles(t *testing.T) {
|
|
// Create a temp db to run tests against
|
|
path, err := ioutil.TempDir("", "TestRepairFlatFiles")
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: TempDir unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
db, err := Open(path)
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: Open unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
isOpen := true
|
|
defer func() {
|
|
if isOpen {
|
|
err := db.Close()
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: Close unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
}
|
|
}()
|
|
|
|
// Cast to ffldb since we're going to be messing with its internals
|
|
ffldbInstance, ok := db.(*ffldb)
|
|
if !ok {
|
|
t.Fatalf("TestRepairFlatFiles: unexpectedly can't cast " +
|
|
"db to ffldb")
|
|
}
|
|
|
|
// Append data to the same store
|
|
storeName := "test"
|
|
_, err = ffldbInstance.AppendToStore(storeName, []byte("data1"))
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: AppendToStore unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
|
|
// Grab the current location to test against later
|
|
oldCurrentLocation, err := ffldbInstance.flatFileDB.CurrentLocation(storeName)
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: CurrentStoreLocation "+
|
|
"unexpectedly failed: %s", err)
|
|
}
|
|
|
|
// Append more data to the same store. We expect this to disappear later.
|
|
location2, err := ffldbInstance.AppendToStore(storeName, []byte("data2"))
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: AppendToStore unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
|
|
// Manually update the current location to point to the first piece of data
|
|
err = setCurrentStoreLocation(ffldbInstance, storeName, oldCurrentLocation)
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: setCurrentStoreLocation "+
|
|
"unexpectedly failed: %s", err)
|
|
}
|
|
|
|
// Reopen the database
|
|
err = ffldbInstance.Close()
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: Close unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
isOpen = false
|
|
db, err = Open(path)
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: Open unexpectedly "+
|
|
"failed: %s", err)
|
|
}
|
|
isOpen = true
|
|
ffldbInstance, ok = db.(*ffldb)
|
|
if !ok {
|
|
t.Fatalf("TestRepairFlatFiles: unexpectedly can't cast " +
|
|
"db to ffldb")
|
|
}
|
|
|
|
// Make sure that the current location rolled back as expected
|
|
currentLocation, err := ffldbInstance.flatFileDB.CurrentLocation(storeName)
|
|
if err != nil {
|
|
t.Fatalf("TestRepairFlatFiles: CurrentStoreLocation "+
|
|
"unexpectedly failed: %s", err)
|
|
}
|
|
if !reflect.DeepEqual(oldCurrentLocation, currentLocation) {
|
|
t.Fatalf("TestRepairFlatFiles: currentLocation did " +
|
|
"not roll back")
|
|
}
|
|
|
|
// Make sure that we can't get data that no longer exists
|
|
_, err = ffldbInstance.RetrieveFromStore(storeName, location2)
|
|
if err == nil {
|
|
t.Fatalf("TestRepairFlatFiles: RetrieveFromStore " +
|
|
"unexpectedly succeeded")
|
|
}
|
|
if !database.IsNotFoundError(err) {
|
|
t.Fatalf("TestRepairFlatFiles: RetrieveFromStore "+
|
|
"returned wrong error: %s", err)
|
|
}
|
|
}
|