mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
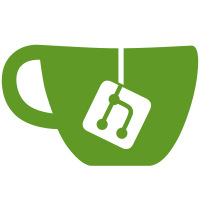
* [NOD-1130] Delete rpcadapters.go. * [NOD-1130] Delete p2p. Move rpc to top level. * [NOD-1130] Remove DAGParams from rpcserverConfig. * [NOD-1130] Remove rpcserverPeer, rpcserverConnManager, rpcserverSyncManager, and rpcserverConfig. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove connmgr and peer. * [NOD-1130] Move rpcmodel into rpc. * [NOD-1130] Implement ConnectionCount. * [NOD-1130] Remove ping and node RPC commands. * [NOD-1130] Dummify handleGetNetTotals. * [NOD-1130] Add NetConnection to Peer. * [NOD-1130] Fix merge errors. * [NOD-1130] Implement Peers. * [NOD-1130] Fix HandleGetConnectedPeerInfo. * [NOD-1130] Fix SendRawTransaction. * [NOD-1130] Rename addManualNode to connect and removeManualNode to disconnect. * [NOD-1130] Add a stub for AddBlock. * [NOD-1130] Fix tests. * [NOD-1130] Replace half-baked contents of RemoveConnection with a stub. * [NOD-1130] Fix merge errors. * [NOD-1130] Make golint happy. * [NOD-1130] Get rid of something weird. * [NOD-1130] Rename minerClient back to client. * [NOD-1130] Add a few fields to GetConnectedPeerInfoResult. * [NOD-1130] Rename oneTry to isPermanent. * [NOD-1130] Implement ConnectionCount in NetAdapter. * [NOD-1130] Move RawMempoolVerbose out of mempool. * [NOD-1130] Move isSynced into the mining package. * [NOD-1130] Fix a compilation error. * [NOD-1130] Make golint happy. * [NOD-1130] Fix merge errors.
109 lines
2.8 KiB
Go
109 lines
2.8 KiB
Go
package rpc
|
|
|
|
import (
|
|
"bufio"
|
|
"bytes"
|
|
"encoding/hex"
|
|
|
|
"github.com/kaspanet/kaspad/rpc/model"
|
|
"github.com/kaspanet/kaspad/util"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
"github.com/kaspanet/kaspad/util/subnetworkid"
|
|
)
|
|
|
|
// handleGetBlock implements the getBlock command.
|
|
func handleGetBlock(s *Server, cmd interface{}, closeChan <-chan struct{}) (interface{}, error) {
|
|
c := cmd.(*model.GetBlockCmd)
|
|
|
|
// Load the raw block bytes from the database.
|
|
hash, err := daghash.NewHashFromStr(c.Hash)
|
|
if err != nil {
|
|
return nil, rpcDecodeHexError(c.Hash)
|
|
}
|
|
|
|
// Return an appropriate error if the block is known to be invalid
|
|
if s.dag.IsKnownInvalid(hash) {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCBlockInvalid,
|
|
Message: "Block is known to be invalid",
|
|
}
|
|
}
|
|
|
|
// Return an appropriate error if the block is an orphan
|
|
if s.dag.IsKnownOrphan(hash) {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCOrphanBlock,
|
|
Message: "Block is an orphan",
|
|
}
|
|
}
|
|
|
|
block, err := s.dag.BlockByHash(hash)
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCBlockNotFound,
|
|
Message: "Block not found",
|
|
}
|
|
}
|
|
blockBytes, err := block.Bytes()
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCBlockInvalid,
|
|
Message: "Cannot serialize block",
|
|
}
|
|
}
|
|
|
|
// Handle partial blocks
|
|
if c.Subnetwork != nil {
|
|
requestSubnetworkID, err := subnetworkid.NewFromStr(*c.Subnetwork)
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidRequest.Code,
|
|
Message: "invalid subnetwork string",
|
|
}
|
|
}
|
|
nodeSubnetworkID := s.cfg.SubnetworkID
|
|
|
|
if requestSubnetworkID != nil {
|
|
if nodeSubnetworkID != nil {
|
|
if !nodeSubnetworkID.IsEqual(requestSubnetworkID) {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidRequest.Code,
|
|
Message: "subnetwork does not match this partial node",
|
|
}
|
|
}
|
|
// nothing to do - partial node stores partial blocks
|
|
} else {
|
|
// Deserialize the block.
|
|
msgBlock := block.MsgBlock()
|
|
msgBlock.ConvertToPartial(requestSubnetworkID)
|
|
var b bytes.Buffer
|
|
msgBlock.Serialize(bufio.NewWriter(&b))
|
|
blockBytes = b.Bytes()
|
|
}
|
|
}
|
|
}
|
|
|
|
// When the verbose flag is set to false, simply return the serialized block
|
|
// as a hex-encoded string (verbose flag is on by default).
|
|
if c.Verbose != nil && !*c.Verbose {
|
|
return hex.EncodeToString(blockBytes), nil
|
|
}
|
|
|
|
// The verbose flag is set, so generate the JSON object and return it.
|
|
|
|
// Deserialize the block.
|
|
block, err = util.NewBlockFromBytes(blockBytes)
|
|
if err != nil {
|
|
context := "Failed to deserialize block"
|
|
return nil, internalRPCError(err.Error(), context)
|
|
}
|
|
|
|
s.dag.RLock()
|
|
defer s.dag.RUnlock()
|
|
blockReply, err := buildGetBlockVerboseResult(s, block, c.VerboseTx == nil || !*c.VerboseTx)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return blockReply, nil
|
|
}
|