mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-05 12:22:30 +00:00
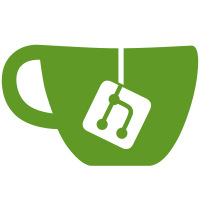
* [NOD-1225] Rename wire to domainmessage * [NOD-1225] Get rid of references to package wire in the code, and get rid of InvType
47 lines
1.2 KiB
Go
47 lines
1.2 KiB
Go
package rpc
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/hex"
|
|
"github.com/kaspanet/kaspad/domainmessage"
|
|
"github.com/kaspanet/kaspad/mempool"
|
|
"github.com/kaspanet/kaspad/rpc/model"
|
|
"github.com/kaspanet/kaspad/util"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// handleSendRawTransaction implements the sendRawTransaction command.
|
|
func handleSendRawTransaction(s *Server, cmd interface{}, closeChan <-chan struct{}) (interface{}, error) {
|
|
c := cmd.(*model.SendRawTransactionCmd)
|
|
// Deserialize and send off to tx relay
|
|
hexStr := c.HexTx
|
|
serializedTx, err := hex.DecodeString(hexStr)
|
|
if err != nil {
|
|
return nil, rpcDecodeHexError(hexStr)
|
|
}
|
|
var msgTx domainmessage.MsgTx
|
|
err = msgTx.Deserialize(bytes.NewReader(serializedTx))
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCDeserialization,
|
|
Message: "TX decode failed: " + err.Error(),
|
|
}
|
|
}
|
|
|
|
tx := util.NewTx(&msgTx)
|
|
err = s.protocolManager.AddTransaction(tx)
|
|
if err != nil {
|
|
if !errors.As(err, &mempool.RuleError{}) {
|
|
panic(err)
|
|
}
|
|
|
|
log.Debugf("Rejected transaction %s: %s", tx.ID(), err)
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCVerify,
|
|
Message: "TX rejected: " + err.Error(),
|
|
}
|
|
}
|
|
|
|
return tx.ID().String(), nil
|
|
}
|