mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-08 22:02:34 +00:00
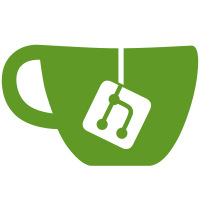
* [NOD-1130] Delete rpcadapters.go. * [NOD-1130] Delete p2p. Move rpc to top level. * [NOD-1130] Remove DAGParams from rpcserverConfig. * [NOD-1130] Remove rpcserverPeer, rpcserverConnManager, rpcserverSyncManager, and rpcserverConfig. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove connmgr and peer. * [NOD-1130] Move rpcmodel into rpc. * [NOD-1130] Implement ConnectionCount. * [NOD-1130] Remove ping and node RPC commands. * [NOD-1130] Dummify handleGetNetTotals. * [NOD-1130] Add NetConnection to Peer. * [NOD-1130] Fix merge errors. * [NOD-1130] Implement Peers. * [NOD-1130] Fix HandleGetConnectedPeerInfo. * [NOD-1130] Fix SendRawTransaction. * [NOD-1130] Rename addManualNode to connect and removeManualNode to disconnect. * [NOD-1130] Add a stub for AddBlock. * [NOD-1130] Fix tests. * [NOD-1130] Replace half-baked contents of RemoveConnection with a stub. * [NOD-1130] Fix merge errors. * [NOD-1130] Make golint happy. * [NOD-1130] Get rid of something weird. * [NOD-1130] Rename minerClient back to client. * [NOD-1130] Add a few fields to GetConnectedPeerInfoResult. * [NOD-1130] Rename oneTry to isPermanent. * [NOD-1130] Implement ConnectionCount in NetAdapter. * [NOD-1130] Move RawMempoolVerbose out of mempool. * [NOD-1130] Move isSynced into the mining package. * [NOD-1130] Fix a compilation error. * [NOD-1130] Make golint happy. * [NOD-1130] Fix merge errors.
81 lines
2.0 KiB
Go
81 lines
2.0 KiB
Go
// Copyright (c) 2014 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package model_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/kaspanet/kaspad/rpc/model"
|
|
)
|
|
|
|
// TestErrorCodeStringer tests the stringized output for the ErrorCode type.
|
|
func TestErrorCodeStringer(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
tests := []struct {
|
|
in model.ErrorCode
|
|
want string
|
|
}{
|
|
{model.ErrDuplicateMethod, "ErrDuplicateMethod"},
|
|
{model.ErrInvalidUsageFlags, "ErrInvalidUsageFlags"},
|
|
{model.ErrInvalidType, "ErrInvalidType"},
|
|
{model.ErrEmbeddedType, "ErrEmbeddedType"},
|
|
{model.ErrUnexportedField, "ErrUnexportedField"},
|
|
{model.ErrUnsupportedFieldType, "ErrUnsupportedFieldType"},
|
|
{model.ErrNonOptionalField, "ErrNonOptionalField"},
|
|
{model.ErrNonOptionalDefault, "ErrNonOptionalDefault"},
|
|
{model.ErrMismatchedDefault, "ErrMismatchedDefault"},
|
|
{model.ErrUnregisteredMethod, "ErrUnregisteredMethod"},
|
|
{model.ErrNumParams, "ErrNumParams"},
|
|
{model.ErrMissingDescription, "ErrMissingDescription"},
|
|
{0xffff, "Unknown ErrorCode (65535)"},
|
|
}
|
|
|
|
// Detect additional error codes that don't have the stringer added.
|
|
if len(tests)-1 != int(model.TstNumErrorCodes) {
|
|
t.Errorf("It appears an error code was added without adding an " +
|
|
"associated stringer test")
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
result := test.in.String()
|
|
if result != test.want {
|
|
t.Errorf("String #%d\n got: %s want: %s", i, result,
|
|
test.want)
|
|
continue
|
|
}
|
|
}
|
|
}
|
|
|
|
// TestError tests the error output for the Error type.
|
|
func TestError(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
tests := []struct {
|
|
in model.Error
|
|
want string
|
|
}{
|
|
{
|
|
model.Error{Description: "some error"},
|
|
"some error",
|
|
},
|
|
{
|
|
model.Error{Description: "human-readable error"},
|
|
"human-readable error",
|
|
},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
result := test.in.Error()
|
|
if result != test.want {
|
|
t.Errorf("Error #%d\n got: %s want: %s", i, result,
|
|
test.want)
|
|
continue
|
|
}
|
|
}
|
|
}
|