mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 06:12:32 +00:00
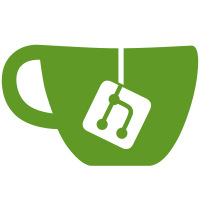
* [NOD-1130] Delete rpcadapters.go. * [NOD-1130] Delete p2p. Move rpc to top level. * [NOD-1130] Remove DAGParams from rpcserverConfig. * [NOD-1130] Remove rpcserverPeer, rpcserverConnManager, rpcserverSyncManager, and rpcserverConfig. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove connmgr and peer. * [NOD-1130] Move rpcmodel into rpc. * [NOD-1130] Implement ConnectionCount. * [NOD-1130] Remove ping and node RPC commands. * [NOD-1130] Dummify handleGetNetTotals. * [NOD-1130] Add NetConnection to Peer. * [NOD-1130] Fix merge errors. * [NOD-1130] Implement Peers. * [NOD-1130] Fix HandleGetConnectedPeerInfo. * [NOD-1130] Fix SendRawTransaction. * [NOD-1130] Rename addManualNode to connect and removeManualNode to disconnect. * [NOD-1130] Add a stub for AddBlock. * [NOD-1130] Fix tests. * [NOD-1130] Replace half-baked contents of RemoveConnection with a stub. * [NOD-1130] Fix merge errors. * [NOD-1130] Make golint happy. * [NOD-1130] Get rid of something weird. * [NOD-1130] Rename minerClient back to client. * [NOD-1130] Add a few fields to GetConnectedPeerInfoResult. * [NOD-1130] Rename oneTry to isPermanent. * [NOD-1130] Implement ConnectionCount in NetAdapter. * [NOD-1130] Move RawMempoolVerbose out of mempool. * [NOD-1130] Move isSynced into the mining package. * [NOD-1130] Fix a compilation error. * [NOD-1130] Make golint happy. * [NOD-1130] Fix merge errors.
139 lines
4.8 KiB
Go
139 lines
4.8 KiB
Go
// Copyright (c) 2014-2017 The btcsuite developers
|
|
// Copyright (c) 2015-2017 The Decred developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// NOTE: This file is intended to house the RPC websocket notifications that are
|
|
// supported by a kaspa rpc server.
|
|
|
|
package model
|
|
|
|
const (
|
|
// FilteredBlockAddedNtfnMethod is the new method used for
|
|
// notifications from the kaspa rpc server that a block has been connected.
|
|
FilteredBlockAddedNtfnMethod = "filteredBlockAdded"
|
|
|
|
// TxAcceptedNtfnMethod is the method used for notifications from the
|
|
// kaspa rpc server that a transaction has been accepted into the mempool.
|
|
TxAcceptedNtfnMethod = "txAccepted"
|
|
|
|
// TxAcceptedVerboseNtfnMethod is the method used for notifications from
|
|
// the kaspa rpc server that a transaction has been accepted into the
|
|
// mempool. This differs from TxAcceptedNtfnMethod in that it provides
|
|
// more details in the notification.
|
|
TxAcceptedVerboseNtfnMethod = "txAcceptedVerbose"
|
|
|
|
// RelevantTxAcceptedNtfnMethod is the new method used for notifications
|
|
// from the kaspa rpc server that inform a client that a transaction that
|
|
// matches the loaded filter was accepted by the mempool.
|
|
RelevantTxAcceptedNtfnMethod = "relevantTxAccepted"
|
|
|
|
// ChainChangedNtfnMethod is the new method used for notifications
|
|
// from the kaspa rpc server that inform a client that the selected chain
|
|
// has changed.
|
|
ChainChangedNtfnMethod = "chainChanged"
|
|
)
|
|
|
|
// FilteredBlockAddedNtfn defines the filteredBlockAdded JSON-RPC
|
|
// notification.
|
|
type FilteredBlockAddedNtfn struct {
|
|
BlueScore uint64
|
|
Header string
|
|
SubscribedTxs []string
|
|
}
|
|
|
|
// NewFilteredBlockAddedNtfn returns a new instance which can be used to
|
|
// issue a filteredBlockAdded JSON-RPC notification.
|
|
func NewFilteredBlockAddedNtfn(blueScore uint64, header string, subscribedTxs []string) *FilteredBlockAddedNtfn {
|
|
return &FilteredBlockAddedNtfn{
|
|
BlueScore: blueScore,
|
|
Header: header,
|
|
SubscribedTxs: subscribedTxs,
|
|
}
|
|
}
|
|
|
|
// ChainChangedNtfn defines the chainChanged JSON-RPC
|
|
// notification.
|
|
type ChainChangedNtfn struct {
|
|
ChainChangedRawParam ChainChangedRawParam
|
|
}
|
|
|
|
// ChainChangedRawParam is the first parameter
|
|
// of ChainChangedNtfn which contains all the
|
|
// remove chain block hashes and the added
|
|
// chain blocks.
|
|
type ChainChangedRawParam struct {
|
|
RemovedChainBlockHashes []string `json:"removedChainBlockHashes"`
|
|
AddedChainBlocks []ChainBlock `json:"addedChainBlocks"`
|
|
}
|
|
|
|
// NewChainChangedNtfn returns a new instance which can be used to
|
|
// issue a chainChanged JSON-RPC notification.
|
|
func NewChainChangedNtfn(removedChainBlockHashes []string,
|
|
addedChainBlocks []ChainBlock) *ChainChangedNtfn {
|
|
return &ChainChangedNtfn{ChainChangedRawParam: ChainChangedRawParam{
|
|
RemovedChainBlockHashes: removedChainBlockHashes,
|
|
AddedChainBlocks: addedChainBlocks,
|
|
}}
|
|
}
|
|
|
|
// BlockDetails describes details of a tx in a block.
|
|
type BlockDetails struct {
|
|
Height uint64 `json:"height"`
|
|
Hash string `json:"hash"`
|
|
Index int `json:"index"`
|
|
Time int64 `json:"time"`
|
|
}
|
|
|
|
// TxAcceptedNtfn defines the txAccepted JSON-RPC notification.
|
|
type TxAcceptedNtfn struct {
|
|
TxID string
|
|
Amount float64
|
|
}
|
|
|
|
// NewTxAcceptedNtfn returns a new instance which can be used to issue a
|
|
// txAccepted JSON-RPC notification.
|
|
func NewTxAcceptedNtfn(txHash string, amount float64) *TxAcceptedNtfn {
|
|
return &TxAcceptedNtfn{
|
|
TxID: txHash,
|
|
Amount: amount,
|
|
}
|
|
}
|
|
|
|
// TxAcceptedVerboseNtfn defines the txAcceptedVerbose JSON-RPC notification.
|
|
type TxAcceptedVerboseNtfn struct {
|
|
RawTx TxRawResult
|
|
}
|
|
|
|
// NewTxAcceptedVerboseNtfn returns a new instance which can be used to issue a
|
|
// txAcceptedVerbose JSON-RPC notification.
|
|
func NewTxAcceptedVerboseNtfn(rawTx TxRawResult) *TxAcceptedVerboseNtfn {
|
|
return &TxAcceptedVerboseNtfn{
|
|
RawTx: rawTx,
|
|
}
|
|
}
|
|
|
|
// RelevantTxAcceptedNtfn defines the parameters to the relevantTxAccepted
|
|
// JSON-RPC notification.
|
|
type RelevantTxAcceptedNtfn struct {
|
|
Transaction string `json:"transaction"`
|
|
}
|
|
|
|
// NewRelevantTxAcceptedNtfn returns a new instance which can be used to issue a
|
|
// relevantxaccepted JSON-RPC notification.
|
|
func NewRelevantTxAcceptedNtfn(txHex string) *RelevantTxAcceptedNtfn {
|
|
return &RelevantTxAcceptedNtfn{Transaction: txHex}
|
|
}
|
|
|
|
func init() {
|
|
// The commands in this file are only usable by websockets and are
|
|
// notifications.
|
|
flags := UFWebsocketOnly | UFNotification
|
|
|
|
MustRegisterCommand(FilteredBlockAddedNtfnMethod, (*FilteredBlockAddedNtfn)(nil), flags)
|
|
MustRegisterCommand(TxAcceptedNtfnMethod, (*TxAcceptedNtfn)(nil), flags)
|
|
MustRegisterCommand(TxAcceptedVerboseNtfnMethod, (*TxAcceptedVerboseNtfn)(nil), flags)
|
|
MustRegisterCommand(RelevantTxAcceptedNtfnMethod, (*RelevantTxAcceptedNtfn)(nil), flags)
|
|
MustRegisterCommand(ChainChangedNtfnMethod, (*ChainChangedNtfn)(nil), flags)
|
|
}
|