mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-01 18:32:32 +00:00
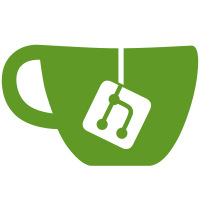
* [NOD-1566] Add a dependency to golang-lru. * [NOD-1566] Add caching to blockstore.go. * [NOD-1566] Add LRUCache to all store objects and initialize them. * [NOD-1566] Add caching to acceptanceDataStore. * [NOD-1566] Add caching to blockHeaderStore. * [NOD-1566] Implement a simpler LRU cache. * [NOD-1566] Use the simpler cache implementation everywhere. * [NOD-1566] Remove dependency in golang-lru. * [NOD-1566] Fix object reuse issues in store Get functions. * [NOD-1566] Add caching to blockRelationStore. * [NOD-1566] Add caching to blockStatusStore. * [NOD-1566] Add caching to ghostdagDataStore. * [NOD-1566] Add caching to multisetStore. * [NOD-1566] Add caching to reachabilityDataStore. * [NOD-1566] Add caching to utxoDiffStore. * [NOD-1566] Add caching to reachabilityReindexRoot. * [NOD-1566] Add caching to pruningStore. * [NOD-1566] Add caching to headerTipsStore. * [NOD-1566] Add caching to consensusStateStore. * [NOD-1566] Add comments explaining why we don't discard staging at the normal location in consensusStateStore. * [NOD-1566] Make go vet happy. * [NOD-1566] Fix merge errors. * [NOD-1566] Add a missing break statement. * [NOD-1566] Run go mod tidy. * [NOD-1566] Remove serializedUTXOSetCache.
60 lines
1.3 KiB
Go
60 lines
1.3 KiB
Go
package lrucache
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// LRUCache is a least-recently-used cache for any type
|
|
// that's able to be indexed by DomainHash
|
|
type LRUCache struct {
|
|
cache map[externalapi.DomainHash]interface{}
|
|
capacity int
|
|
}
|
|
|
|
// New creates a new LRUCache
|
|
func New(capacity int) *LRUCache {
|
|
return &LRUCache{
|
|
cache: make(map[externalapi.DomainHash]interface{}, capacity+1),
|
|
capacity: capacity,
|
|
}
|
|
}
|
|
|
|
// Add adds an entry to the LRUCache
|
|
func (c *LRUCache) Add(key *externalapi.DomainHash, value interface{}) {
|
|
c.cache[*key] = value
|
|
|
|
if len(c.cache) > c.capacity {
|
|
c.evictRandom()
|
|
}
|
|
}
|
|
|
|
// Get returns the entry for the given key, or (nil, false) otherwise
|
|
func (c *LRUCache) Get(key *externalapi.DomainHash) (interface{}, bool) {
|
|
value, ok := c.cache[*key]
|
|
if !ok {
|
|
return nil, false
|
|
}
|
|
return value, true
|
|
}
|
|
|
|
// Has returns whether the LRUCache contains the given key
|
|
func (c *LRUCache) Has(key *externalapi.DomainHash) bool {
|
|
_, ok := c.cache[*key]
|
|
return ok
|
|
}
|
|
|
|
// Remove removes the entry for the the given key. Does nothing if
|
|
// the entry does not exist
|
|
func (c *LRUCache) Remove(key *externalapi.DomainHash) {
|
|
delete(c.cache, *key)
|
|
}
|
|
|
|
func (c *LRUCache) evictRandom() {
|
|
var keyToEvict externalapi.DomainHash
|
|
for key := range c.cache {
|
|
keyToEvict = key
|
|
break
|
|
}
|
|
c.Remove(&keyToEvict)
|
|
}
|