mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-24 15:56:42 +00:00
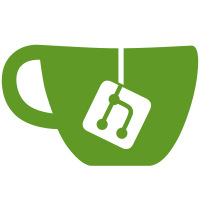
* [NOD-1451] Implement block validator * [NOD-1451] Implement block validator * [NOD-1451] Fix merge errors * [NOD-1451] Implement block validator * [NOD-1451] Implement checkTransactionInIsolation * [NOD-1451] Copy txscript to validator * [NOD-1451] Change txscript to new design * [NOD-1451] Add checkTransactionInContext * [NOD-1451] Add checkBlockSize * [NOD-1451] Add error handling * [NOD-1451] Implement checkTransactionInContext * [NOD-1451] Add checkTransactionMass placeholder * [NOD-1451] Finish validators * [NOD-1451] Add comments and stringers * [NOD-1451] Return model.TransactionValidator interface * [NOD-1451] Premake rule errors for each "code" * [NOD-1451] Populate transaction mass * [NOD-1451] Renmae functions * [NOD-1451] Always use skipPow=false * [NOD-1451] Renames * [NOD-1451] Remove redundant types from WriteElement * [NOD-1451] Fix error message * [NOD-1451] Add checkTransactionPayload * [NOD-1451] Add ValidateProofOfWorkAndDifficulty to block validator interface * [NOD-1451] Move stringers to model * [NOD-1451] Fix error message
56 lines
1.2 KiB
Go
56 lines
1.2 KiB
Go
package externalapi
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"fmt"
|
|
)
|
|
|
|
// DomainTransaction represents a Kaspa transaction
|
|
type DomainTransaction struct {
|
|
Version int32
|
|
Inputs []*DomainTransactionInput
|
|
Outputs []*DomainTransactionOutput
|
|
LockTime uint64
|
|
SubnetworkID DomainSubnetworkID
|
|
Gas uint64
|
|
PayloadHash DomainHash
|
|
Payload []byte
|
|
|
|
Fee uint64
|
|
Mass uint64
|
|
}
|
|
|
|
// DomainTransactionInput represents a Kaspa transaction input
|
|
type DomainTransactionInput struct {
|
|
PreviousOutpoint DomainOutpoint
|
|
SignatureScript []byte
|
|
Sequence uint64
|
|
|
|
UTXOEntry *UTXOEntry
|
|
}
|
|
|
|
// DomainOutpoint represents a Kaspa transaction outpoint
|
|
type DomainOutpoint struct {
|
|
ID DomainTransactionID
|
|
Index uint32
|
|
}
|
|
|
|
// String stringifies an outpoint.
|
|
func (op DomainOutpoint) String() string {
|
|
return fmt.Sprintf("%s:%d", op.ID, op.Index)
|
|
}
|
|
|
|
// DomainTransactionOutput represents a Kaspad transaction output
|
|
type DomainTransactionOutput struct {
|
|
Value uint64
|
|
ScriptPublicKey []byte
|
|
}
|
|
|
|
// DomainTransactionID represents the ID of a Kaspa transaction
|
|
type DomainTransactionID DomainHash
|
|
|
|
// String stringifies a transaction ID.
|
|
func (id *DomainTransactionID) String() string {
|
|
return hex.EncodeToString(id[:])
|
|
}
|