mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-22 23:07:04 +00:00
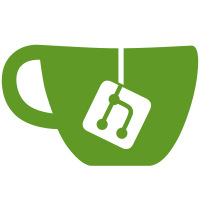
* [NOD-1451] Implement block validator * [NOD-1451] Implement block validator * [NOD-1451] Fix merge errors * [NOD-1451] Implement block validator * [NOD-1451] Implement checkTransactionInIsolation * [NOD-1451] Copy txscript to validator * [NOD-1451] Change txscript to new design * [NOD-1451] Add checkTransactionInContext * [NOD-1451] Add checkBlockSize * [NOD-1451] Add error handling * [NOD-1451] Implement checkTransactionInContext * [NOD-1451] Add checkTransactionMass placeholder * [NOD-1451] Finish validators * [NOD-1451] Add comments and stringers * [NOD-1451] Return model.TransactionValidator interface * [NOD-1451] Premake rule errors for each "code" * [NOD-1451] Populate transaction mass * [NOD-1451] Renmae functions * [NOD-1451] Always use skipPow=false * [NOD-1451] Renames * [NOD-1451] Remove redundant types from WriteElement * [NOD-1451] Fix error message * [NOD-1451] Add checkTransactionPayload * [NOD-1451] Add ValidateProofOfWorkAndDifficulty to block validator interface * [NOD-1451] Move stringers to model * [NOD-1451] Fix error message
104 lines
3.8 KiB
Go
104 lines
3.8 KiB
Go
package dagtopologymanager
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/database"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// dagTopologyManager exposes methods for querying relationships
|
|
// between blocks in the DAG
|
|
type dagTopologyManager struct {
|
|
reachabilityTree model.ReachabilityTree
|
|
blockRelationStore model.BlockRelationStore
|
|
databaseContext *database.DomainDBContext
|
|
}
|
|
|
|
// New instantiates a new DAGTopologyManager
|
|
func New(
|
|
databaseContext *database.DomainDBContext,
|
|
reachabilityTree model.ReachabilityTree,
|
|
blockRelationStore model.BlockRelationStore) model.DAGTopologyManager {
|
|
|
|
return &dagTopologyManager{
|
|
databaseContext: databaseContext,
|
|
reachabilityTree: reachabilityTree,
|
|
blockRelationStore: blockRelationStore,
|
|
}
|
|
}
|
|
|
|
// Parents returns the DAG parents of the given blockHash
|
|
func (dtm *dagTopologyManager) Parents(blockHash *externalapi.DomainHash) ([]*externalapi.DomainHash, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return blockRelations.Parents, nil
|
|
}
|
|
|
|
// Children returns the DAG children of the given blockHash
|
|
func (dtm *dagTopologyManager) Children(blockHash *externalapi.DomainHash) ([]*externalapi.DomainHash, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return blockRelations.Children, nil
|
|
}
|
|
|
|
// IsParentOf returns true if blockHashA is a direct DAG parent of blockHashB
|
|
func (dtm *dagTopologyManager) IsParentOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHashB)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
return isHashInSlice(blockHashA, blockRelations.Parents), nil
|
|
}
|
|
|
|
// IsChildOf returns true if blockHashA is a direct DAG child of blockHashB
|
|
func (dtm *dagTopologyManager) IsChildOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
blockRelations, err := dtm.blockRelationStore.BlockRelation(dtm.databaseContext, blockHashB)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
return isHashInSlice(blockHashA, blockRelations.Children), nil
|
|
}
|
|
|
|
// IsAncestorOf returns true if blockHashA is a DAG ancestor of blockHashB
|
|
func (dtm *dagTopologyManager) IsAncestorOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
return dtm.reachabilityTree.IsDAGAncestorOf(blockHashA, blockHashB)
|
|
}
|
|
|
|
// IsDescendantOf returns true if blockHashA is a DAG descendant of blockHashB
|
|
func (dtm *dagTopologyManager) IsDescendantOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
return dtm.reachabilityTree.IsDAGAncestorOf(blockHashB, blockHashA)
|
|
}
|
|
|
|
// IsAncestorOfAny returns true if `blockHash` is an ancestor of at least one of `potentialDescendants`
|
|
func (dtm *dagTopologyManager) IsAncestorOfAny(blockHash *externalapi.DomainHash, potentialDescendants []*externalapi.DomainHash) (bool, error) {
|
|
panic("unimplemented")
|
|
}
|
|
|
|
// IsInSelectedParentChainOf returns true if blockHashA is in the selected parent chain of blockHashB
|
|
func (dtm *dagTopologyManager) IsInSelectedParentChainOf(blockHashA *externalapi.DomainHash, blockHashB *externalapi.DomainHash) (bool, error) {
|
|
panic("unimplemented")
|
|
}
|
|
|
|
func isHashInSlice(hash *externalapi.DomainHash, hashes []*externalapi.DomainHash) bool {
|
|
for _, h := range hashes {
|
|
if *h == *hash {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
// Tips returns the current DAG tips
|
|
func (dtm *dagTopologyManager) Tips() ([]*externalapi.DomainHash, error) {
|
|
panic("implement me")
|
|
}
|
|
|
|
// AddTip adds the given tip to the current DAG tips
|
|
func (dtm *dagTopologyManager) AddTip(tipHash *externalapi.DomainHash) error {
|
|
panic("implement me")
|
|
}
|