mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 14:22:33 +00:00
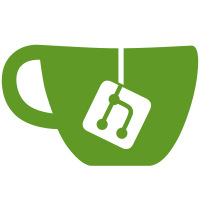
* [NOD-1451] Implement block validator * [NOD-1451] Implement block validator * [NOD-1451] Fix merge errors * [NOD-1451] Implement block validator * [NOD-1451] Implement checkTransactionInIsolation * [NOD-1451] Copy txscript to validator * [NOD-1451] Change txscript to new design * [NOD-1451] Add checkTransactionInContext * [NOD-1451] Add checkBlockSize * [NOD-1451] Add error handling * [NOD-1451] Implement checkTransactionInContext * [NOD-1451] Add checkTransactionMass placeholder * [NOD-1451] Finish validators * [NOD-1451] Add comments and stringers * [NOD-1451] Return model.TransactionValidator interface * [NOD-1451] Premake rule errors for each "code" * [NOD-1451] Populate transaction mass * [NOD-1451] Renmae functions * [NOD-1451] Always use skipPow=false * [NOD-1451] Renames * [NOD-1451] Remove redundant types from WriteElement * [NOD-1451] Fix error message * [NOD-1451] Add checkTransactionPayload * [NOD-1451] Add ValidateProofOfWorkAndDifficulty to block validator interface * [NOD-1451] Move stringers to model * [NOD-1451] Fix error message
43 lines
1.1 KiB
Go
43 lines
1.1 KiB
Go
package hashes
|
|
|
|
import (
|
|
"crypto/sha256"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/pkg/errors"
|
|
"hash"
|
|
)
|
|
|
|
// HashWriter is used to incrementally hash data without concatenating all of the data to a single buffer
|
|
// it exposes an io.Writer api and a Finalize function to get the resulting hash.
|
|
// The used hash function is double-sha256.
|
|
type HashWriter struct {
|
|
inner hash.Hash
|
|
}
|
|
|
|
// NewHashWriter Returns a new HashWriter
|
|
func NewHashWriter() *HashWriter {
|
|
return &HashWriter{sha256.New()}
|
|
}
|
|
|
|
// Write will always return (len(p), nil)
|
|
func (h *HashWriter) Write(p []byte) (n int, err error) {
|
|
return h.inner.Write(p)
|
|
}
|
|
|
|
// Finalize returns the resulting double hash
|
|
func (h *HashWriter) Finalize() externalapi.DomainHash {
|
|
firstHashInTheSum := h.inner.Sum(nil)
|
|
return sha256.Sum256(firstHashInTheSum)
|
|
}
|
|
|
|
// HashData hashes the given byte slice
|
|
func HashData(data []byte) externalapi.DomainHash {
|
|
w := NewHashWriter()
|
|
_, err := w.Write(data)
|
|
if err != nil {
|
|
panic(errors.Wrap(err, "this should never happen. SHA256's digest should never return an error"))
|
|
}
|
|
|
|
return w.Finalize()
|
|
}
|