mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
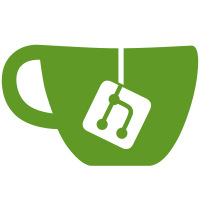
* Copy over the CLI wallet from Kasparov. * Fix trivial compilation errors. * Reimplement the balance command. * Extract isUTXOSpendable to a separate function. * Reimplement the send command. * Fix bad transaction ID parsing. * Add a missing newline in a log. * Don't use msgTx in send(). * Fix isUTXOSpendable not checking whether a UTXO is of a coinbase transaction. * Add --devnet, --testnet, etc. to command line flags. * In `create`, only print the public key of the active network. * Use coinbase maturity in isUTXOSpendable. * Add a readme. * Fix formatting in readme.
86 lines
2.4 KiB
Go
86 lines
2.4 KiB
Go
package main
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/infrastructure/config"
|
|
"github.com/pkg/errors"
|
|
"os"
|
|
|
|
"github.com/jessevdk/go-flags"
|
|
)
|
|
|
|
const (
|
|
createSubCmd = "create"
|
|
balanceSubCmd = "balance"
|
|
sendSubCmd = "send"
|
|
)
|
|
|
|
type createConfig struct {
|
|
config.NetworkFlags
|
|
}
|
|
|
|
type balanceConfig struct {
|
|
RPCServer string `long:"rpcserver" short:"s" description:"RPC server to connect to"`
|
|
Address string `long:"address" short:"d" description:"The public address to check the balance of" required:"true"`
|
|
config.NetworkFlags
|
|
}
|
|
|
|
type sendConfig struct {
|
|
RPCServer string `long:"rpcserver" short:"s" description:"RPC server to connect to"`
|
|
PrivateKey string `long:"private-key" short:"k" description:"The private key of the sender (encoded in hex)" required:"true"`
|
|
ToAddress string `long:"to-address" short:"t" description:"The public address to send Kaspa to" required:"true"`
|
|
SendAmount float64 `long:"send-amount" short:"v" description:"An amount to send in Kaspa (e.g. 1234.12345678)" required:"true"`
|
|
config.NetworkFlags
|
|
}
|
|
|
|
func parseCommandLine() (subCommand string, config interface{}) {
|
|
cfg := &struct{}{}
|
|
parser := flags.NewParser(cfg, flags.PrintErrors|flags.HelpFlag)
|
|
|
|
createConf := &createConfig{}
|
|
parser.AddCommand(createSubCmd, "Creates a new wallet",
|
|
"Creates a private key and 3 public addresses, one for each of MainNet, TestNet and DevNet", createConf)
|
|
|
|
balanceConf := &balanceConfig{}
|
|
parser.AddCommand(balanceSubCmd, "Shows the balance of a public address",
|
|
"Shows the balance for a public address in Kaspa", balanceConf)
|
|
|
|
sendConf := &sendConfig{}
|
|
parser.AddCommand(sendSubCmd, "Sends a Kaspa transaction to a public address",
|
|
"Sends a Kaspa transaction to a public address", sendConf)
|
|
|
|
_, err := parser.Parse()
|
|
|
|
if err != nil {
|
|
var flagsErr *flags.Error
|
|
if ok := errors.As(err, &flagsErr); ok && flagsErr.Type == flags.ErrHelp {
|
|
os.Exit(0)
|
|
} else {
|
|
os.Exit(1)
|
|
}
|
|
return "", nil
|
|
}
|
|
|
|
switch parser.Command.Active.Name {
|
|
case createSubCmd:
|
|
err := createConf.ResolveNetwork(parser)
|
|
if err != nil {
|
|
printErrorAndExit(err)
|
|
}
|
|
config = createConf
|
|
case balanceSubCmd:
|
|
err := balanceConf.ResolveNetwork(parser)
|
|
if err != nil {
|
|
printErrorAndExit(err)
|
|
}
|
|
config = balanceConf
|
|
case sendSubCmd:
|
|
err := sendConf.ResolveNetwork(parser)
|
|
if err != nil {
|
|
printErrorAndExit(err)
|
|
}
|
|
config = sendConf
|
|
}
|
|
|
|
return parser.Command.Active.Name, config
|
|
}
|