mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
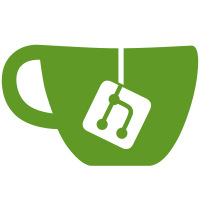
* Replace BlockMessage with RpcBlock in rpc.proto. * Convert everything in kaspad to use RPCBlocks and fix tests. * Fix compilation errors in stability tests and the miner. * Update TransactionVerboseData in rpc.proto. * Update TransactionVerboseData in the rest of kaspad. * Make golint happy. * Include RpcTransactionVerboseData in RpcTransaction instead of the other way around. * Regenerate rpc.pb.go after merge. * Update appmessage types. * Update appmessage request and response types. * Reimplement conversion functions between appmessage.RPCTransaction and protowire.RpcTransaction. * Extract RpcBlockHeader toAppMessage/fromAppMessage out of RpcBlock. * Fix compilation errors in getBlock, getBlocks, and submitBlock. * Fix compilation errors in getMempoolEntry. * Fix compilation errors in notifyBlockAdded. * Update verbosedata.go. * Fix compilation errors in getBlock and getBlocks. * Fix compilation errors in getBlocks tests. * Fix conversions between getBlocks message types. * Fix integration tests. * Fix a comment. * Add selectedParent to the verbose block response.
54 lines
1.6 KiB
Go
54 lines
1.6 KiB
Go
package appmessage
|
|
|
|
// NotifyBlockAddedRequestMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type NotifyBlockAddedRequestMessage struct {
|
|
baseMessage
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *NotifyBlockAddedRequestMessage) Command() MessageCommand {
|
|
return CmdNotifyBlockAddedRequestMessage
|
|
}
|
|
|
|
// NewNotifyBlockAddedRequestMessage returns a instance of the message
|
|
func NewNotifyBlockAddedRequestMessage() *NotifyBlockAddedRequestMessage {
|
|
return &NotifyBlockAddedRequestMessage{}
|
|
}
|
|
|
|
// NotifyBlockAddedResponseMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type NotifyBlockAddedResponseMessage struct {
|
|
baseMessage
|
|
Error *RPCError
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *NotifyBlockAddedResponseMessage) Command() MessageCommand {
|
|
return CmdNotifyBlockAddedResponseMessage
|
|
}
|
|
|
|
// NewNotifyBlockAddedResponseMessage returns a instance of the message
|
|
func NewNotifyBlockAddedResponseMessage() *NotifyBlockAddedResponseMessage {
|
|
return &NotifyBlockAddedResponseMessage{}
|
|
}
|
|
|
|
// BlockAddedNotificationMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type BlockAddedNotificationMessage struct {
|
|
baseMessage
|
|
Block *RPCBlock
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *BlockAddedNotificationMessage) Command() MessageCommand {
|
|
return CmdBlockAddedNotificationMessage
|
|
}
|
|
|
|
// NewBlockAddedNotificationMessage returns a instance of the message
|
|
func NewBlockAddedNotificationMessage(block *RPCBlock) *BlockAddedNotificationMessage {
|
|
return &BlockAddedNotificationMessage{
|
|
Block: block,
|
|
}
|
|
}
|