mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-03 03:12:30 +00:00
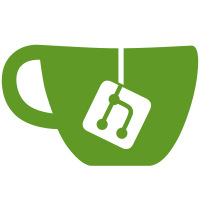
* [DEV-81] Overwrite maxOpenFiles for testInterface to force tests to check the LRU-mechanism in openFile * [DEV-81] Added database.UseLogger test * [DEV-81] Completed coverage of reconcileDB() * [DEV-81] Added some tests for dbcache * [DEV-81] Moved init and UseLogger to separate file to make them more easily-testable + added tests * [DEV-81] Added tests for deleteFile * [DEV-81] Added tests to cursor.Delete + made sure it returns error when transaction is not writable * [DEV-81] Moved database/error_test.go from database_test package to database package + added test for IsErrorCode * [DEV-81] Added tests for handleRollback error-cases * [DEV-81] Added tests for cursor.skipPendingUpdates * [DEV-81] Added tests for various cursor edge-cases * [DEV-81] tx.putKey no longer returns error, because there is no case when it does * [DEV-81] Added tests to CreateBucket error cases * [DEV-81] Added tests to bucket.Get and .Delete error cases + .Delete now returns error on empty key * [DEV-81] Added test for ForEachBucket * [DEV-81] Added tests to StoreBlock * [DEV-81] Added test for deleting a double nested bucket * [DEV-81] Removed log_test, as it is no longer necessary with the logging system re-design * [DEV-81] Added test to some of writePendingAndCommit error-cases * [DEV-81] Update references from btcutil to btcd/util * [DEV-81] Add tests for dbCacheIterator{.Next(), .Prev(), .Key, .Value()} in cases when iterator is exhausted * [DEV-81] Added tests for ldbIterator placeholder functions * [DEV-81] Added test name to Error messsages in TestSkipPendingUpdates * [DEV-81] Begin writing TestSkipPendingUpdatesCache * [DEV-81] Added error-cases for DBCache.flush() and DBCache.commitTreaps() * [DEV-81] Use monkey.patch from bou.ke and not from github * [DEV-81] Rewrote IsErrorCode in both database and txscript packages to be more concise * [DEV-81] Rename any database.Tx to dbTx instead of tx - to remove confusion with coin Tx * [DEV-81] Fix typo * [DEV-81] Use os.TempDir() instead of /tmp/ to be cross-platform * [DEV-81] use SimNet for database tests + Error if testDB exists after deleting it * [DEV-81] Removed useLogger - it's redundant * [DEV-81] Added comment on how CRC32 checksums are calculated in reconcile_test.go * [DEV-81] Added comment that explains what setWriteRow does * [DEV-81] Use constant instead of hard-coded value * [DEV-81] Fixed some typo's + better formatting
119 lines
3.0 KiB
Go
119 lines
3.0 KiB
Go
// Copyright (c) 2015-2016 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package database
|
|
|
|
import (
|
|
"errors"
|
|
"testing"
|
|
)
|
|
|
|
// TestErrorCodeStringer tests the stringized output for the ErrorCode type.
|
|
func TestErrorCodeStringer(t *testing.T) {
|
|
tests := []struct {
|
|
in ErrorCode
|
|
want string
|
|
}{
|
|
{ErrDbTypeRegistered, "ErrDbTypeRegistered"},
|
|
{ErrDbUnknownType, "ErrDbUnknownType"},
|
|
{ErrDbDoesNotExist, "ErrDbDoesNotExist"},
|
|
{ErrDbExists, "ErrDbExists"},
|
|
{ErrDbNotOpen, "ErrDbNotOpen"},
|
|
{ErrDbAlreadyOpen, "ErrDbAlreadyOpen"},
|
|
{ErrInvalid, "ErrInvalid"},
|
|
{ErrCorruption, "ErrCorruption"},
|
|
{ErrTxClosed, "ErrTxClosed"},
|
|
{ErrTxNotWritable, "ErrTxNotWritable"},
|
|
{ErrBucketNotFound, "ErrBucketNotFound"},
|
|
{ErrBucketExists, "ErrBucketExists"},
|
|
{ErrBucketNameRequired, "ErrBucketNameRequired"},
|
|
{ErrKeyRequired, "ErrKeyRequired"},
|
|
{ErrKeyTooLarge, "ErrKeyTooLarge"},
|
|
{ErrValueTooLarge, "ErrValueTooLarge"},
|
|
{ErrIncompatibleValue, "ErrIncompatibleValue"},
|
|
{ErrBlockNotFound, "ErrBlockNotFound"},
|
|
{ErrBlockExists, "ErrBlockExists"},
|
|
{ErrBlockRegionInvalid, "ErrBlockRegionInvalid"},
|
|
{ErrDriverSpecific, "ErrDriverSpecific"},
|
|
|
|
{0xffff, "Unknown ErrorCode (65535)"},
|
|
}
|
|
|
|
// Detect additional error codes that don't have the stringer added.
|
|
if len(tests)-1 != int(TstNumErrorCodes) {
|
|
t.Errorf("It appears an error code was added without adding " +
|
|
"an associated stringer test")
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
result := test.in.String()
|
|
if result != test.want {
|
|
t.Errorf("String #%d\ngot: %s\nwant: %s", i, result,
|
|
test.want)
|
|
continue
|
|
}
|
|
}
|
|
}
|
|
|
|
// TestError tests the error output for the Error type.
|
|
func TestError(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
tests := []struct {
|
|
in Error
|
|
want string
|
|
}{
|
|
{
|
|
Error{Description: "some error"},
|
|
"some error",
|
|
},
|
|
{
|
|
Error{Description: "human-readable error"},
|
|
"human-readable error",
|
|
},
|
|
{
|
|
Error{
|
|
ErrorCode: ErrDriverSpecific,
|
|
Description: "some error",
|
|
Err: errors.New("driver-specific error"),
|
|
},
|
|
"some error: driver-specific error",
|
|
},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
result := test.in.Error()
|
|
if result != test.want {
|
|
t.Errorf("Error #%d\n got: %s want: %s", i, result,
|
|
test.want)
|
|
continue
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestIsErrorCode(t *testing.T) {
|
|
dummyError := errors.New("")
|
|
|
|
tests := []struct {
|
|
err error
|
|
code ErrorCode
|
|
expectedResult bool
|
|
}{
|
|
{makeError(ErrBucketExists, "", dummyError), ErrBucketExists, true},
|
|
{makeError(ErrBucketExists, "", dummyError), ErrBlockExists, false},
|
|
{dummyError, ErrBlockExists, false},
|
|
{nil, ErrBlockExists, false},
|
|
}
|
|
|
|
for i, test := range tests {
|
|
actualResult := IsErrorCode(test.err, test.code)
|
|
if test.expectedResult != actualResult {
|
|
t.Errorf("TestIsErrorCode: %d: Expected: %t, but got: %t",
|
|
i, test.expectedResult, actualResult)
|
|
}
|
|
}
|
|
}
|