mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 07:16:47 +00:00
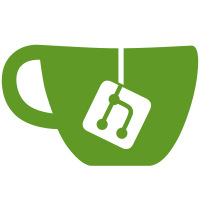
* [NOD-1420] Start working on ConsensusStateManager. Might be redundant due to recent changes * [NOD-1420] Convert model to externalapi in utxo_algerbra helpers * [NOD-1420] Add UTXO-diff algebra * [NOD-1420] Prepare skeleton of calculateAcceptanceDataAndMultiset * [NOD-1420] Added skeleton for AddBlockToVirtual * [NOD-1420] Implement PopulateTransactionWithUTXOEntries * [NOD-1420] Implement restorePastUTXO * [NOD-1420] Implement finality check * [NOD-1420] Move handling of tips to consensusStateManager * [NOD-1420] Implement calculateAcceptanceDataAndMultiset * [NOD-1420] Start implementing resolveBlockStatus * [NOD-1420] Implement resolveBlockStatus * [NOD-1420] Update related fields in end of resolveSingleBlockStatus * [NOD-1420] Start working on selectVirtualParents * [NOD-1420] Implemented BlockHeap * [NOD-1420] Implement selectVirtualParents * [NOD-1420] Implement updateVirtual * [NOD-1420] Added comments where they were missing * [NOD-1420] Place all consensusStateManager functions in correct files * [NOD-1420] Return the missing outpoints from populateTransactionWithUTXOEntriesFromVirtualOrDiff * [NOD-1420] Outpoint.ID -> TransactionID * [NOD-1420] Fix Stringer tests * [NOD-1420] Copy hash.FromString into utils * [NOD-1420] SetParents should return an error * [NOD-1420] Remove all reachabilityManager references from consensusStateManager * [NOD-1420] Remove VirtualData. Get the info from the stores where needed * [NOD-1420] Invert parameters to IsAncestorOf * [NOD-1420] Use model.AcceptanceData * [NOD-1420] Don't return accumulatedMassBefore in error cases * [NOD-1420] Don't expect store functions to return nil when the requested data was found - instead add HasXXX functions * [NOD-1420] addTransactionToMultiset sets isCoinbase properly * [NOD-1420] expected hash string length is externalapi.DomainHashSize * 2 * [NOD-1420] Rename reachabilityTree -> reachabilityManager + updateReindexRoot if isNextVirtualSelectedParent * [NOD-1420] ValidateCoinbaseTransaction in csm.verifyAndBuildUTXO * [NOD-1420] Re-write HAsUTXODiffChild * [NOD-1420] delete past_utxo.go.bak * [NOD-1420] Implement validateCoinbaseTransaction in CSM * [NOD-1420] Imlemented missing functionality in ValidateTransactionAndPopulateWithConsensusData * [NOD-1420] Moved merge depth logic to MergeDepthManager * [NOD-1420] Add logs
96 lines
3.0 KiB
Go
96 lines
3.0 KiB
Go
package dagtraversalmanager
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// dagTraversalManager exposes methods for travering blocks
|
|
// in the DAG
|
|
type dagTraversalManager struct {
|
|
databaseContext model.DBReader
|
|
|
|
dagTopologyManager model.DAGTopologyManager
|
|
ghostdagDataStore model.GHOSTDAGDataStore
|
|
ghostdagManager model.GHOSTDAGManager
|
|
}
|
|
|
|
// selectedParentIterator implements the `model.SelectedParentIterator` API
|
|
type selectedParentIterator struct {
|
|
databaseContext model.DBReader
|
|
ghostdagDataStore model.GHOSTDAGDataStore
|
|
current *externalapi.DomainHash
|
|
}
|
|
|
|
func (spi *selectedParentIterator) Next() bool {
|
|
if spi.current == nil {
|
|
return false
|
|
}
|
|
ghostdagData, err := spi.ghostdagDataStore.Get(spi.databaseContext, spi.current)
|
|
if err != nil {
|
|
panic(fmt.Sprintf("ghostdagDataStore is missing ghostdagData for: %v. '%s' ", spi.current, err))
|
|
}
|
|
spi.current = ghostdagData.SelectedParent
|
|
return spi.current != nil
|
|
}
|
|
|
|
func (spi *selectedParentIterator) Get() *externalapi.DomainHash {
|
|
return spi.current
|
|
}
|
|
|
|
// New instantiates a new DAGTraversalManager
|
|
func New(
|
|
databaseContext model.DBReader,
|
|
dagTopologyManager model.DAGTopologyManager,
|
|
ghostdagDataStore model.GHOSTDAGDataStore,
|
|
ghostdagManager model.GHOSTDAGManager) model.DAGTraversalManager {
|
|
return &dagTraversalManager{
|
|
databaseContext: databaseContext,
|
|
dagTopologyManager: dagTopologyManager,
|
|
ghostdagDataStore: ghostdagDataStore,
|
|
ghostdagManager: ghostdagManager,
|
|
}
|
|
}
|
|
|
|
// SelectedParentIterator creates an iterator over the selected
|
|
// parent chain of the given highHash
|
|
func (dtm *dagTraversalManager) SelectedParentIterator(highHash *externalapi.DomainHash) model.SelectedParentIterator {
|
|
return &selectedParentIterator{
|
|
databaseContext: dtm.databaseContext,
|
|
ghostdagDataStore: dtm.ghostdagDataStore,
|
|
current: highHash,
|
|
}
|
|
}
|
|
|
|
// HighestChainBlockBelowBlueScore returns the hash of the
|
|
// highest block with a blue score lower than the given
|
|
// blueScore in the block with the given highHash's selected
|
|
// parent chain
|
|
func (dtm *dagTraversalManager) HighestChainBlockBelowBlueScore(highHash *externalapi.DomainHash, blueScore uint64) (*externalapi.DomainHash, error) {
|
|
|
|
blockHash := highHash
|
|
chainBlock, err := dtm.ghostdagDataStore.Get(dtm.databaseContext, highHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if chainBlock.BlueScore < blueScore { // will practically return genesis.
|
|
blueScore = chainBlock.BlueScore
|
|
}
|
|
|
|
requiredBlueScore := chainBlock.BlueScore - blueScore
|
|
|
|
// If we used `SelectedParentIterator` we'd need to do more calls to `ghostdagDataStore` so we can get the blueScore
|
|
for chainBlock.BlueScore >= requiredBlueScore {
|
|
if chainBlock.SelectedParent == nil { // genesis
|
|
return blockHash, nil
|
|
}
|
|
blockHash = chainBlock.SelectedParent
|
|
chainBlock, err = dtm.ghostdagDataStore.Get(dtm.databaseContext, highHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
return blockHash, nil
|
|
}
|