mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
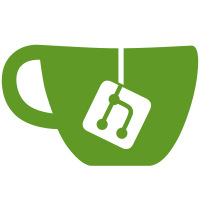
* [NOD-1223] Delete unused files/packages. * [NOD-1223] Move signal and limits to the os package. * [NOD-1223] Put database and dbaccess into the db package. * [NOD-1223] Fold the logs package into the logger package. * [NOD-1223] Rename domainmessage to appmessage. * [NOD-1223] Rename to/from DomainMessage to AppMessage. * [NOD-1223] Move appmessage to the app packge. * [NOD-1223] Move protocol to the app packge. * [NOD-1223] Move the network package to the infrastructure packge. * [NOD-1223] Rename cmd to executables. * [NOD-1223] Fix go.doc in the logger package.
115 lines
2.9 KiB
Go
115 lines
2.9 KiB
Go
package grpcserver
|
|
|
|
import (
|
|
"github.com/pkg/errors"
|
|
"net"
|
|
"sync/atomic"
|
|
"time"
|
|
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/router"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/server/grpcserver/protowire"
|
|
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/server"
|
|
"google.golang.org/grpc"
|
|
)
|
|
|
|
type gRPCConnection struct {
|
|
server *gRPCServer
|
|
address *net.TCPAddr
|
|
isOutbound bool
|
|
stream grpcStream
|
|
router *router.Router
|
|
|
|
stopChan chan struct{}
|
|
clientConn grpc.ClientConn
|
|
onDisconnectedHandler server.OnDisconnectedHandler
|
|
onInvalidMessageHandler server.OnInvalidMessageHandler
|
|
|
|
isConnected uint32
|
|
}
|
|
|
|
func newConnection(server *gRPCServer, address *net.TCPAddr, isOutbound bool, stream grpcStream) *gRPCConnection {
|
|
connection := &gRPCConnection{
|
|
server: server,
|
|
address: address,
|
|
isOutbound: isOutbound,
|
|
stream: stream,
|
|
stopChan: make(chan struct{}),
|
|
isConnected: 1,
|
|
}
|
|
|
|
return connection
|
|
}
|
|
|
|
func (c *gRPCConnection) Start(router *router.Router) {
|
|
if c.onDisconnectedHandler == nil {
|
|
panic(errors.New("onDisconnectedHandler is nil"))
|
|
}
|
|
|
|
if c.onInvalidMessageHandler == nil {
|
|
panic(errors.New("onInvalidMessageHandler is nil"))
|
|
}
|
|
|
|
c.router = router
|
|
|
|
spawn("gRPCConnection.Start-connectionLoops", func() {
|
|
err := c.connectionLoops()
|
|
if err != nil {
|
|
log.Errorf("error from connectionLoops for %s: %+v", c.address, err)
|
|
}
|
|
})
|
|
}
|
|
|
|
func (c *gRPCConnection) String() string {
|
|
return c.Address().String()
|
|
}
|
|
|
|
func (c *gRPCConnection) IsConnected() bool {
|
|
return atomic.LoadUint32(&c.isConnected) != 0
|
|
}
|
|
|
|
func (c *gRPCConnection) SetOnDisconnectedHandler(onDisconnectedHandler server.OnDisconnectedHandler) {
|
|
c.onDisconnectedHandler = onDisconnectedHandler
|
|
}
|
|
|
|
func (c *gRPCConnection) SetOnInvalidMessageHandler(onInvalidMessageHandler server.OnInvalidMessageHandler) {
|
|
c.onInvalidMessageHandler = onInvalidMessageHandler
|
|
}
|
|
|
|
func (c *gRPCConnection) IsOutbound() bool {
|
|
return c.isOutbound
|
|
}
|
|
|
|
// Disconnect disconnects the connection
|
|
// Calling this function a second time doesn't do anything
|
|
//
|
|
// This is part of the Connection interface
|
|
func (c *gRPCConnection) Disconnect() {
|
|
if !c.IsConnected() {
|
|
return
|
|
}
|
|
atomic.StoreUint32(&c.isConnected, 0)
|
|
|
|
close(c.stopChan)
|
|
|
|
if c.isOutbound {
|
|
spawn("gRPCConnection.Disconnect-clientStream.CloseSend", func() {
|
|
// Wait a second before closing the stream, to let the send queue to get emptied.
|
|
const finishSendDuration = time.Second
|
|
time.Sleep(finishSendDuration)
|
|
clientStream := c.stream.(protowire.P2P_MessageStreamClient)
|
|
_ = clientStream.CloseSend() // ignore error because we don't really know what's the status of the connection
|
|
log.Debugf("Disconnected from %s", c)
|
|
})
|
|
}
|
|
|
|
log.Debugf("Disconnecting from %s", c)
|
|
if c.onDisconnectedHandler != nil {
|
|
c.onDisconnectedHandler()
|
|
}
|
|
}
|
|
|
|
func (c *gRPCConnection) Address() *net.TCPAddr {
|
|
return c.address
|
|
}
|