mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
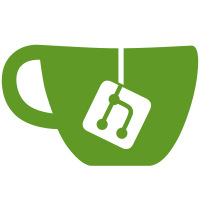
* [NOD-1223] Delete unused files/packages. * [NOD-1223] Move signal and limits to the os package. * [NOD-1223] Put database and dbaccess into the db package. * [NOD-1223] Fold the logs package into the logger package. * [NOD-1223] Rename domainmessage to appmessage. * [NOD-1223] Rename to/from DomainMessage to AppMessage. * [NOD-1223] Move appmessage to the app packge. * [NOD-1223] Move protocol to the app packge. * [NOD-1223] Move the network package to the infrastructure packge. * [NOD-1223] Rename cmd to executables. * [NOD-1223] Fix go.doc in the logger package.
109 lines
2.5 KiB
Go
109 lines
2.5 KiB
Go
package protowire
|
|
|
|
import (
|
|
"math"
|
|
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
"github.com/kaspanet/kaspad/util/mstime"
|
|
"github.com/kaspanet/kaspad/util/subnetworkid"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
func (x *Hash) toWire() (*daghash.Hash, error) {
|
|
return daghash.NewHash(x.Bytes)
|
|
}
|
|
|
|
func protoHashesToWire(protoHashes []*Hash) ([]*daghash.Hash, error) {
|
|
hashes := make([]*daghash.Hash, len(protoHashes))
|
|
for i, protoHash := range protoHashes {
|
|
var err error
|
|
hashes[i], err = protoHash.toWire()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
return hashes, nil
|
|
}
|
|
|
|
func wireHashToProto(hash *daghash.Hash) *Hash {
|
|
return &Hash{
|
|
Bytes: hash.CloneBytes(),
|
|
}
|
|
}
|
|
|
|
func wireHashesToProto(hashes []*daghash.Hash) []*Hash {
|
|
protoHashes := make([]*Hash, len(hashes))
|
|
for i, hash := range hashes {
|
|
protoHashes[i] = wireHashToProto(hash)
|
|
}
|
|
return protoHashes
|
|
}
|
|
|
|
func (x *TransactionID) toWire() (*daghash.TxID, error) {
|
|
return daghash.NewTxID(x.Bytes)
|
|
}
|
|
|
|
func protoTransactionIDsToWire(protoIDs []*TransactionID) ([]*daghash.TxID, error) {
|
|
txIDs := make([]*daghash.TxID, len(protoIDs))
|
|
for i, protoID := range protoIDs {
|
|
var err error
|
|
txIDs[i], err = protoID.toWire()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
return txIDs, nil
|
|
}
|
|
|
|
func wireTransactionIDToProto(id *daghash.TxID) *TransactionID {
|
|
return &TransactionID{
|
|
Bytes: id.CloneBytes(),
|
|
}
|
|
}
|
|
|
|
func wireTransactionIDsToProto(ids []*daghash.TxID) []*TransactionID {
|
|
protoIDs := make([]*TransactionID, len(ids))
|
|
for i, hash := range ids {
|
|
protoIDs[i] = wireTransactionIDToProto(hash)
|
|
}
|
|
return protoIDs
|
|
}
|
|
|
|
func (x *SubnetworkID) toWire() (*subnetworkid.SubnetworkID, error) {
|
|
if x == nil {
|
|
return nil, nil
|
|
}
|
|
return subnetworkid.New(x.Bytes)
|
|
}
|
|
|
|
func wireSubnetworkIDToProto(id *subnetworkid.SubnetworkID) *SubnetworkID {
|
|
if id == nil {
|
|
return nil
|
|
}
|
|
return &SubnetworkID{
|
|
Bytes: id.CloneBytes(),
|
|
}
|
|
}
|
|
|
|
func (x *NetAddress) toWire() (*appmessage.NetAddress, error) {
|
|
if x.Port > math.MaxUint16 {
|
|
return nil, errors.Errorf("port number is larger than %d", math.MaxUint16)
|
|
}
|
|
return &appmessage.NetAddress{
|
|
Timestamp: mstime.UnixMilliseconds(x.Timestamp),
|
|
Services: appmessage.ServiceFlag(x.Services),
|
|
IP: x.Ip,
|
|
Port: uint16(x.Port),
|
|
}, nil
|
|
}
|
|
|
|
func wireNetAddressToProto(address *appmessage.NetAddress) *NetAddress {
|
|
return &NetAddress{
|
|
Timestamp: address.Timestamp.UnixMilliseconds(),
|
|
Services: uint64(address.Services),
|
|
Ip: address.IP,
|
|
Port: uint32(address.Port),
|
|
}
|
|
}
|