mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-03 19:32:30 +00:00
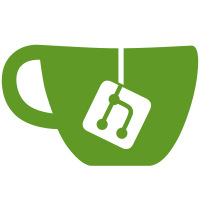
* [NOD-201] Implemented the AddSubnetwork CLI tool. * [NOD-201] Fixed various bugs in AddSubnetwork. * [NOD-201] Fixed mempool maybeAcceptTransaction verifying gasLimit for a subnetwork registry transaction. * [NOD-201] Fixed serialization/deserialization bugs in addrIndex. * [NOD-201] Fixed BlockConfirmationsByHash not handling the zeroHash. * [NOD-201] Used btclog instead of go log. * [NOD-201] Made gasLimit a command-line flag. Made waitForSubnetworkToBecomeAccepted only return an error. * [NOD-201] Filtered out mempool transactions. * [NOD-201] Fixed embarrassing typos. * [NOD-201] Added subnetwork registry tx fee + appropriate cli flag. * [NOD-201] Skipped TXOs that can't pay for registration.
38 lines
734 B
Go
38 lines
734 B
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/daglabs/btcd/rpcclient"
|
|
"io/ioutil"
|
|
)
|
|
|
|
func connect(cfg *config) (*rpcclient.Client, error) {
|
|
var cert []byte
|
|
if !cfg.DisableTLS {
|
|
var err error
|
|
cert, err = ioutil.ReadFile(cfg.RPCCert)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("error reading certificates file: %s", err)
|
|
}
|
|
}
|
|
|
|
connCfg := &rpcclient.ConnConfig{
|
|
Host: cfg.RPCServer,
|
|
Endpoint: "ws",
|
|
User: cfg.RPCUser,
|
|
Pass: cfg.RPCPassword,
|
|
DisableTLS: cfg.DisableTLS,
|
|
}
|
|
|
|
if !cfg.DisableTLS {
|
|
connCfg.Certificates = cert
|
|
}
|
|
|
|
client, err := rpcclient.New(connCfg, nil)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("error connecting to address %s: %s", cfg.RPCServer, err)
|
|
}
|
|
|
|
return client, nil
|
|
}
|