mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-08 22:02:34 +00:00
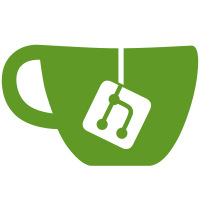
* [NOD-196] move coinbase scriptpukey to payload (no tests) (#311) * [NOD-196] Move coinbase scriptPubKey to payload * [NOD-196] Rename SubnetworkID.IsFull to SubnetworkID.IsBuiltIn * [NOD-196] Fix comments * [NOD-196] Add block subsidy to fee transaction * [NOD-196] Fix comments * [NOD-217] Merge coinbase and fee transaction (#328) * [NOD-196] Fix tests * [NOD-196] Fix tests * [NOD-217] Add error to getBluesFeeData * [NOD-217] Merge Coinbase and fee transaction * [NOD-217] Format project * [NOD-217] Remove OpTrue default for mining.NewBlockTemplate * [NOD-196] Format project * [NOD-217] Add missing space before comment * [NOD-196] Change MaxCoinbasePayloadLen to 150
155 lines
6.4 KiB
Go
155 lines
6.4 KiB
Go
// Copyright (c) 2014-2016 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package dagconfig
|
|
|
|
import (
|
|
"bytes"
|
|
"testing"
|
|
|
|
"github.com/davecgh/go-spew/spew"
|
|
)
|
|
|
|
// TestGenesisBlock tests the genesis block of the main network for validity by
|
|
// checking the encoded bytes and hashes.
|
|
func TestGenesisBlock(t *testing.T) {
|
|
// Encode the genesis block to raw bytes.
|
|
var buf bytes.Buffer
|
|
err := MainNetParams.GenesisBlock.Serialize(&buf)
|
|
if err != nil {
|
|
t.Fatalf("TestGenesisBlock: %v", err)
|
|
}
|
|
|
|
// Ensure the encoded block matches the expected bytes.
|
|
if !bytes.Equal(buf.Bytes(), genesisBlockBytes) {
|
|
t.Fatalf("TestGenesisBlock: Genesis block does not appear valid - "+
|
|
"got %v, want %v", spew.Sdump(buf.Bytes()),
|
|
spew.Sdump(genesisBlockBytes))
|
|
}
|
|
|
|
// Check hash of the block against expected hash.
|
|
hash := MainNetParams.GenesisBlock.BlockHash()
|
|
if !MainNetParams.GenesisHash.IsEqual(hash) {
|
|
t.Fatalf("TestGenesisBlock: Genesis block hash does not "+
|
|
"appear valid - got %v, want %v", spew.Sdump(hash),
|
|
spew.Sdump(MainNetParams.GenesisHash))
|
|
}
|
|
}
|
|
|
|
// TestRegTestGenesisBlock tests the genesis block of the regression test
|
|
// network for validity by checking the encoded bytes and hashes.
|
|
func TestRegTestGenesisBlock(t *testing.T) {
|
|
// Encode the genesis block to raw bytes.
|
|
var buf bytes.Buffer
|
|
err := RegressionNetParams.GenesisBlock.Serialize(&buf)
|
|
if err != nil {
|
|
t.Fatalf("TestRegTestGenesisBlock: %v", err)
|
|
}
|
|
|
|
// Ensure the encoded block matches the expected bytes.
|
|
if !bytes.Equal(buf.Bytes(), regTestGenesisBlockBytes) {
|
|
t.Fatalf("TestRegTestGenesisBlock: Genesis block does not "+
|
|
"appear valid - got %v, want %v",
|
|
spew.Sdump(buf.Bytes()),
|
|
spew.Sdump(regTestGenesisBlockBytes))
|
|
}
|
|
|
|
// Check hash of the block against expected hash.
|
|
hash := RegressionNetParams.GenesisBlock.BlockHash()
|
|
if !RegressionNetParams.GenesisHash.IsEqual(hash) {
|
|
t.Fatalf("TestRegTestGenesisBlock: Genesis block hash does "+
|
|
"not appear valid - got %v, want %v", spew.Sdump(hash),
|
|
spew.Sdump(RegressionNetParams.GenesisHash))
|
|
}
|
|
}
|
|
|
|
// TestTestNet3GenesisBlock tests the genesis block of the test network (version
|
|
// 3) for validity by checking the encoded bytes and hashes.
|
|
func TestTestNet3GenesisBlock(t *testing.T) {
|
|
// Encode the genesis block to raw bytes.
|
|
var buf bytes.Buffer
|
|
err := TestNet3Params.GenesisBlock.Serialize(&buf)
|
|
if err != nil {
|
|
t.Fatalf("TestTestNet3GenesisBlock: %v", err)
|
|
}
|
|
|
|
// Ensure the encoded block matches the expected bytes.
|
|
if !bytes.Equal(buf.Bytes(), testNet3GenesisBlockBytes) {
|
|
t.Fatalf("TestTestNet3GenesisBlock: Genesis block does not "+
|
|
"appear valid - got %v, want %v",
|
|
spew.Sdump(buf.Bytes()),
|
|
spew.Sdump(testNet3GenesisBlockBytes))
|
|
}
|
|
|
|
// Check hash of the block against expected hash.
|
|
hash := TestNet3Params.GenesisBlock.BlockHash()
|
|
if !TestNet3Params.GenesisHash.IsEqual(hash) {
|
|
t.Fatalf("TestTestNet3GenesisBlock: Genesis block hash does "+
|
|
"not appear valid - got %v, want %v", spew.Sdump(hash),
|
|
spew.Sdump(TestNet3Params.GenesisHash))
|
|
}
|
|
}
|
|
|
|
// TestSimNetGenesisBlock tests the genesis block of the simulation test network
|
|
// for validity by checking the encoded bytes and hashes.
|
|
func TestSimNetGenesisBlock(t *testing.T) {
|
|
// Encode the genesis block to raw bytes.
|
|
var buf bytes.Buffer
|
|
err := SimNetParams.GenesisBlock.Serialize(&buf)
|
|
if err != nil {
|
|
t.Fatalf("TestSimNetGenesisBlock: %v", err)
|
|
}
|
|
|
|
// Ensure the encoded block matches the expected bytes.
|
|
if !bytes.Equal(buf.Bytes(), simNetGenesisBlockBytes) {
|
|
t.Fatalf("TestSimNetGenesisBlock: Genesis block does not "+
|
|
"appear valid - got %v, want %v",
|
|
spew.Sdump(buf.Bytes()),
|
|
spew.Sdump(simNetGenesisBlockBytes))
|
|
}
|
|
|
|
// Check hash of the block against expected hash.
|
|
hash := SimNetParams.GenesisBlock.BlockHash()
|
|
if !SimNetParams.GenesisHash.IsEqual(hash) {
|
|
t.Fatalf("TestSimNetGenesisBlock: Genesis block hash does "+
|
|
"not appear valid - got %v, want %v", spew.Sdump(hash),
|
|
spew.Sdump(SimNetParams.GenesisHash))
|
|
}
|
|
}
|
|
|
|
// genesisBlockBytes are the wire encoded bytes for the genesis block of the
|
|
// main network as of protocol version 60002.
|
|
var genesisBlockBytes = []byte{
|
|
0x01, 0x00, 0x00, 0x00, 0x00, 0x72, 0x10, 0x35, 0x85, 0xdd, 0xac, 0x82, 0x5c, 0x49, 0x13, 0x9f,
|
|
0xc0, 0x0e, 0x37, 0xc0, 0x45, 0x71, 0xdf, 0xd9, 0xf6, 0x36, 0xdf, 0x4c, 0x42, 0x72, 0x7b, 0x9e,
|
|
0x86, 0xdd, 0x37, 0xd2, 0xbd, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0xb0, 0xc4, 0xda, 0x5c, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0x7f,
|
|
0x20, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff,
|
|
0xff, 0xff, 0xff, 0x0e, 0x00, 0x00, 0x0b, 0x2f, 0x50, 0x32, 0x53, 0x48, 0x2f, 0x62, 0x74, 0x63,
|
|
0x64, 0x2f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xd2,
|
|
0xea, 0x82, 0x4e, 0xb8, 0x87, 0x42, 0xd0, 0x6d, 0x1f, 0x8d, 0xc3, 0xad, 0x9f, 0x43, 0x9e, 0xed,
|
|
0x6f, 0x43, 0x3c, 0x02, 0x71, 0x71, 0x69, 0xfb, 0xbc, 0x91, 0x44, 0xac, 0xf1, 0x93, 0xd3, 0x18,
|
|
0x17, 0xa9, 0x14, 0xda, 0x17, 0x45, 0xe9, 0xb5, 0x49, 0xbd, 0x0b, 0xfa, 0x1a, 0x56, 0x99, 0x71,
|
|
0xc7, 0x7e, 0xba, 0x30, 0xcd, 0x5a, 0x4b, 0x87,
|
|
}
|
|
|
|
// regTestGenesisBlockBytes are the wire encoded bytes for the genesis block of
|
|
// the regression test network as of protocol version 60002.
|
|
var regTestGenesisBlockBytes = genesisBlockBytes
|
|
|
|
// testNet3GenesisBlockBytes are the wire encoded bytes for the genesis block of
|
|
// the test network (version 3) as of protocol version 60002.
|
|
var testNet3GenesisBlockBytes = genesisBlockBytes
|
|
|
|
// simNetGenesisBlockBytes are the wire encoded bytes for the genesis block of
|
|
// the simulation test network as of protocol version 70002.
|
|
var simNetGenesisBlockBytes = genesisBlockBytes
|