mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-03 03:12:30 +00:00
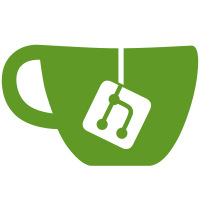
* Add acceptedTransactionIds to GetVirtualSelectedParentChainFromBlockResponseMessage and VirtualSelectedParentChainChangedNotificationMessage * Modify appmessage structs to include new fields * Implement the functionality for acceptedTransactionID notifications * Add missing field for IncludeAcceptedTransactionIds * Notify of block added before notifying that chain changed * Use consensushashing instead of Transaction.ID * Don't notify of empty virtual changes * Don't generate virtualChainChanged notification if there's nobody subscribed * Fix test to not expect empty notifications * Don't generate acceptedTransactionIDs if they were not requested by anyone Co-authored-by: Ori Newman <orinewman1@gmail.com>
42 lines
1.7 KiB
Go
42 lines
1.7 KiB
Go
package rpcclient
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
routerpkg "github.com/kaspanet/kaspad/infrastructure/network/netadapter/router"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// RegisterForVirtualSelectedParentChainChangedNotifications sends an RPC request respective to the function's name and returns the RPC server's response.
|
|
// Additionally, it starts listening for the appropriate notification using the given handler function
|
|
func (c *RPCClient) RegisterForVirtualSelectedParentChainChangedNotifications(includeAcceptedTransactionIDs bool,
|
|
onChainChanged func(notification *appmessage.VirtualSelectedParentChainChangedNotificationMessage)) error {
|
|
|
|
err := c.rpcRouter.outgoingRoute().Enqueue(
|
|
appmessage.NewNotifyVirtualSelectedParentChainChangedRequestMessage(includeAcceptedTransactionIDs))
|
|
if err != nil {
|
|
return err
|
|
}
|
|
response, err := c.route(appmessage.CmdNotifyVirtualSelectedParentChainChangedResponseMessage).DequeueWithTimeout(c.timeout)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
notifyChainChangedResponse := response.(*appmessage.NotifyVirtualSelectedParentChainChangedResponseMessage)
|
|
if notifyChainChangedResponse.Error != nil {
|
|
return c.convertRPCError(notifyChainChangedResponse.Error)
|
|
}
|
|
spawn("RegisterForVirtualSelectedParentChainChangedNotifications", func() {
|
|
for {
|
|
notification, err := c.route(appmessage.CmdVirtualSelectedParentChainChangedNotificationMessage).Dequeue()
|
|
if err != nil {
|
|
if errors.Is(err, routerpkg.ErrRouteClosed) {
|
|
break
|
|
}
|
|
panic(err)
|
|
}
|
|
ChainChangedNotification := notification.(*appmessage.VirtualSelectedParentChainChangedNotificationMessage)
|
|
onChainChanged(ChainChangedNotification)
|
|
}
|
|
})
|
|
return nil
|
|
}
|