mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-20 05:46:44 +00:00
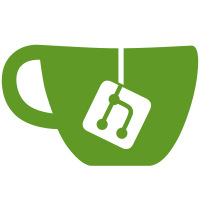
* Add DAAScore, BlueWork, and FinalityPoint to externalapi.BlockHeader. * Add DAAScore, BlueWork, and FinalityPoint to NewImmutableBlockHeader and fix compilation errors. * Add DAAScore, BlueWork, and FinalityPoint to protowire header types and fix failing tests. * Check for header DAA score in validateDifficulty. * Add DAA score to buildBlock. * Fix failing tests. * Add a blue work check in validateDifficultyDAAAndBlueWork. * Add blue work to buildBlock and fix failing tests. * Add finality point validation to ValidateHeaderInContext. * Fix genesis blocks' finality points. * Add finalityPoint to blockBuilder. * Fix tests that failed due to missing reachability data. * Make blockBuilder use VirtualFinalityPoint instead of directly calling FinalityPoint with the virtual hash. * Don't validate the finality point for blocks with trusted data. * Add debug logs. * Skip finality point validation for block whose finality points are the virtual genesis. * Revert "Add debug logs." This reverts commit 3c18f519ccbb382f86f63904dbb1c4cd6bc68b00. * Move checkDAAScore and checkBlueWork to validateBlockHeaderInContext. * Add checkCoinbaseBlueScore to validateBodyInContext. * Fix failing tests. * Add DAAScore, blueWork, and finalityPoint to blocks' hashes. * Generate new genesis blocks. * Fix failing tests. * In BuildUTXOInvalidBlock, get the bits from StageDAADataAndReturnRequiredDifficulty instead of calling RequiredDifficulty separately.
102 lines
2.9 KiB
Go
102 lines
2.9 KiB
Go
// Copyright (c) 2013-2016 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package appmessage
|
|
|
|
import (
|
|
"math/big"
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/davecgh/go-spew/spew"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/util/mstime"
|
|
)
|
|
|
|
// TestBlockHeader tests the MsgBlockHeader API.
|
|
func TestBlockHeader(t *testing.T) {
|
|
nonce := uint64(0xba4d87a69924a93d)
|
|
|
|
hashes := []*externalapi.DomainHash{mainnetGenesisHash, simnetGenesisHash}
|
|
|
|
merkleHash := mainnetGenesisMerkleRoot
|
|
acceptedIDMerkleRoot := exampleAcceptedIDMerkleRoot
|
|
bits := uint32(0x1d00ffff)
|
|
daaScore := uint64(123)
|
|
blueWork := big.NewInt(456)
|
|
finalityPoint := simnetGenesisHash
|
|
bh := NewBlockHeader(1, hashes, merkleHash, acceptedIDMerkleRoot, exampleUTXOCommitment, bits, nonce,
|
|
daaScore, blueWork, finalityPoint)
|
|
|
|
// Ensure we get the same data back out.
|
|
if !reflect.DeepEqual(bh.ParentHashes, hashes) {
|
|
t.Errorf("NewBlockHeader: wrong prev hashes - got %v, want %v",
|
|
spew.Sprint(bh.ParentHashes), spew.Sprint(hashes))
|
|
}
|
|
if bh.HashMerkleRoot != merkleHash {
|
|
t.Errorf("NewBlockHeader: wrong merkle root - got %v, want %v",
|
|
spew.Sprint(bh.HashMerkleRoot), spew.Sprint(merkleHash))
|
|
}
|
|
if bh.Bits != bits {
|
|
t.Errorf("NewBlockHeader: wrong bits - got %v, want %v",
|
|
bh.Bits, bits)
|
|
}
|
|
if bh.Nonce != nonce {
|
|
t.Errorf("NewBlockHeader: wrong nonce - got %v, want %v",
|
|
bh.Nonce, nonce)
|
|
}
|
|
if bh.DAAScore != daaScore {
|
|
t.Errorf("NewBlockHeader: wrong daaScore - got %v, want %v",
|
|
bh.DAAScore, daaScore)
|
|
}
|
|
if bh.BlueWork != blueWork {
|
|
t.Errorf("NewBlockHeader: wrong blueWork - got %v, want %v",
|
|
bh.BlueWork, blueWork)
|
|
}
|
|
if !bh.FinalityPoint.Equal(finalityPoint) {
|
|
t.Errorf("NewBlockHeader: wrong finalityHash - got %v, want %v",
|
|
bh.FinalityPoint, finalityPoint)
|
|
}
|
|
}
|
|
|
|
func TestIsGenesis(t *testing.T) {
|
|
nonce := uint64(123123) // 0x1e0f3
|
|
bits := uint32(0x1d00ffff)
|
|
timestamp := mstime.UnixMilliseconds(0x495fab29000)
|
|
|
|
baseBlockHdr := &MsgBlockHeader{
|
|
Version: 1,
|
|
ParentHashes: []*externalapi.DomainHash{mainnetGenesisHash, simnetGenesisHash},
|
|
HashMerkleRoot: mainnetGenesisMerkleRoot,
|
|
Timestamp: timestamp,
|
|
Bits: bits,
|
|
Nonce: nonce,
|
|
}
|
|
genesisBlockHdr := &MsgBlockHeader{
|
|
Version: 1,
|
|
ParentHashes: []*externalapi.DomainHash{},
|
|
HashMerkleRoot: mainnetGenesisMerkleRoot,
|
|
Timestamp: timestamp,
|
|
Bits: bits,
|
|
Nonce: nonce,
|
|
}
|
|
|
|
tests := []struct {
|
|
in *MsgBlockHeader // Block header to encode
|
|
isGenesis bool // Expected result for call of .IsGenesis
|
|
}{
|
|
{genesisBlockHdr, true},
|
|
{baseBlockHdr, false},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
isGenesis := test.in.IsGenesis()
|
|
if isGenesis != test.isGenesis {
|
|
t.Errorf("MsgBlockHeader.IsGenesis: #%d got: %t, want: %t",
|
|
i, isGenesis, test.isGenesis)
|
|
}
|
|
}
|
|
}
|