mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-01 18:32:32 +00:00
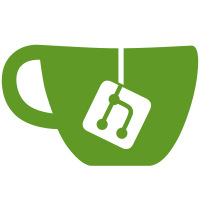
* Add DAAScore, BlueWork, and FinalityPoint to externalapi.BlockHeader. * Add DAAScore, BlueWork, and FinalityPoint to NewImmutableBlockHeader and fix compilation errors. * Add DAAScore, BlueWork, and FinalityPoint to protowire header types and fix failing tests. * Check for header DAA score in validateDifficulty. * Add DAA score to buildBlock. * Fix failing tests. * Add a blue work check in validateDifficultyDAAAndBlueWork. * Add blue work to buildBlock and fix failing tests. * Add finality point validation to ValidateHeaderInContext. * Fix genesis blocks' finality points. * Add finalityPoint to blockBuilder. * Fix tests that failed due to missing reachability data. * Make blockBuilder use VirtualFinalityPoint instead of directly calling FinalityPoint with the virtual hash. * Don't validate the finality point for blocks with trusted data. * Add debug logs. * Skip finality point validation for block whose finality points are the virtual genesis. * Revert "Add debug logs." This reverts commit 3c18f519ccbb382f86f63904dbb1c4cd6bc68b00. * Move checkDAAScore and checkBlueWork to validateBlockHeaderInContext. * Add checkCoinbaseBlueScore to validateBodyInContext. * Fix failing tests. * Add DAAScore, blueWork, and finalityPoint to blocks' hashes. * Generate new genesis blocks. * Fix failing tests. * In BuildUTXOInvalidBlock, get the bits from StageDAADataAndReturnRequiredDifficulty instead of calling RequiredDifficulty separately.
95 lines
2.6 KiB
Go
95 lines
2.6 KiB
Go
package appmessage
|
|
|
|
// SubmitBlockRequestMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type SubmitBlockRequestMessage struct {
|
|
baseMessage
|
|
Block *RPCBlock
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *SubmitBlockRequestMessage) Command() MessageCommand {
|
|
return CmdSubmitBlockRequestMessage
|
|
}
|
|
|
|
// NewSubmitBlockRequestMessage returns a instance of the message
|
|
func NewSubmitBlockRequestMessage(block *RPCBlock) *SubmitBlockRequestMessage {
|
|
return &SubmitBlockRequestMessage{
|
|
Block: block,
|
|
}
|
|
}
|
|
|
|
// RejectReason describes the reason why a block sent by SubmitBlock was rejected
|
|
type RejectReason byte
|
|
|
|
// RejectReason constants
|
|
// Not using iota, since in the .proto file those are hardcoded
|
|
const (
|
|
RejectReasonNone RejectReason = 0
|
|
RejectReasonBlockInvalid RejectReason = 1
|
|
RejectReasonIsInIBD RejectReason = 2
|
|
)
|
|
|
|
var rejectReasonToString = map[RejectReason]string{
|
|
RejectReasonNone: "None",
|
|
RejectReasonBlockInvalid: "Block is invalid",
|
|
RejectReasonIsInIBD: "Node is in IBD",
|
|
}
|
|
|
|
func (rr RejectReason) String() string {
|
|
return rejectReasonToString[rr]
|
|
}
|
|
|
|
// SubmitBlockResponseMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type SubmitBlockResponseMessage struct {
|
|
baseMessage
|
|
RejectReason RejectReason
|
|
Error *RPCError
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *SubmitBlockResponseMessage) Command() MessageCommand {
|
|
return CmdSubmitBlockResponseMessage
|
|
}
|
|
|
|
// NewSubmitBlockResponseMessage returns a instance of the message
|
|
func NewSubmitBlockResponseMessage() *SubmitBlockResponseMessage {
|
|
return &SubmitBlockResponseMessage{}
|
|
}
|
|
|
|
// RPCBlock is a kaspad block representation meant to be
|
|
// used over RPC
|
|
type RPCBlock struct {
|
|
Header *RPCBlockHeader
|
|
Transactions []*RPCTransaction
|
|
VerboseData *RPCBlockVerboseData
|
|
}
|
|
|
|
// RPCBlockHeader is a kaspad block header representation meant to be
|
|
// used over RPC
|
|
type RPCBlockHeader struct {
|
|
Version uint32
|
|
ParentHashes []string
|
|
HashMerkleRoot string
|
|
AcceptedIDMerkleRoot string
|
|
UTXOCommitment string
|
|
Timestamp int64
|
|
Bits uint32
|
|
Nonce uint64
|
|
DAAScore uint64
|
|
BlueWork string
|
|
FinalityPoint string
|
|
}
|
|
|
|
// RPCBlockVerboseData holds verbose data about a block
|
|
type RPCBlockVerboseData struct {
|
|
Hash string
|
|
Difficulty float64
|
|
SelectedParentHash string
|
|
TransactionIDs []string
|
|
IsHeaderOnly bool
|
|
BlueScore uint64
|
|
ChildrenHashes []string
|
|
}
|