mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-11 07:12:33 +00:00
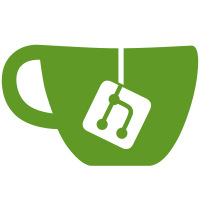
* [NOD-1130] Delete rpcadapters.go. * [NOD-1130] Delete p2p. Move rpc to top level. * [NOD-1130] Remove DAGParams from rpcserverConfig. * [NOD-1130] Remove rpcserverPeer, rpcserverConnManager, rpcserverSyncManager, and rpcserverConfig. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove connmgr and peer. * [NOD-1130] Move rpcmodel into rpc. * [NOD-1130] Implement ConnectionCount. * [NOD-1130] Remove ping and node RPC commands. * [NOD-1130] Dummify handleGetNetTotals. * [NOD-1130] Add NetConnection to Peer. * [NOD-1130] Fix merge errors. * [NOD-1130] Implement Peers. * [NOD-1130] Fix HandleGetConnectedPeerInfo. * [NOD-1130] Fix SendRawTransaction. * [NOD-1130] Rename addManualNode to connect and removeManualNode to disconnect. * [NOD-1130] Add a stub for AddBlock. * [NOD-1130] Fix tests. * [NOD-1130] Replace half-baked contents of RemoveConnection with a stub. * [NOD-1130] Fix merge errors. * [NOD-1130] Make golint happy. * [NOD-1130] Get rid of something weird. * [NOD-1130] Rename minerClient back to client. * [NOD-1130] Add a few fields to GetConnectedPeerInfoResult. * [NOD-1130] Rename oneTry to isPermanent. * [NOD-1130] Implement ConnectionCount in NetAdapter. * [NOD-1130] Move RawMempoolVerbose out of mempool. * [NOD-1130] Move isSynced into the mining package. * [NOD-1130] Fix a compilation error. * [NOD-1130] Make golint happy. * [NOD-1130] Fix merge errors.
81 lines
2.6 KiB
Go
81 lines
2.6 KiB
Go
package rpc
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/hex"
|
|
"fmt"
|
|
"github.com/kaspanet/kaspad/rpc/model"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
"strconv"
|
|
)
|
|
|
|
// handleGetBlockHeader implements the getBlockHeader command.
|
|
func handleGetBlockHeader(s *Server, cmd interface{}, closeChan <-chan struct{}) (interface{}, error) {
|
|
c := cmd.(*model.GetBlockHeaderCmd)
|
|
|
|
// Fetch the header from DAG.
|
|
hash, err := daghash.NewHashFromStr(c.Hash)
|
|
if err != nil {
|
|
return nil, rpcDecodeHexError(c.Hash)
|
|
}
|
|
blockHeader, err := s.dag.HeaderByHash(hash)
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCBlockNotFound,
|
|
Message: "Block not found",
|
|
}
|
|
}
|
|
|
|
// When the verbose flag isn't set, simply return the serialized block
|
|
// header as a hex-encoded string.
|
|
if c.Verbose != nil && !*c.Verbose {
|
|
var headerBuf bytes.Buffer
|
|
err := blockHeader.Serialize(&headerBuf)
|
|
if err != nil {
|
|
context := "Failed to serialize block header"
|
|
return nil, internalRPCError(err.Error(), context)
|
|
}
|
|
return hex.EncodeToString(headerBuf.Bytes()), nil
|
|
}
|
|
|
|
// The verbose flag is set, so generate the JSON object and return it.
|
|
|
|
// Get the hashes for the next blocks unless there are none.
|
|
childHashes, err := s.dag.ChildHashesByHash(hash)
|
|
if err != nil {
|
|
context := "No next block"
|
|
return nil, internalRPCError(err.Error(), context)
|
|
}
|
|
childHashStrings := daghash.Strings(childHashes)
|
|
|
|
blockConfirmations, err := s.dag.BlockConfirmationsByHash(hash)
|
|
if err != nil {
|
|
context := "Could not get block confirmations"
|
|
return nil, internalRPCError(err.Error(), context)
|
|
}
|
|
|
|
selectedParentHash, err := s.dag.SelectedParentHash(hash)
|
|
if err != nil {
|
|
context := "Could not get block selected parent"
|
|
return nil, internalRPCError(err.Error(), context)
|
|
}
|
|
|
|
params := s.dag.Params
|
|
blockHeaderReply := model.GetBlockHeaderVerboseResult{
|
|
Hash: c.Hash,
|
|
Confirmations: blockConfirmations,
|
|
Version: blockHeader.Version,
|
|
VersionHex: fmt.Sprintf("%08x", blockHeader.Version),
|
|
HashMerkleRoot: blockHeader.HashMerkleRoot.String(),
|
|
AcceptedIDMerkleRoot: blockHeader.AcceptedIDMerkleRoot.String(),
|
|
ChildHashes: childHashStrings,
|
|
ParentHashes: daghash.Strings(blockHeader.ParentHashes),
|
|
SelectedParentHash: selectedParentHash.String(),
|
|
Nonce: blockHeader.Nonce,
|
|
Time: blockHeader.Timestamp.UnixMilliseconds(),
|
|
Bits: strconv.FormatInt(int64(blockHeader.Bits), 16),
|
|
Difficulty: getDifficultyRatio(blockHeader.Bits, params),
|
|
}
|
|
return blockHeaderReply, nil
|
|
}
|