mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
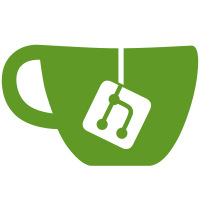
* [NOD-1130] Delete rpcadapters.go. * [NOD-1130] Delete p2p. Move rpc to top level. * [NOD-1130] Remove DAGParams from rpcserverConfig. * [NOD-1130] Remove rpcserverPeer, rpcserverConnManager, rpcserverSyncManager, and rpcserverConfig. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove connmgr and peer. * [NOD-1130] Move rpcmodel into rpc. * [NOD-1130] Implement ConnectionCount. * [NOD-1130] Remove ping and node RPC commands. * [NOD-1130] Dummify handleGetNetTotals. * [NOD-1130] Add NetConnection to Peer. * [NOD-1130] Fix merge errors. * [NOD-1130] Implement Peers. * [NOD-1130] Fix HandleGetConnectedPeerInfo. * [NOD-1130] Fix SendRawTransaction. * [NOD-1130] Rename addManualNode to connect and removeManualNode to disconnect. * [NOD-1130] Add a stub for AddBlock. * [NOD-1130] Fix tests. * [NOD-1130] Replace half-baked contents of RemoveConnection with a stub. * [NOD-1130] Fix merge errors. * [NOD-1130] Make golint happy. * [NOD-1130] Get rid of something weird. * [NOD-1130] Rename minerClient back to client. * [NOD-1130] Add a few fields to GetConnectedPeerInfoResult. * [NOD-1130] Rename oneTry to isPermanent. * [NOD-1130] Implement ConnectionCount in NetAdapter. * [NOD-1130] Move RawMempoolVerbose out of mempool. * [NOD-1130] Move isSynced into the mining package. * [NOD-1130] Fix a compilation error. * [NOD-1130] Make golint happy. * [NOD-1130] Fix merge errors.
91 lines
2.4 KiB
Go
91 lines
2.4 KiB
Go
package rpc
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/kaspanet/kaspad/rpc/model"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
)
|
|
|
|
const (
|
|
// maxBlocksInGetChainFromBlockResult is the max amount of blocks that
|
|
// are allowed in a GetChainFromBlockResult.
|
|
maxBlocksInGetChainFromBlockResult = 1000
|
|
)
|
|
|
|
// handleGetChainFromBlock implements the getChainFromBlock command.
|
|
func handleGetChainFromBlock(s *Server, cmd interface{}, closeChan <-chan struct{}) (interface{}, error) {
|
|
if s.acceptanceIndex == nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCNoAcceptanceIndex,
|
|
Message: "The acceptance index must be " +
|
|
"enabled to get the selected parent chain " +
|
|
"(specify --acceptanceindex)",
|
|
}
|
|
}
|
|
|
|
c := cmd.(*model.GetChainFromBlockCmd)
|
|
var startHash *daghash.Hash
|
|
if c.StartHash != nil {
|
|
startHash = &daghash.Hash{}
|
|
err := daghash.Decode(startHash, *c.StartHash)
|
|
if err != nil {
|
|
return nil, rpcDecodeHexError(*c.StartHash)
|
|
}
|
|
}
|
|
|
|
s.dag.RLock()
|
|
defer s.dag.RUnlock()
|
|
|
|
// If startHash is not in the selected parent chain, there's nothing
|
|
// to do; return an error.
|
|
if startHash != nil && !s.dag.IsInDAG(startHash) {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCBlockNotFound,
|
|
Message: "Block not found in the DAG",
|
|
}
|
|
}
|
|
|
|
// Retrieve the selected parent chain.
|
|
removedChainHashes, addedChainHashes, err := s.dag.SelectedParentChain(startHash)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// Limit the amount of blocks in the response
|
|
if len(addedChainHashes) > maxBlocksInGetChainFromBlockResult {
|
|
addedChainHashes = addedChainHashes[:maxBlocksInGetChainFromBlockResult]
|
|
}
|
|
|
|
// Collect addedChainBlocks.
|
|
addedChainBlocks, err := collectChainBlocks(s, addedChainHashes)
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInternal.Code,
|
|
Message: fmt.Sprintf("could not collect chain blocks: %s", err),
|
|
}
|
|
}
|
|
|
|
// Collect removedHashes.
|
|
removedHashes := make([]string, len(removedChainHashes))
|
|
for i, hash := range removedChainHashes {
|
|
removedHashes[i] = hash.String()
|
|
}
|
|
|
|
result := &model.GetChainFromBlockResult{
|
|
RemovedChainBlockHashes: removedHashes,
|
|
AddedChainBlocks: addedChainBlocks,
|
|
Blocks: nil,
|
|
}
|
|
|
|
// If the user specified to include the blocks, collect them as well.
|
|
if c.IncludeBlocks {
|
|
getBlockVerboseResults, err := hashesToGetBlockVerboseResults(s, addedChainHashes)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
result.Blocks = getBlockVerboseResults
|
|
}
|
|
|
|
return result, nil
|
|
}
|