mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
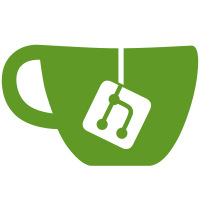
* [NOD-1130] Delete rpcadapters.go. * [NOD-1130] Delete p2p. Move rpc to top level. * [NOD-1130] Remove DAGParams from rpcserverConfig. * [NOD-1130] Remove rpcserverPeer, rpcserverConnManager, rpcserverSyncManager, and rpcserverConfig. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove wallet RPC commands. * [NOD-1130] Remove connmgr and peer. * [NOD-1130] Move rpcmodel into rpc. * [NOD-1130] Implement ConnectionCount. * [NOD-1130] Remove ping and node RPC commands. * [NOD-1130] Dummify handleGetNetTotals. * [NOD-1130] Add NetConnection to Peer. * [NOD-1130] Fix merge errors. * [NOD-1130] Implement Peers. * [NOD-1130] Fix HandleGetConnectedPeerInfo. * [NOD-1130] Fix SendRawTransaction. * [NOD-1130] Rename addManualNode to connect and removeManualNode to disconnect. * [NOD-1130] Add a stub for AddBlock. * [NOD-1130] Fix tests. * [NOD-1130] Replace half-baked contents of RemoveConnection with a stub. * [NOD-1130] Fix merge errors. * [NOD-1130] Make golint happy. * [NOD-1130] Get rid of something weird. * [NOD-1130] Rename minerClient back to client. * [NOD-1130] Add a few fields to GetConnectedPeerInfoResult. * [NOD-1130] Rename oneTry to isPermanent. * [NOD-1130] Implement ConnectionCount in NetAdapter. * [NOD-1130] Move RawMempoolVerbose out of mempool. * [NOD-1130] Move isSynced into the mining package. * [NOD-1130] Fix a compilation error. * [NOD-1130] Make golint happy. * [NOD-1130] Fix merge errors.
64 lines
1.8 KiB
Go
64 lines
1.8 KiB
Go
package rpc
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/rpc/model"
|
|
"github.com/kaspanet/kaspad/util/subnetworkid"
|
|
)
|
|
|
|
// handleNotifyNewTransations implements the notifyNewTransactions command
|
|
// extension for websocket connections.
|
|
func handleNotifyNewTransactions(wsc *wsClient, icmd interface{}) (interface{}, error) {
|
|
cmd, ok := icmd.(*model.NotifyNewTransactionsCmd)
|
|
if !ok {
|
|
return nil, model.ErrRPCInternal
|
|
}
|
|
|
|
isVerbose := cmd.Verbose != nil && *cmd.Verbose
|
|
if !isVerbose && cmd.Subnetwork != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidParameter,
|
|
Message: "Subnetwork switch is only allowed if verbose=true",
|
|
}
|
|
}
|
|
|
|
var subnetworkID *subnetworkid.SubnetworkID
|
|
if cmd.Subnetwork != nil {
|
|
var err error
|
|
subnetworkID, err = subnetworkid.NewFromStr(*cmd.Subnetwork)
|
|
if err != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidParameter,
|
|
Message: "Subnetwork is malformed",
|
|
}
|
|
}
|
|
}
|
|
|
|
if isVerbose {
|
|
nodeSubnetworkID := wsc.server.dag.SubnetworkID()
|
|
if nodeSubnetworkID.IsEqual(subnetworkid.SubnetworkIDNative) && subnetworkID != nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidParameter,
|
|
Message: "Subnetwork switch is disabled when node is in Native subnetwork",
|
|
}
|
|
} else if nodeSubnetworkID != nil {
|
|
if subnetworkID == nil {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidParameter,
|
|
Message: "Subnetwork switch is required when node is partial",
|
|
}
|
|
}
|
|
if !nodeSubnetworkID.IsEqual(subnetworkID) {
|
|
return nil, &model.RPCError{
|
|
Code: model.ErrRPCInvalidParameter,
|
|
Message: "Subnetwork must equal the node's subnetwork when the node is partial",
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
wsc.verboseTxUpdates = isVerbose
|
|
wsc.subnetworkIDForTxUpdates = subnetworkID
|
|
wsc.server.ntfnMgr.RegisterNewMempoolTxsUpdates(wsc)
|
|
return nil, nil
|
|
}
|