mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 06:12:32 +00:00
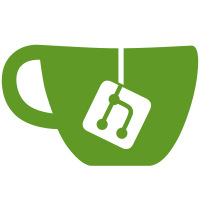
* Get rid of insertMode * Rename AddBlockToVirtual->AddBlock * When F is not in the future of P, enforce finality with P and not with F. * Don't allow blocks with invalid parents or with missing block body * Check finality violation before checking block status * Implement CalculateIndependentPruningPoint * Move checkBlockStatus to validateBlock * Add ValidateBlock to block processor interface * Adjust SetPruningPoint to the new IBD flow * Add pruning store to CSM's constructor * Flip wrong condition on AddHeaderTip * Fix func (hts *headerSelectedTipStore) Has * Fix block stage order * Call to ValidateBodyInContext from validatePostProofOfWork * Enable overrideDAGParams * Update log * Rename SetPruningPoint to ValidateAndInsertPruningPoint and move most of its logic inside block processor * Rename hasValidatedHeader->hasValidatedOnlyHeader * Fix typo * Name return values for fetchMissingUTXOSet * Add comment * Return ErrMissingParents when block body is missing * Add logs and comments * Fix merge error * Fix pruning point calculation to be by virtual selected parent * Replace CalculateIndependentPruningPoint to CalculatePruningPointByHeaderSelectedTip * Fix isAwaitingUTXOSet to check pruning point by headers * Change isAwaitingUTXOSet indication * Remove IsBlockInHeaderPruningPointFuture from BlockInfo * Fix LowestChainBlockAboveOrEqualToBlueScore * Add validateNewPruningPointTransactions * Add validateNewPruningAgainstPastUTXO * Rename set_pruning_utxo_set.go to update_pruning_utxo_set.go * Check missing block body hashes by missing block instead of status * Validate pruning point against past UTXO with the pruning point as block hash * Remove virtualHeaderHash * Fix comment * Fix imports
124 lines
4.1 KiB
Go
124 lines
4.1 KiB
Go
package consensusstatemanager
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// consensusStateManager manages the node's consensus state
|
|
type consensusStateManager struct {
|
|
pruningDepth uint64
|
|
maxMassAcceptedByBlock uint64
|
|
maxBlockParents model.KType
|
|
mergeSetSizeLimit uint64
|
|
genesisHash *externalapi.DomainHash
|
|
databaseContext model.DBManager
|
|
|
|
ghostdagManager model.GHOSTDAGManager
|
|
dagTopologyManager model.DAGTopologyManager
|
|
dagTraversalManager model.DAGTraversalManager
|
|
pastMedianTimeManager model.PastMedianTimeManager
|
|
transactionValidator model.TransactionValidator
|
|
blockValidator model.BlockValidator
|
|
reachabilityManager model.ReachabilityManager
|
|
coinbaseManager model.CoinbaseManager
|
|
mergeDepthManager model.MergeDepthManager
|
|
finalityManager model.FinalityManager
|
|
|
|
headersSelectedTipStore model.HeaderSelectedTipStore
|
|
blockStatusStore model.BlockStatusStore
|
|
ghostdagDataStore model.GHOSTDAGDataStore
|
|
consensusStateStore model.ConsensusStateStore
|
|
multisetStore model.MultisetStore
|
|
blockStore model.BlockStore
|
|
utxoDiffStore model.UTXODiffStore
|
|
blockRelationStore model.BlockRelationStore
|
|
acceptanceDataStore model.AcceptanceDataStore
|
|
blockHeaderStore model.BlockHeaderStore
|
|
pruningStore model.PruningStore
|
|
|
|
stores []model.Store
|
|
}
|
|
|
|
// New instantiates a new ConsensusStateManager
|
|
func New(
|
|
databaseContext model.DBManager,
|
|
pruningDepth uint64,
|
|
maxMassAcceptedByBlock uint64,
|
|
maxBlockParents model.KType,
|
|
mergeSetSizeLimit uint64,
|
|
genesisHash *externalapi.DomainHash,
|
|
|
|
ghostdagManager model.GHOSTDAGManager,
|
|
dagTopologyManager model.DAGTopologyManager,
|
|
dagTraversalManager model.DAGTraversalManager,
|
|
pastMedianTimeManager model.PastMedianTimeManager,
|
|
transactionValidator model.TransactionValidator,
|
|
blockValidator model.BlockValidator,
|
|
reachabilityManager model.ReachabilityManager,
|
|
coinbaseManager model.CoinbaseManager,
|
|
mergeDepthManager model.MergeDepthManager,
|
|
finalityManager model.FinalityManager,
|
|
|
|
blockStatusStore model.BlockStatusStore,
|
|
ghostdagDataStore model.GHOSTDAGDataStore,
|
|
consensusStateStore model.ConsensusStateStore,
|
|
multisetStore model.MultisetStore,
|
|
blockStore model.BlockStore,
|
|
utxoDiffStore model.UTXODiffStore,
|
|
blockRelationStore model.BlockRelationStore,
|
|
acceptanceDataStore model.AcceptanceDataStore,
|
|
blockHeaderStore model.BlockHeaderStore,
|
|
headersSelectedTipStore model.HeaderSelectedTipStore,
|
|
pruningStore model.PruningStore) (model.ConsensusStateManager, error) {
|
|
|
|
csm := &consensusStateManager{
|
|
pruningDepth: pruningDepth,
|
|
maxMassAcceptedByBlock: maxMassAcceptedByBlock,
|
|
maxBlockParents: maxBlockParents,
|
|
mergeSetSizeLimit: mergeSetSizeLimit,
|
|
genesisHash: genesisHash,
|
|
databaseContext: databaseContext,
|
|
|
|
ghostdagManager: ghostdagManager,
|
|
dagTopologyManager: dagTopologyManager,
|
|
dagTraversalManager: dagTraversalManager,
|
|
pastMedianTimeManager: pastMedianTimeManager,
|
|
transactionValidator: transactionValidator,
|
|
blockValidator: blockValidator,
|
|
reachabilityManager: reachabilityManager,
|
|
coinbaseManager: coinbaseManager,
|
|
mergeDepthManager: mergeDepthManager,
|
|
finalityManager: finalityManager,
|
|
|
|
multisetStore: multisetStore,
|
|
blockStore: blockStore,
|
|
blockStatusStore: blockStatusStore,
|
|
ghostdagDataStore: ghostdagDataStore,
|
|
consensusStateStore: consensusStateStore,
|
|
utxoDiffStore: utxoDiffStore,
|
|
blockRelationStore: blockRelationStore,
|
|
acceptanceDataStore: acceptanceDataStore,
|
|
blockHeaderStore: blockHeaderStore,
|
|
headersSelectedTipStore: headersSelectedTipStore,
|
|
pruningStore: pruningStore,
|
|
|
|
stores: []model.Store{
|
|
consensusStateStore,
|
|
acceptanceDataStore,
|
|
blockStore,
|
|
blockStatusStore,
|
|
blockRelationStore,
|
|
multisetStore,
|
|
ghostdagDataStore,
|
|
consensusStateStore,
|
|
utxoDiffStore,
|
|
blockHeaderStore,
|
|
headersSelectedTipStore,
|
|
pruningStore,
|
|
},
|
|
}
|
|
|
|
return csm, nil
|
|
}
|