mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 15:26:42 +00:00
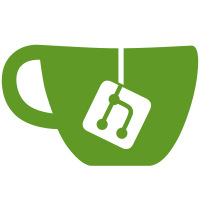
* [NOD-1422] Implement GHOSTDAG * [NOD-1422] Rename bluest->findSelectedParent * [NOD-1422] Remove preallocations from MergeSetBlues and add preallocation in candidateBluesAnticoneSizes * [NOD-1422] Rename blockghostdagdata.go to ghostdag.go
36 lines
1022 B
Go
36 lines
1022 B
Go
package ghostdagmanager
|
|
|
|
import "github.com/kaspanet/kaspad/domain/consensus/model"
|
|
|
|
func (gm *GHOSTDAGManager) findSelectedParent(parentHashes []*model.DomainHash) *model.DomainHash {
|
|
var selectedParent *model.DomainHash
|
|
for _, hash := range parentHashes {
|
|
if selectedParent == nil || gm.less(selectedParent, hash) {
|
|
selectedParent = hash
|
|
}
|
|
}
|
|
return selectedParent
|
|
}
|
|
|
|
func (gm *GHOSTDAGManager) less(blockA, blockB *model.DomainHash) bool {
|
|
blockABlueScore := gm.ghostdagDataStore.Get(gm.databaseContext, blockA).BlueScore
|
|
blockBBlueScore := gm.ghostdagDataStore.Get(gm.databaseContext, blockB).BlueScore
|
|
if blockABlueScore == blockBBlueScore {
|
|
return hashesLess(blockA, blockB)
|
|
}
|
|
return blockABlueScore < blockBBlueScore
|
|
}
|
|
|
|
func hashesLess(a, b *model.DomainHash) bool {
|
|
// We compare the hashes backwards because Hash is stored as a little endian byte array.
|
|
for i := len(a) - 1; i >= 0; i-- {
|
|
switch {
|
|
case a[i] < b[i]:
|
|
return true
|
|
case a[i] > b[i]:
|
|
return false
|
|
}
|
|
}
|
|
return false
|
|
}
|