mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-07 21:32:32 +00:00
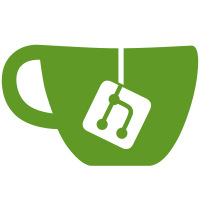
* Implement bip32 * Unite private and public extended keys * Change variable names * Change test name and add comment * Rename var name * Rename ckd.go to child_key_derivation.go * Rename ser32 -> serializeUint32 * Add PrivateKey method * Rename Path -> DeriveFromPath * Add comment to validateChecksum * Remove redundant condition from parsePath * Rename Fingerprint->ParentFingerprint * Merge hardened and non-hardened paths in calcI * Change fingerPrintFromPoint to method * Move hash160 to hash.go * Fix a bug in calcI * Simplify doubleSha256 * Remove slice end bound * Split long line * Change KaspaMainnetPrivate/public to represent kprv/kpub * Add comments * Fix comment * Copy base58 library to kaspad * Add versions for all networks * Change versions to hex * Add comments Co-authored-by: Svarog <feanorr@gmail.com> Co-authored-by: Elichai Turkel <elichai.turkel@gmail.com>
61 lines
1.2 KiB
Go
61 lines
1.2 KiB
Go
package bip32
|
|
|
|
import (
|
|
"github.com/pkg/errors"
|
|
"strconv"
|
|
"strings"
|
|
)
|
|
|
|
type path struct {
|
|
isPrivate bool
|
|
indexes []uint32
|
|
}
|
|
|
|
func parsePath(pathString string) (*path, error) {
|
|
parts := strings.Split(pathString, "/")
|
|
isPrivate := false
|
|
switch parts[0] {
|
|
case "m":
|
|
isPrivate = true
|
|
case "M":
|
|
isPrivate = false
|
|
default:
|
|
return nil, errors.Errorf("%s is an invalid extended key type", parts[0])
|
|
}
|
|
|
|
indexParts := parts[1:]
|
|
indexes := make([]uint32, len(indexParts))
|
|
for i, part := range indexParts {
|
|
var err error
|
|
indexes[i], err = parseIndex(part)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
|
|
return &path{
|
|
isPrivate: isPrivate,
|
|
indexes: indexes,
|
|
}, nil
|
|
}
|
|
|
|
func parseIndex(indexString string) (uint32, error) {
|
|
const isHardenedSuffix = "'"
|
|
isHardened := strings.HasSuffix(indexString, isHardenedSuffix)
|
|
trimmedIndexString := strings.TrimSuffix(indexString, isHardenedSuffix)
|
|
index, err := strconv.Atoi(trimmedIndexString)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
|
|
if index >= hardenedIndexStart {
|
|
return 0, errors.Errorf("max index value is %d but got %d", hardenedIndexStart, index)
|
|
}
|
|
|
|
if isHardened {
|
|
return uint32(index) + hardenedIndexStart, nil
|
|
}
|
|
|
|
return uint32(index), nil
|
|
}
|