mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-24 15:56:42 +00:00
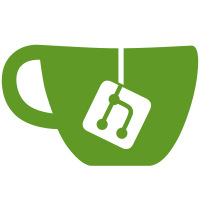
* [NOD-1517] Properly initialize consensus with Genesis block * [NOD-1517] Remove redundant AddHeaderTip * [NOD-1517] Don't return nil from dbHash<->DomainHash converters * [NOD-1517] Use pointer receivers * [NOD-1517] Use domain block in dagParams * [NOD-1517] Remove boolean from SelectedTip * [NOD-1517] Rename hasHeader to isHeadersOnlyBlock * [NOD-1517] Add comment * [NOD-1517] Change genesis version * [NOD-1517] Rename TestNewFactory->TestNewConsensus
58 lines
1.2 KiB
Go
58 lines
1.2 KiB
Go
package consensusstatestore
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// consensusStateStore represents a store for the current consensus state
|
|
type consensusStateStore struct {
|
|
stagedTips []*externalapi.DomainHash
|
|
stagedVirtualDiffParents []*externalapi.DomainHash
|
|
stagedVirtualUTXODiff *model.UTXODiff
|
|
stagedVirtualUTXOSet model.UTXOCollection
|
|
}
|
|
|
|
// New instantiates a new ConsensusStateStore
|
|
func New() model.ConsensusStateStore {
|
|
return &consensusStateStore{}
|
|
}
|
|
|
|
func (c *consensusStateStore) Discard() {
|
|
c.stagedTips = nil
|
|
c.stagedVirtualUTXODiff = nil
|
|
c.stagedVirtualDiffParents = nil
|
|
c.stagedVirtualUTXOSet = nil
|
|
}
|
|
|
|
func (c *consensusStateStore) Commit(dbTx model.DBTransaction) error {
|
|
err := c.commitTips(dbTx)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
err = c.commitVirtualDiffParents(dbTx)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = c.commitVirtualUTXODiff(dbTx)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = c.commitVirtualUTXOSet(dbTx)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
c.Discard()
|
|
|
|
return nil
|
|
}
|
|
|
|
func (c *consensusStateStore) IsStaged() bool {
|
|
return c.stagedTips != nil ||
|
|
c.stagedVirtualDiffParents != nil ||
|
|
c.stagedVirtualUTXODiff != nil
|
|
}
|