mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
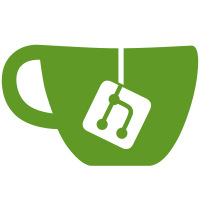
* [NOD-350] Implement testnet faucet * [NOD-350] Add JSON annotations to api server response types * [NOD-350] Fix IP check query, update IP usage with upsert, and make IP a primary key * [NOD-377] Remove redundant float conversion * [NOD-377] Change not current database error message * [NOD-377] change API route from /money_request to /request_money * [NOD-377] Add a constant for 24 hours * [NOD-377] Remove redundant call for getWalletUTXOSet() * [NOD-377] Condition refactoring * [NOD-377] Fix POST request to API server content type * [NOD-350] Rename day -> timeBetweenRequests * [NOD-377] Rename timeBetweenRequests -> minRequestInterval, timeBefore24Hours -> minRequestInterval * [NOD-350] Rename file responsetypes -> response_types * [NOD-350] Rename convertTxModelToTxResponse -> convertTxDBModelToTxResponse * [NOD-350] Explicitly select blue_score in fetchSelectedTipBlueScore * [NOD-350] Refactor and add comments * [NOD-350] Make calcFee use MassPerTxByte * [NOD-350] Convert IP column to varchar(39) to allow ipv6 addresses * [NOD-350] Add comments to isFundedAndIsChangeOutputRequired * [NOD-350] Remove approximateConfirmationsForCoinbaseMaturity * [NOD-350] Fix comments
80 lines
2.3 KiB
Go
80 lines
2.3 KiB
Go
package httpserverutils
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
)
|
|
|
|
type contextKey string
|
|
|
|
const (
|
|
contextKeyRequestID contextKey = "REQUEST_ID"
|
|
)
|
|
|
|
// ServerContext is a context.Context wrapper that
|
|
// enables custom logs with request ID.
|
|
type ServerContext struct {
|
|
context.Context
|
|
}
|
|
|
|
// ToServerContext takes a context.Context instance
|
|
// and converts it to *ServerContext.
|
|
func ToServerContext(ctx context.Context) *ServerContext {
|
|
if asCtx, ok := ctx.(*ServerContext); ok {
|
|
return asCtx
|
|
}
|
|
return &ServerContext{Context: ctx}
|
|
}
|
|
|
|
// SetRequestID associates a request ID for the context.
|
|
func (ctx *ServerContext) SetRequestID(requestID uint64) context.Context {
|
|
context.WithValue(ctx, contextKeyRequestID, requestID)
|
|
return ctx
|
|
}
|
|
|
|
func (ctx *ServerContext) requestID() uint64 {
|
|
id := ctx.Value(contextKeyRequestID)
|
|
uint64ID, _ := id.(uint64)
|
|
return uint64ID
|
|
}
|
|
|
|
func (ctx *ServerContext) getLogString(format string, params ...interface{}) string {
|
|
return fmt.Sprintf("RID %d: ", ctx.requestID()) + fmt.Sprintf(format, params...)
|
|
}
|
|
|
|
// Tracef writes a customized formatted context
|
|
// related log with log level 'Trace'.
|
|
func (ctx *ServerContext) Tracef(format string, params ...interface{}) {
|
|
log.Trace(ctx.getLogString(format, params...))
|
|
}
|
|
|
|
// Debugf writes a customized formatted context
|
|
// related log with log level 'Debug'.
|
|
func (ctx *ServerContext) Debugf(format string, params ...interface{}) {
|
|
log.Debug(ctx.getLogString(format, params...))
|
|
}
|
|
|
|
// Infof writes a customized formatted context
|
|
// related log with log level 'Info'.
|
|
func (ctx *ServerContext) Infof(format string, params ...interface{}) {
|
|
log.Info(ctx.getLogString(format, params...))
|
|
}
|
|
|
|
// Warnf writes a customized formatted context
|
|
// related log with log level 'Warn'.
|
|
func (ctx *ServerContext) Warnf(format string, params ...interface{}) {
|
|
log.Warn(ctx.getLogString(format, params...))
|
|
}
|
|
|
|
// Errorf writes a customized formatted context
|
|
// related log with log level 'Error'.
|
|
func (ctx *ServerContext) Errorf(format string, params ...interface{}) {
|
|
log.Error(ctx.getLogString(format, params...))
|
|
}
|
|
|
|
// Criticalf writes a customized formatted context
|
|
// related log with log level 'Critical'.
|
|
func (ctx *ServerContext) Criticalf(format string, params ...interface{}) {
|
|
log.Criticalf(ctx.getLogString(format, params...))
|
|
}
|