mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
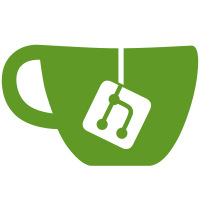
* [NOD-208] Added blockBlueScore to UTXOEntry. * [NOD-208] Added blueBlockScore to NewUTXOEntry. * [NOD-208] Fixed compilation errors in policy, utxoset, and dag tests. * [NOD-208] Changed validateBlockRewardMaturity and CheckTransactionInputsAndCalulateFee to use blueScore. * [NOD-208] Changed CalcBlockSubsidy to use blueScore. * [NOD-208] Changed SequenceLockActive to use blueScore. * [NOD-208] Removed ExtractCoinbaseHeight. * [NOD-208] Removed reference to block height in ensureNoDuplicateTx. * [NOD-208] Changed IsFinalizedTransaction to use blueScore. * [NOD-208] Fixed merge errors. * [NOD-208] Made UTXOEntry serialization use blueScore. * [NOD-208] Changed CalcPriority and calcInputValueAge to use blueScore. * [NOD-208] Changed calcSequenceLock to use blueScore. * [NOD-208] Removed blockChainHeight from UTXOEntry. * [NOD-208] Fixed compilation errors in feeEstimator. Fixed a bug in the test pool hardness. * [NOD-208] Fixed oldestChainBlockWithBlueScoreGreaterThan not handling an extreme case. * [NOD-208] Fixed TestDiffFromTx. * [NOD-208] Got rid of priority and support of free transactions. * [NOD-208] Fixed TestProcessTransaction. * [NOD-208] Fixed TestTxFeePrioHeap. * [NOD-208] Fixed TestAddrIndex and TestFeeEstimatorCfg. * [NOD-208] Removed unused rateLimit parameter from ProcessTransaction. * [NOD-208] Fixed tests that rely on CreateTxChain. * [NOD-208] Fixed tests that rely on CreateSignedTxForSubnetwork. * [NOD-208] Fixed TestFetchTransaction. * [NOD-208] Fixed TestHandleNewBlock. Fixed HandleNewBlock erroneously processing fee transactions. * [NOD-208] Fixed TestTxIndexConnectBlock. * [NOD-208] Removed the use of Height() from the fee estimator. * [NOD-208] Removed unused methods from rpcwebsocket.go. * [NOD-208] Removed Height from util.Block. * [NOD-208] Removed ErrForkTooOld. It doesn't make sense in a DAG. * [NOD-208] Made blockHeap use blueScore instead of height. * [NOD-208] Removed fee estimator. * [NOD-208] Removed DAG.Height. * [NOD-208] Made TestAncestorErrors test chainHeight instead of height. * [NOD-208] Fixed a couple of comments that were still speaking about block height. * [NOD-208] Replaced all uses of HighestTipHash with SelectedTipHash. * [NOD-208] Remove blockNode highest and some remaining erroneous uses of height. * [NOD-208] Fixed a couple of comments. Fixed outPoint -> outpoint merge error. * [NOD-208] Fixed a couple more comments. * [NOD-208] Used calcMinRequiredTxRelayFee instead of DefaultMinRelayTxFee for mempool tests. * [NOD-208] Renamed mempool Config BestHeight to DAGChainHeight. * [NOD-208] Fixed a bug in oldestChainBlockWithBlueScoreGreaterThan. Made calcSequenceLock use the node's selected parent chain rather than the virtual block's. * [NOD-208] Removed chainHeight from blockNode String(). Renamed checkpointsByHeight to checkpointsByChainHeight and prevCheckpointHeight to prevCheckpointChainHeight. Removed reference to chainHeight in blockIndexKey. Fixed comments in dagio.go. * [NOD-208] Removed indexers/blocklogger.go, as no one was using it. * [NOD-208] Made blocklogger.go log blueScore instead of height. * [NOD-208] Fixed typo. * [NOD-208] Fixed comments, did minor renaming. * [NOD-208] Made a "common sense" wrapper around sort.Search. * [NOD-208] Fixed comment in SearchSlice.
36 lines
962 B
Go
36 lines
962 B
Go
// Copyright (c) 2017 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package netsync
|
|
|
|
import (
|
|
"github.com/daglabs/btcd/blockdag"
|
|
"github.com/daglabs/btcd/dagconfig"
|
|
"github.com/daglabs/btcd/mempool"
|
|
"github.com/daglabs/btcd/util"
|
|
"github.com/daglabs/btcd/wire"
|
|
)
|
|
|
|
// PeerNotifier exposes methods to notify peers of status changes to
|
|
// transactions, blocks, etc. Currently server (in the main package) implements
|
|
// this interface.
|
|
type PeerNotifier interface {
|
|
AnnounceNewTransactions(newTxs []*mempool.TxDesc)
|
|
|
|
RelayInventory(invVect *wire.InvVect, data interface{})
|
|
|
|
TransactionConfirmed(tx *util.Tx)
|
|
}
|
|
|
|
// Config is a configuration struct used to initialize a new SyncManager.
|
|
type Config struct {
|
|
PeerNotifier PeerNotifier
|
|
DAG *blockdag.BlockDAG
|
|
TxMemPool *mempool.TxPool
|
|
ChainParams *dagconfig.Params
|
|
|
|
DisableCheckpoints bool
|
|
MaxPeers int
|
|
}
|