mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
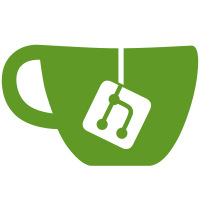
* [NOD-885] Create database.Key type * [NOD-885] Rename FullKey()->FullKeyBytes() and Key()->KeyBytes() * [NOD-885] Make Key.String return a hex string * [NOD-885] Rename key parts * [NOD-885] Rename separator->bucketSeparator * [NOD-885] Rename SuffixBytes->Suffix and PrefixBytes->Prefix * [NOD-885] Change comments * [NOD-885] Change key prefix to bucket * [NOD-885] Don't use database.NewKey inside dbaccess * [NOD-885] Fix nil bug in Bucket.Path() * [NOD-885] Rename helpers.go -> keys.go * [NOD-885] Unexport database.NewKey * [NOD-885] Remove redundant code in Bucket.Path()
47 lines
1.1 KiB
Go
47 lines
1.1 KiB
Go
package dbaccess
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/database"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
)
|
|
|
|
var multisetBucket = database.MakeBucket([]byte("multiset"))
|
|
|
|
func multisetKey(hash *daghash.Hash) *database.Key {
|
|
return multisetBucket.Key(hash[:])
|
|
}
|
|
|
|
// MultisetCursor opens a cursor over all the
|
|
// multiset entries.
|
|
func MultisetCursor(context Context) (database.Cursor, error) {
|
|
accessor, err := context.accessor()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return accessor.Cursor(multisetBucket)
|
|
}
|
|
|
|
// StoreMultiset stores the multiset of a block by its hash.
|
|
func StoreMultiset(context Context, blockHash *daghash.Hash, multiset []byte) error {
|
|
accessor, err := context.accessor()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
key := multisetKey(blockHash)
|
|
return accessor.Put(key, multiset)
|
|
}
|
|
|
|
// HasMultiset returns whether the multiset of
|
|
// the given block exists in the database.
|
|
func HasMultiset(context Context, blockHash *daghash.Hash) (bool, error) {
|
|
accessor, err := context.accessor()
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
|
|
key := multisetKey(blockHash)
|
|
return accessor.Has(key)
|
|
}
|