mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
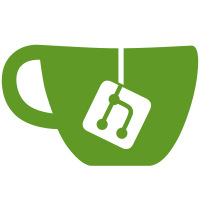
* [NOD-420] Delay blocks with valid timestamp (non-delayed) that point to a delayed block. * [NOD-420] Mark block as requested when setting as delayed. * [NOD-420] Merge master; Use dag.timeSource.AdjustedTime() instead of time.Now; * [NOD-420] Return nil when not expecting an error * [NOD-420] Initialise delyaed blocks mapping * [NOD-420] Trigger delayed blocks processing every time we process a block. * [NOD-420] Hold the read lock in processDelayedBlocks * [NOD-420] Add delayed blocks heap sorted by their process time so we could process them in order. * [NOD-420] Update debug log * [NOD-420] Fix process blocks loop * [NOD-420] Add comment * [NOD-420] Log error message * [NOD-420] Implement peek method for delayed block heap. extract delayed block processing to another function. * [NOD-420] Trigger process delayed blocks only in process block * [NOD-420] Move delayed block addition to process block * [NOD-420] Use process block to make sure we fully process the delayed block and deal with orphans. * [NOD-420] Unexport functions when not needed; Return isDelayed boolean from ProcessBlock instead of the delay duration * [NOd-420] Remove redundant delayedBlocksLock * [NOD-420] Resolve merge conflict; Return delay 0 instead of boolean * [NOD-420] Do not treat delayed block as orphan * [NOD-420] Make sure block is not processed if we have already sa delayed. * [NOD-420] Process delayed block if parent is delayed to make sure it would not be treated as orphan. * [NOD-420] Rename variable * [NOD-420] Rename function. Move maxDelayOfParents to process.go * [NOD-420] Fix typo * [NOD-420] Handle errors from processDelayedBlocks properly * [NOD-420] Return default values if err != nil from dag.addDelayedBlock * [NOD-420] Return default values if err != nil from dag.addDelayedBlock in another place Co-authored-by: Svarog <feanorr@gmail.com>
74 lines
1.7 KiB
Go
74 lines
1.7 KiB
Go
package blockdag
|
|
|
|
import (
|
|
"container/heap"
|
|
)
|
|
|
|
type baseDelayedBlocksHeap []*delayedBlock
|
|
|
|
func (h baseDelayedBlocksHeap) Len() int {
|
|
return len(h)
|
|
}
|
|
func (h baseDelayedBlocksHeap) Swap(i, j int) {
|
|
h[i], h[j] = h[j], h[i]
|
|
}
|
|
|
|
func (h *baseDelayedBlocksHeap) Push(x interface{}) {
|
|
*h = append(*h, x.(*delayedBlock))
|
|
}
|
|
|
|
func (h *baseDelayedBlocksHeap) Pop() interface{} {
|
|
oldHeap := *h
|
|
oldLength := len(oldHeap)
|
|
popped := oldHeap[oldLength-1]
|
|
*h = oldHeap[0 : oldLength-1]
|
|
return popped
|
|
}
|
|
|
|
func (h baseDelayedBlocksHeap) peek() interface{} {
|
|
if h.Len() > 0 {
|
|
return h[h.Len()-1]
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (h baseDelayedBlocksHeap) Less(i, j int) bool {
|
|
return h[j].processTime.After(h[i].processTime)
|
|
}
|
|
|
|
type delayedBlocksHeap struct {
|
|
baseDelayedBlocksHeap *baseDelayedBlocksHeap
|
|
impl heap.Interface
|
|
}
|
|
|
|
// newDelayedBlocksHeap initializes and returns a new delayedBlocksHeap
|
|
func newDelayedBlocksHeap() delayedBlocksHeap {
|
|
baseHeap := &baseDelayedBlocksHeap{}
|
|
h := delayedBlocksHeap{impl: baseHeap, baseDelayedBlocksHeap: baseHeap}
|
|
heap.Init(h.impl)
|
|
return h
|
|
}
|
|
|
|
// pop removes the block with lowest height from this heap and returns it
|
|
func (dbh delayedBlocksHeap) pop() *delayedBlock {
|
|
return heap.Pop(dbh.impl).(*delayedBlock)
|
|
}
|
|
|
|
// Push pushes the block onto the heap
|
|
func (dbh delayedBlocksHeap) Push(block *delayedBlock) {
|
|
heap.Push(dbh.impl, block)
|
|
}
|
|
|
|
// Len returns the length of this heap
|
|
func (dbh delayedBlocksHeap) Len() int {
|
|
return dbh.impl.Len()
|
|
}
|
|
|
|
// peek returns the topmost element in the queue without poping it
|
|
func (dbh delayedBlocksHeap) peek() *delayedBlock {
|
|
if dbh.baseDelayedBlocksHeap.peek() == nil {
|
|
return nil
|
|
}
|
|
return dbh.baseDelayedBlocksHeap.peek().(*delayedBlock)
|
|
}
|