mirror of
https://github.com/openpgpjs/openpgpjs.git
synced 2025-06-30 18:12:30 +00:00
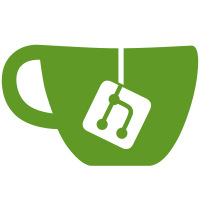
Mocha v10 requires the lib to be esm compliant. ESM mandates the use of file extensions in imports, so to minimize the changes (for now), we rely on the flag `experimental-specifier-resolution=node` and on `ts-node` (needed only for Node 20). Breaking changes: downstream bundlers might be affected by the package.json changes depending on how they load the library. NB: legacy package.json entrypoints are still available.
60 lines
1.7 KiB
JavaScript
60 lines
1.7 KiB
JavaScript
import { use as chaiUse, expect } from 'chai';
|
|
import chaiAsPromised from 'chai-as-promised';
|
|
chaiUse(chaiAsPromised);
|
|
|
|
const openpgp = typeof window !== 'undefined' && window.openpgp ? window.openpgp : await import('openpgp');
|
|
|
|
const password = 'I am a password';
|
|
|
|
const tests = {
|
|
zip: {
|
|
input: `-----BEGIN PGP MESSAGE-----
|
|
|
|
jA0ECQMC5rhAA7l3jOzk0kwBTMc07y+1NME5RCUQ2EOlSofbh1KARLC5B1NMeBlq
|
|
jS917VBeCW3R21xG+0ZJ6Z5iWwdQD7XBtg19doWOqExSmXBWWW/6vSaD81ox
|
|
=Gw9+
|
|
-----END PGP MESSAGE-----`,
|
|
output: 'Hello world! With zip.'
|
|
},
|
|
zlib: {
|
|
input: `-----BEGIN PGP MESSAGE-----
|
|
|
|
jA0ECQMC8Qfig2+Tygnk0lMB++5JoyZUcpUy5EJqcxBuy93tXw+BSk7OhFhda1Uo
|
|
JuQlKv27HlyUaA55tMJsFYPypGBLEXW3k0xi3Cs87RrLqmVGTZSqNhHOVNE28lVe
|
|
W40mpQ==
|
|
=z0we
|
|
-----END PGP MESSAGE-----`,
|
|
output: 'Hello world! With zlib.'
|
|
},
|
|
bzip2: {
|
|
input: `-----BEGIN PGP MESSAGE-----
|
|
|
|
jA0ECQMC97w+wp7u9/Xk0oABBfapJBuuxGBiHDfNmVgsRzbjLDBWTJ3LD4UtxEku
|
|
qu6hwp5JXB0TgI/XQ3tKobSqHv1wSJ9SVxtWZq6WvWulu+j9GtzIVC3mbDA/qRA3
|
|
41sUEMdAFC6I7BYLYGEiUAVNpjbvGOmJWptDyawjRgEuZeTzKyTI/UcMc/rLy9Pz
|
|
Xg==
|
|
=6ek1
|
|
-----END PGP MESSAGE-----`,
|
|
output: 'Hello world! With bzip2.'
|
|
}
|
|
};
|
|
|
|
export default () => describe('Decrypt and decompress message tests', function () {
|
|
|
|
function runTest(key, test) {
|
|
it(`Decrypts message compressed with ${key}`, async function () {
|
|
const message = await openpgp.readMessage({ armoredMessage: test.input });
|
|
const options = {
|
|
passwords: password,
|
|
message
|
|
};
|
|
return openpgp.decrypt(options).then(function (decrypted) {
|
|
expect(decrypted.data).to.equal(test.output + '\n');
|
|
});
|
|
});
|
|
}
|
|
|
|
Object.keys(tests).forEach(key => runTest(key, tests[key]));
|
|
|
|
});
|