mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-03-30 15:08:28 +00:00
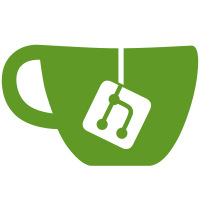
* Initializing rootDir of dao and machine keeper with the home path of the validator key material * added Block height logging of context decorator * removed SetRoot usage * fixed data races of the attest machine go-routine * reproduction of the issue * fixed testing URL issue * refactored the machine-nft functions/mock * fixed keeper.param read-bug that increased the gas prices in an inconsistent way * increased the validator number to 3 for all e2e tests * added go routine to attest machine workflow --------- Signed-off-by: Julian Strobl <jmastr@mailbox.org> Signed-off-by: Jürgen Eckel <juergen@riddleandcode.com> Signed-off-by: Lorenz Herzberger <lorenzherzberger@gmail.com> Co-authored-by: Julian Strobl <jmastr@mailbox.org> Co-authored-by: Lorenz Herzberger <lorenzherzberger@gmail.com>
74 lines
2.5 KiB
Go
74 lines
2.5 KiB
Go
package keeper
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/planetmint/planetmint-go/x/machine/keeper"
|
|
"github.com/planetmint/planetmint-go/x/machine/types"
|
|
|
|
tmdb "github.com/cometbft/cometbft-db"
|
|
"github.com/cometbft/cometbft/libs/log"
|
|
tmproto "github.com/cometbft/cometbft/proto/tendermint/types"
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
codectypes "github.com/cosmos/cosmos-sdk/codec/types"
|
|
"github.com/cosmos/cosmos-sdk/store"
|
|
storetypes "github.com/cosmos/cosmos-sdk/store/types"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
authtypes "github.com/cosmos/cosmos-sdk/x/auth/types"
|
|
govtypes "github.com/cosmos/cosmos-sdk/x/gov/types"
|
|
typesparams "github.com/cosmos/cosmos-sdk/x/params/types"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func MachineKeeper(t testing.TB) (*keeper.Keeper, sdk.Context) {
|
|
storeKey := sdk.NewKVStoreKey(types.StoreKey)
|
|
taIndexStoreKey := sdk.NewKVStoreKey(types.TAIndexKey)
|
|
issuerPlanetmintIndexStoreKey := sdk.NewKVStoreKey(types.IssuerPlanetmintIndexKey)
|
|
issuerLiquidIndexStoreKey := sdk.NewKVStoreKey(types.IssuerLiquidIndexKey)
|
|
trustAnchorStoreKey := sdk.NewKVStoreKey(types.TrustAnchorKey)
|
|
addressStoreKey := sdk.NewKVStoreKey(types.AddressIndexKey)
|
|
memStoreKey := storetypes.NewMemoryStoreKey(types.MemStoreKey)
|
|
|
|
db := tmdb.NewMemDB()
|
|
stateStore := store.NewCommitMultiStore(db)
|
|
stateStore.MountStoreWithDB(storeKey, storetypes.StoreTypeIAVL, db)
|
|
stateStore.MountStoreWithDB(taIndexStoreKey, storetypes.StoreTypeIAVL, db)
|
|
stateStore.MountStoreWithDB(issuerPlanetmintIndexStoreKey, storetypes.StoreTypeIAVL, db)
|
|
stateStore.MountStoreWithDB(issuerLiquidIndexStoreKey, storetypes.StoreTypeIAVL, db)
|
|
stateStore.MountStoreWithDB(trustAnchorStoreKey, storetypes.StoreTypeIAVL, db)
|
|
stateStore.MountStoreWithDB(addressStoreKey, storetypes.StoreTypeIAVL, db)
|
|
stateStore.MountStoreWithDB(memStoreKey, storetypes.StoreTypeMemory, nil)
|
|
require.NoError(t, stateStore.LoadLatestVersion())
|
|
|
|
registry := codectypes.NewInterfaceRegistry()
|
|
cdc := codec.NewProtoCodec(registry)
|
|
|
|
paramsSubspace := typesparams.NewSubspace(cdc,
|
|
types.Amino,
|
|
storeKey,
|
|
memStoreKey,
|
|
"MachineParams",
|
|
)
|
|
|
|
k := keeper.NewKeeper(
|
|
cdc,
|
|
storeKey,
|
|
taIndexStoreKey,
|
|
issuerPlanetmintIndexStoreKey,
|
|
issuerLiquidIndexStoreKey,
|
|
trustAnchorStoreKey,
|
|
addressStoreKey,
|
|
memStoreKey,
|
|
paramsSubspace,
|
|
authtypes.NewModuleAddress(govtypes.ModuleName).String(),
|
|
"",
|
|
)
|
|
|
|
ctx := sdk.NewContext(stateStore, tmproto.Header{}, false, log.NewNopLogger())
|
|
|
|
// Initialize params
|
|
_ = k.SetParams(ctx, types.DefaultParams())
|
|
|
|
return k, ctx
|
|
}
|