mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-03-30 15:08:28 +00:00
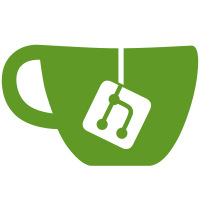
* distributed & result msgs * added DistributionResult * added RDDL token conversion methods * set proper validatoraddress within the testcases for e2e/dao * set proper root dir for test cases * fixed some wordings --------- Signed-off-by: Jürgen Eckel <juergen@riddleandcode.com>
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
package cli
|
|
|
|
import (
|
|
"strconv"
|
|
|
|
"encoding/json"
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
"github.com/cosmos/cosmos-sdk/client/flags"
|
|
"github.com/cosmos/cosmos-sdk/client/tx"
|
|
"github.com/planetmint/planetmint-go/x/dao/types"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
var _ = strconv.Itoa(0)
|
|
|
|
func CmdDistributionRequest() *cobra.Command {
|
|
cmd := &cobra.Command{
|
|
Use: "distribution-request [distribution]",
|
|
Short: "Broadcast message distribution-request",
|
|
Args: cobra.ExactArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) (err error) {
|
|
argDistribution := new(types.DistributionOrder)
|
|
err = json.Unmarshal([]byte(args[0]), argDistribution)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
clientCtx, err := client.GetClientTxContext(cmd)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
msg := types.NewMsgDistributionRequest(
|
|
clientCtx.GetFromAddress().String(),
|
|
argDistribution,
|
|
)
|
|
if err := msg.ValidateBasic(); err != nil {
|
|
return err
|
|
}
|
|
return tx.GenerateOrBroadcastTxCLI(clientCtx, cmd.Flags(), msg)
|
|
},
|
|
}
|
|
|
|
flags.AddTxFlagsToCmd(cmd)
|
|
|
|
return cmd
|
|
}
|