mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-03-30 15:08:28 +00:00
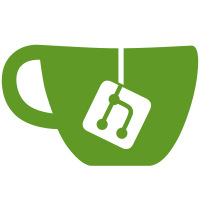
* refactor: replace claim client with exported version * refactor: replace shamir coordinator client with exported version * chore: update rddl-claim-service/client dependency * chore: update shamir-coordinator-service client Signed-off-by: Lorenz Herzberger <lorenzherzberger@gmail.com> Signed-off-by: Julian Strobl <jmastr@mailbox.org>
67 lines
2.1 KiB
Go
67 lines
2.1 KiB
Go
// Code generated by MockGen. DO NOT EDIT.
|
|
// Source: ./clients/claim_client.go
|
|
|
|
// Package mocks is a generated GoMock package.
|
|
package mocks
|
|
|
|
import (
|
|
context "context"
|
|
reflect "reflect"
|
|
|
|
gomock "github.com/golang/mock/gomock"
|
|
"github.com/rddl-network/rddl-claim-service/types"
|
|
)
|
|
|
|
// MockIRCClient is a mock of IRCClient interface.
|
|
type MockIRCClient struct {
|
|
ctrl *gomock.Controller
|
|
recorder *MockIRCClientMockRecorder
|
|
}
|
|
|
|
// MockIRCClientMockRecorder is the mock recorder for MockIRCClient.
|
|
type MockIRCClientMockRecorder struct {
|
|
mock *MockIRCClient
|
|
}
|
|
|
|
// NewMockIRCClient creates a new mock instance.
|
|
func NewMockIRCClient(ctrl *gomock.Controller) *MockIRCClient {
|
|
mock := &MockIRCClient{ctrl: ctrl}
|
|
mock.recorder = &MockIRCClientMockRecorder{mock}
|
|
return mock
|
|
}
|
|
|
|
// EXPECT returns an object that allows the caller to indicate expected use.
|
|
func (m *MockIRCClient) EXPECT() *MockIRCClientMockRecorder {
|
|
return m.recorder
|
|
}
|
|
|
|
// GetClaim mocks base method.
|
|
func (m *MockIRCClient) GetClaim(ctx context.Context, id int) (types.GetClaimResponse, error) {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetClaim", ctx, id)
|
|
ret0, _ := ret[0].(types.GetClaimResponse)
|
|
ret1, _ := ret[1].(error)
|
|
return ret0, ret1
|
|
}
|
|
|
|
// GetClaim indicates an expected call of GetClaim.
|
|
func (mr *MockIRCClientMockRecorder) GetClaim(ctx, id interface{}) *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetClaim", reflect.TypeOf((*MockIRCClient)(nil).GetClaim), ctx, id)
|
|
}
|
|
|
|
// PostClaim mocks base method.
|
|
func (m *MockIRCClient) PostClaim(ctx context.Context, req types.PostClaimRequest) (types.PostClaimResponse, error) {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "PostClaim", ctx, req)
|
|
ret0, _ := ret[0].(types.PostClaimResponse)
|
|
ret1, _ := ret[1].(error)
|
|
return ret0, ret1
|
|
}
|
|
|
|
// PostClaim indicates an expected call of PostClaim.
|
|
func (mr *MockIRCClientMockRecorder) PostClaim(ctx, req interface{}) *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "PostClaim", reflect.TypeOf((*MockIRCClient)(nil).PostClaim), ctx, req)
|
|
}
|