mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-07-08 05:32:30 +00:00
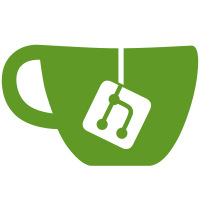
* chore: remove unused asset.proto and asset.pb.go files * feat: add AssetByAddress store functionality * fix: asset query now returns numElements passed in req * chore: add migration for new store mechanics * chore: set upgradehandler for assetmodule migration * chore: removed obsolete GetCIDsByAddress function * chore: adjust cmd usage --------- Signed-off-by: Lorenz Herzberger <lorenzherzberger@gmail.com>
43 lines
1.0 KiB
Go
43 lines
1.0 KiB
Go
package util
|
|
|
|
import (
|
|
"encoding/binary"
|
|
"encoding/hex"
|
|
)
|
|
|
|
func SerializeInt64(value int64) []byte {
|
|
// Adding 1 because 0 will be interpreted as nil, which is an invalid key
|
|
buf := make([]byte, 8)
|
|
// Use binary.BigEndian to write the int64 into the byte slice
|
|
binary.BigEndian.PutUint64(buf, uint64(value+1))
|
|
return buf
|
|
}
|
|
|
|
func DeserializeInt64(value []byte) int64 {
|
|
integer := binary.BigEndian.Uint64(value)
|
|
// Subtract 1 because addition in serialization
|
|
return int64(integer - 1)
|
|
}
|
|
|
|
func SerializeUint64(value uint64) []byte {
|
|
buf := make([]byte, 8)
|
|
// Adding 1 because 0 will be interpreted as nil, which is an invalid key
|
|
binary.BigEndian.PutUint64(buf, value+1)
|
|
return buf
|
|
}
|
|
|
|
func DeserializeUint64(value []byte) uint64 {
|
|
integer := binary.BigEndian.Uint64(value)
|
|
// Subtract 1 because addition in serialization
|
|
return integer - 1
|
|
}
|
|
|
|
func SerializeString(value string) []byte {
|
|
byteArray := []byte(value)
|
|
return byteArray
|
|
}
|
|
|
|
func SerializeHexString(value string) ([]byte, error) {
|
|
return hex.DecodeString(value)
|
|
}
|