mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-06-30 18:02:30 +00:00
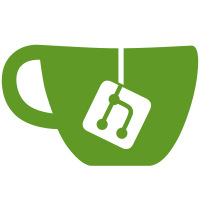
Golang uses: - Camel Case for variable names, e.g. `firstName` - Camel Case for private function names, e.g. `getFirstName` - Pascal Case for public function names, e.g. `GetFirstName` Signed-off-by: Julian Strobl <jmastr@mailbox.org>
60 lines
1.6 KiB
Go
60 lines
1.6 KiB
Go
package util
|
|
|
|
import (
|
|
"bytes"
|
|
"errors"
|
|
"os/exec"
|
|
"strconv"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/planetmint/planetmint-go/config"
|
|
)
|
|
|
|
func InitRDDLReissuanceProcess(ctx sdk.Context, proposerAddress string, txUnsigned string, blockHeight int64) error {
|
|
//get_last_PoPBlockHeight() // TODO: to be read form the upcoming PoP-store
|
|
logger := ctx.Logger()
|
|
// Construct the command
|
|
sendingValidatorAddress := config.GetConfig().ValidatorAddress
|
|
logger.Debug("REISSUE: create Proposal")
|
|
cmd := exec.Command("planetmint-god", "tx", "dao", "reissue-rddl-proposal",
|
|
"--from", sendingValidatorAddress, "-y",
|
|
proposerAddress, txUnsigned, strconv.FormatInt(blockHeight, 10))
|
|
|
|
var stdout, stderr bytes.Buffer
|
|
cmd.Stdout = &stdout
|
|
cmd.Stderr = &stderr
|
|
|
|
// Start the command in a non-blocking way
|
|
err := cmd.Start()
|
|
errstr := stderr.String()
|
|
if err != nil || len(errstr) > 0 {
|
|
if err == nil {
|
|
err = errors.New(errstr)
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
|
|
func SendRDDLReissuanceResult(ctx sdk.Context, proposerAddress string, txID string, blockHeight int64) error {
|
|
logger := ctx.Logger()
|
|
// Construct the command
|
|
sendingValidatorAddress := config.GetConfig().ValidatorAddress
|
|
logger.Debug("REISSUE: create Result")
|
|
cmd := exec.Command("planetmint-god", "tx", "dao", "reissue-rddl-result",
|
|
"--from", sendingValidatorAddress, "-y",
|
|
proposerAddress, txID, strconv.FormatInt(blockHeight, 10))
|
|
|
|
var stdout, stderr bytes.Buffer
|
|
cmd.Stdout = &stdout
|
|
cmd.Stderr = &stderr
|
|
// Start the command in a non-blocking way
|
|
err := cmd.Start()
|
|
errstr := stderr.String()
|
|
if err != nil || len(errstr) > 0 {
|
|
if err == nil {
|
|
err = errors.New(errstr)
|
|
}
|
|
}
|
|
return err
|
|
}
|