mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-03-30 15:08:28 +00:00
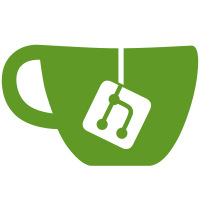
* added upper and lower case TA resolution testing * added more detailed error reporting to the ValidateSignature method. * extended test cases to test and verify these errs and their differences * fixed CID attestation issue. CIDs are send in web compatible encoding that is not hex encoded and can be utilized without any further decoding on the server side. * added checks and error handling for the Ta store object storage/loading Signed-off-by: Jürgen Eckel <juergen@riddleandcode.com>
87 lines
2.0 KiB
Go
87 lines
2.0 KiB
Go
package keeper_test
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"fmt"
|
|
"strings"
|
|
"testing"
|
|
|
|
keepertest "planetmint-go/testutil/keeper"
|
|
|
|
"planetmint-go/x/machine/keeper"
|
|
"planetmint-go/x/machine/types"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func createNTrustAnchor(t *testing.T, keeper *keeper.Keeper, ctx sdk.Context, n int) []types.TrustAnchor {
|
|
items := make([]types.TrustAnchor, n)
|
|
for i := range items {
|
|
pk := fmt.Sprintf("pubkey%v", i)
|
|
if i%2 == 1 {
|
|
pk = strings.ToUpper(pk)
|
|
}
|
|
|
|
items[i].Pubkey = hex.EncodeToString([]byte(pk))
|
|
var activated bool
|
|
if i%2 == 1 {
|
|
activated = true
|
|
} else {
|
|
activated = false
|
|
}
|
|
err := keeper.StoreTrustAnchor(ctx, items[i], activated)
|
|
assert.False(t, (err != nil))
|
|
}
|
|
return items
|
|
}
|
|
|
|
func TestGetTrustAnchor(t *testing.T) {
|
|
keeper, ctx := keepertest.MachineKeeper(t)
|
|
items := createNTrustAnchor(t, keeper, ctx, 10)
|
|
for i, item := range items {
|
|
ta, activated, found := keeper.GetTrustAnchor(ctx, item.Pubkey)
|
|
assert.True(t, found)
|
|
assert.Equal(t, item, ta)
|
|
if i%2 == 1 {
|
|
assert.True(t, activated)
|
|
} else {
|
|
assert.False(t, activated)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestUpdateTrustAnchor(t *testing.T) {
|
|
keeper, ctx := keepertest.MachineKeeper(t)
|
|
items := createNTrustAnchor(t, keeper, ctx, 10)
|
|
for _, item := range items {
|
|
ta, activated, _ := keeper.GetTrustAnchor(ctx, item.Pubkey)
|
|
if !activated {
|
|
err := keeper.StoreTrustAnchor(ctx, ta, true)
|
|
assert.False(t, (err != nil))
|
|
}
|
|
}
|
|
|
|
for _, item := range items {
|
|
_, activated, _ := keeper.GetTrustAnchor(ctx, item.Pubkey)
|
|
assert.True(t, activated)
|
|
}
|
|
}
|
|
|
|
func TestUpdateTrustAnchorInvalidPubKey(t *testing.T) {
|
|
keeper, ctx := keepertest.MachineKeeper(t)
|
|
items := createNTrustAnchor(t, keeper, ctx, 10)
|
|
for _, item := range items {
|
|
ta, activated, _ := keeper.GetTrustAnchor(ctx, item.Pubkey)
|
|
if !activated {
|
|
err := keeper.StoreTrustAnchor(ctx, ta, true)
|
|
assert.False(t, (err != nil))
|
|
}
|
|
}
|
|
|
|
for _, item := range items {
|
|
_, activated, _ := keeper.GetTrustAnchor(ctx, item.Pubkey)
|
|
assert.True(t, activated)
|
|
}
|
|
}
|